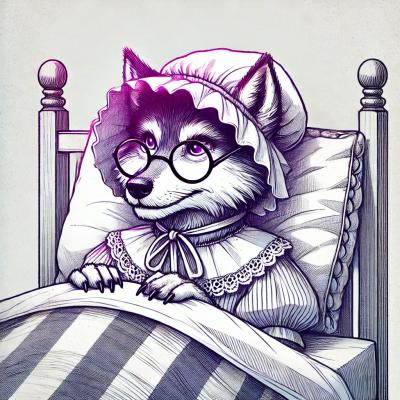
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The rc-form package is a form management library for React. It provides a set of utilities to manage form state, validation, and submission, making it easier to handle complex forms in React applications.
Form Creation
This feature allows you to create forms with validation rules. The `createForm` function is used to wrap the form component, and `getFieldDecorator` is used to register form fields with validation rules.
```jsx
import React from 'react';
import { createForm } from 'rc-form';
class MyForm extends React.Component {
handleSubmit = () => {
this.props.form.validateFields((err, values) => {
if (!err) {
console.log('Received values of form: ', values);
}
});
};
render() {
const { getFieldDecorator } = this.props.form;
return (
<form onSubmit={this.handleSubmit}>
<div>
{getFieldDecorator('username', {
rules: [{ required: true, message: 'Please input your username!' }],
})(<input type="text" placeholder="Username" />)}
</div>
<button type="submit">Submit</button>
</form>
);
}
}
export default createForm()(MyForm);
```
Field Validation
This feature allows you to add validation rules to form fields. In this example, the email field is validated to ensure it is a valid email address and is required.
```jsx
import React from 'react';
import { createForm } from 'rc-form';
class MyForm extends React.Component {
handleSubmit = () => {
this.props.form.validateFields((err, values) => {
if (!err) {
console.log('Received values of form: ', values);
}
});
};
render() {
const { getFieldDecorator } = this.props.form;
return (
<form onSubmit={this.handleSubmit}>
<div>
{getFieldDecorator('email', {
rules: [
{ type: 'email', message: 'The input is not valid E-mail!' },
{ required: true, message: 'Please input your E-mail!' }
],
})(<input type="email" placeholder="Email" />)}
</div>
<button type="submit">Submit</button>
</form>
);
}
}
export default createForm()(MyForm);
```
Dynamic Form Fields
This feature allows you to dynamically add form fields. The `addField` method updates the state to add new fields, and `getFieldDecorator` is used to register these fields with validation rules.
```jsx
import React from 'react';
import { createForm } from 'rc-form';
class DynamicForm extends React.Component {
state = { keys: [] };
addField = () => {
const { keys } = this.state;
this.setState({ keys: keys.concat(keys.length) });
};
handleSubmit = () => {
this.props.form.validateFields((err, values) => {
if (!err) {
console.log('Received values of form: ', values);
}
});
};
render() {
const { getFieldDecorator } = this.props.form;
const { keys } = this.state;
return (
<form onSubmit={this.handleSubmit}>
{keys.map(k => (
<div key={k}>
{getFieldDecorator(`field-${k}`, {
rules: [{ required: true, message: 'Please input a value!' }],
})(<input type="text" placeholder="Dynamic Field" />)}
</div>
))}
<button type="button" onClick={this.addField}>Add Field</button>
<button type="submit">Submit</button>
</form>
);
}
}
export default createForm()(DynamicForm);
```
Formik is a popular form management library for React. It provides a set of tools to handle form state, validation, and submission. Compared to rc-form, Formik offers a more modern API and better integration with React hooks.
React Hook Form is a performant, flexible, and extensible form library for React. It leverages React hooks to manage form state and validation. It is known for its minimal re-renders and easy integration with existing components, making it a strong alternative to rc-form.
Redux Form is a library that integrates form state management with Redux. It allows you to manage form state in a centralized Redux store. While it offers powerful features, it can be more complex to set up compared to rc-form.
React High Order Form Component.
Note: This is unstable, under development now.
npm install
npm start
http://localhost:8000/examples/
online example: http://react-component.github.io/form/examples/
import { createForm } from 'rc-form';
@createForm()
class Form extends React.Component {
render() {
let errors;
const {getFieldProps, getFieldError, isFieldValidating} = props.form;
return (<div>
<input {...getFieldProps('normal')}/>
<input {...getFieldProps('required',{
rules:[{required:true}]
})}/>
{(errors = getFieldError('required')) ? errors.join(',') : null}
</div>)
}
}
Called when field changed, you can dispatch fields to redux store.
convert value from props to fields. used for read fields from redux store.
createForm() will return another function:
Will pass a object as prop form with the following members to WrappedComponent:
Will create props which can be set on a input/InputComponent which support value and onChange interface.
After set, this will create a binding with this input.
type: String. this input's unique name
type: String. event which is listened to validate. Default to 'onChange', set to false to only validate when call props.validateFields.
type: String. event which is listened to collect form data. Default to 'onChange', set to false to stop collect form data.
type: Object[]. validator rules. see: https://github.com/yiminghe/async-validator
Get fields value by fieldNames.
set fields value by kv object.
Validate and get fields value by fieldNames.
Get input's validate errors
Whether this input is validating
http://localhost:8000/tests/runner.html?coverage
rc-form is released under the MIT license.
FAQs
React High Order Form Component
We found that rc-form demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.