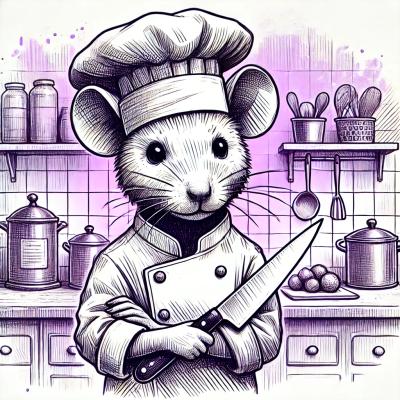
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
ra-i18n-polyglot
Advanced tools
Polyglot i18n provider for react-admin, the frontend framework for building admin applications on top of REST/GraphQL services. It relies on polyglot.js, which uses JSON files for translations.
npm install --save ra-i18n-polyglot
Wrap the function exported by this package around a function returning translation messages based on a locale to produce a valid i18nProvider
.
import * as React from "react";
import { Admin, Resource } from 'react-admin';
import polyglotI18nProvider from 'ra-i18n-polyglot';
import englishMessages from 'ra-language-english';
import frenchMessages from 'ra-language-french';
const messages = {
fr: frenchMessages,
en: englishMessages,
};
const i18nProvider = polyglotI18nProvider(locale => messages[locale]);
const App = () => (
<Admin locale="en" i18nProvider={i18nProvider}>
...
</Admin>
);
export default App;
The message
returned by the function argument should be a dictionary where the keys identify interface components, and values are the translated string. This dictionary is a simple JavaScript object looking like the following:
{
ra: {
action: {
delete: 'Delete',
show: 'Show',
list: 'List',
save: 'Save',
create: 'Create',
edit: 'Edit',
cancel: 'Cancel',
},
...
},
}
All core translations are in the ra
namespace, in order to prevent collisions with your own custom translations. The root key used at runtime is determined by the value of the locale
prop.
The default messages are available here.
The function passed as parameter of polyglotI18nProvider
can return a Promise for messages instead of a messages object. This lets you lazy load messages upon language change.
Note that the messages for the default locale (used by react-admin for the initial render) must be returned in a synchronous way.
import polyglotI18nProvider from 'ra-i18n-polyglot';
import englishMessages from 'ra-language-english';
const asyncMessages = {
fr: () => import('ra-language-french').then(messages => messages.default),
it: () => import('ra-language-italian').then(messages => messages.default),
};
const messagesResolver = locale => {
if (locale === 'en') {
// initial call, must return synchronously
return englishMessages;
}
// change of locale after initial call returns a promise
return asyncMessages[params.locale]();
}
const i18nProvider = polyglotI18nProvider(messagesResolver);
Polyglot.js is a fantastic library: in addition to being small, fully maintained, and totally framework agnostic, it provides some nice features such as interpolation and pluralization, that you can use in react-admin.
const messages = {
'hello_name': 'Hello, %{name}',
'count_beer': 'One beer |||| %{smart_count} beers',
};
// interpolation
translate('hello_name', { name: 'John Doe' });
=> 'Hello, John Doe.'
// pluralization
translate('count_beer', { smart_count: 1 });
=> 'One beer'
translate('count_beer', { smart_count: 2 });
=> '2 beers'
// default value
translate('not_yet_translated', { _: 'Default translation' });
=> 'Default translation'
To find more detailed examples, please refer to http://airbnb.io/polyglot.js/
v4.0.0
React-admin v4 focuses on modernizing the inner workings of the library. It improves the developper experience a great deal, and paves the way for future changes. It is the result of 6 months of intensive refactoring, development, and test.
The following list concerns version 4.0.0, as well as all the pre-releases (alpha, beta, and rc).
<Admin requireAuth>
to hide the app until auth is checked (#7475)<Admin basename>
to allow mounting react-admin inside a sub path (#7100, #6917)dataProvider
hooks (#7116)total
) (#7120)sx
props in all ra-ui-materialui
components (#7175)<Form>
component (#7087)<ReferenceOneField>
(#7060)<CustomRoutes>
(#7345)useStore
and persistent preferences (backport from ra-enterprise
) (#7158, #7366)<ToggleThemeButton>
(#7340)<LocalesMenuButton>
(#7332)useSetTheme
(#7008)combineDataProvider
helper (#7055)<Datagrid expandSingle>
to limit the number of expanded rows to 1 (#7454)<ChoicesContextProvider>
in all ReferenceInputs to avoid child cloning and allow choices filtering, pagination, and sorting (#7185)<FileInput validateFileRemoval>
prop to allow confirmation before file deletion (#7003)<Resource options>
(#7392)material-ui
to v5 (#6650)react-query
for data fetching instead of home made solution (#6779, #6916, #7006, #7016, #7025, #6891, #7035, #7020, #7035, #7001)react-final-form
with react-hook-form
(#7087)react-router
to V6 (#6873)Quill
by TipTap
in <RichTextInput>
(#7153)Record
TypeScript name to RaRecord
(#7078)record
prop injectionvariant
and margin
, and document theme override instead (#7223)loading
to isLoading
in authProvider
hooks return type (#7334)initialValues
to defaultValues
in <Form>
(caused by switch to react-hook-form
)bulkActionButtons
from <List>
to <Datagrid>
(#7114)currentSort
to sort
(#7076)setSort
signature to make it consistent across components (#7065)<TranslationProvider>
to <I18nContextProvider>
<WithPermissions>
wrapping to a useAuthenticated
hook in main controllers (#6921)<Notification>
component into <AdminUI>
to avoid gotchas when overriding the layout (#7082)Redux
(#7177)redux-saga
and saga-based side effects (#6684)connected-react-router
(#6704)basePath
(#7100)addLabel
prop in Field components (#7223)Resource
initialization, Store Resource definitions in Context rather than in store (#7051)addField
) and render propsuseQuery
and useMutation
(as react-query
already provides them) (#7001)validUntil
(#7001)useVersion
(#7001)allowEmpty
prop in choice inputs (#7200)sort
prop in <DataGridHeaderCell>
(#7065)<FormWithRedirect>
and handleSubmitWithRedirect
(#7087)TestContext
(<AdminContext>
does the trick) and ra-test
(#7148)useGetMatching
(use getList instead) (#6916)undoable
prop now that we have mutationMode
(#6711)withTranslate
HOC (#7157)ra-test
(#7148)esbuild
instead of webpack
for simple exampleAs this is a major release, there are breaking changes. We documented all the changes required in a react-admin v3 application to make it compatible with version 4 in the react-admin v4 Upgrade Guide.
Since react-admin counts about 112,000 lines of code, this means that 90% of the codebase was touched.
Many thanks to all the contributors (whether they helped developing, testing, documenting, proofreading react-admin v4), and in particular to the core team (fzaninotto, djhi, WiXSL) for their hard work.
FAQs
Polyglot i18n provider for react-admin
The npm package ra-i18n-polyglot receives a total of 57,228 weekly downloads. As such, ra-i18n-polyglot popularity was classified as popular.
We found that ra-i18n-polyglot demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.