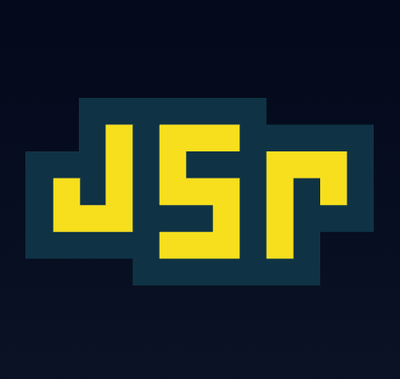
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
rc-select
Advanced tools
The rc-select npm package is a React component that provides a customizable select box or dropdown list. It supports various functionalities such as searching, multiple selection, custom rendering, and more, making it a versatile choice for implementing select inputs in web applications.
Basic Select
This code sample demonstrates how to create a basic select dropdown with predefined options. Users can select one of the options from the dropdown.
import Select from 'rc-select';
<Select placeholder="Please select">
<Select.Option value="option1">Option 1</Select.Option>
<Select.Option value="option2">Option 2</Select.Option>
</Select>
Multiple Selection
This example shows how to enable multiple selections, allowing users to select more than one option from the dropdown.
import Select from 'rc-select';
<Select mode="multiple" placeholder="Please select">
<Select.Option value="option1">Option 1</Select.Option>
<Select.Option value="option2">Option 2</Select.Option>
</Select>
Searchable Select
This code snippet enables a search functionality within the select dropdown, making it easier for users to find and select options by typing.
import Select from 'rc-select';
<Select showSearch placeholder="Search to select">
<Select.Option value="option1">Option 1</Select.Option>
<Select.Option value="option2">Option 2</Select.Option>
</Select>
Custom Dropdown Render
This example demonstrates how to customize the rendering of the dropdown menu, allowing for additional elements like a custom footer to be added.
import Select from 'rc-select';
<Select dropdownRender={menu => (
<div>
{menu}
<div style={{ padding: '8px', cursor: 'pointer' }}>Custom footer</div>
</div>
)}>
<Select.Option value="option1">Option 1</Select.Option>
<Select.Option value="option2">Option 2</Select.Option>
</Select>
react-select is another popular React component for building select inputs. It offers similar functionalities to rc-select, such as searchable options, multi-select, and custom option rendering. However, react-select might be preferred for its extensive documentation and larger community support.
antd, or Ant Design, is a comprehensive UI toolkit for React that includes a Select component with functionalities similar to rc-select. While rc-select is focused solely on the select component, antd offers a broader range of UI components, making it a good choice if you're looking for a complete design system.
Material-UI is another UI framework for React that includes a Select component. It adheres to the Material Design guidelines and offers a different look and feel compared to rc-select. Material-UI's Select component provides similar functionalities but is ideal for those looking to implement Material Design in their projects.
React Select Component.
import Select, { Option } from 'rc-select';
import 'rc-select/assets/index.css';
export default () => (
<Select>
<Option value="jack">jack</Option>
<Option value="lucy">lucy</Option>
<Option value="yiminghe">yiminghe</Option>
</Select>
);
name | description | type | default |
---|---|---|---|
id | html id to set on the component wrapper | String | '' |
className | additional css class of root dom node | String | '' |
data-* | html data attributes to set on the component wrapper | String | '' |
prefixCls | prefix class | String | '' |
animation | dropdown animation name. only support slide-up now | String | '' |
transitionName | dropdown css animation name | String | '' |
choiceTransitionName | css animation name for selected items at multiple mode | String | '' |
dropdownMatchSelectWidth | whether dropdown's width is same with select | boolean | true |
dropdownClassName | additional className applied to dropdown | String | - |
dropdownStyle | additional style applied to dropdown | React.CSSProperties | {} |
dropdownAlign | additional align applied to dropdown | AlignType | {} |
dropdownMenuStyle | additional style applied to dropdown menu | Object | React.CSSProperties |
notFoundContent | specify content to show when no result matches. | ReactNode | 'Not Found' |
tokenSeparators | separator used to tokenize on tag/multiple mode | string[]? | |
open | control select open | boolean | |
defaultOpen | control select default open | boolean | |
placeholder | select placeholder | React Node | |
showSearch | whether show search input in single mode | boolean | true |
allowClear | whether allowClear | boolean | { clearIcon?: ReactNode } |
tags | when tagging is enabled the user can select from pre-existing options or create a new tag by picking the first choice, which is what the user has typed into the search box so far. | boolean | false |
tagRender | render custom tags. | (props: CustomTagProps) => ReactNode | - |
maxTagTextLength | max tag text length to show | number | - |
maxTagCount | max tag count to show | number | - |
maxTagPlaceholder | placeholder for omitted values | ReactNode/function(omittedValues) | - |
combobox | enable combobox mode(can not set multiple at the same time) | boolean | false |
multiple | whether multiple select | boolean | false |
disabled | whether disabled select | boolean | false |
filterOption | whether filter options by input value. default filter by option's optionFilterProp prop's value | boolean | true/Function(inputValue:string, option:Option) |
optionFilterProp | which prop value of option will be used for filter if filterOption is true | String | 'value' |
filterSort | Sort function for search options sorting, see Array.sort's compareFunction. | Function(optionA:Option, optionB: Option) | - |
optionLabelProp | render option value or option children as content of select | String: 'value'/'children' | 'value' |
defaultValue | initial selected option(s) | String | String[] | - |
value | current selected option(s) | String | String[] | {key:String, label:React.Node} | {key:String, label:React.Node}[] | - |
labelInValue | whether to embed label in value, see above value type. Not support combobox mode | boolean | false |
backfill | whether backfill select option to search input (Only works in single and combobox mode) | boolean | false |
onChange | called when select an option or input value change(combobox) | function(value, option:Option | Option[]) | - |
onSearch | called when input changed | function | - |
onBlur | called when blur | function | - |
onFocus | called when focus | function | - |
onPopupScroll | called when menu is scrolled | function | - |
onSelect | called when a option is selected. param is option's value and option instance | Function(value, option:Option) | - |
onDeselect | called when a option is deselected. param is option's value. only called for multiple or tags | Function(value, option:Option) | - |
onInputKeyDown | called when key down on input | Function(event) | - |
defaultActiveFirstOption | whether active first option by default | boolean | true |
getPopupContainer | container which popup select menu rendered into | function(trigger:Node):Node | function(){return document.body;} |
getInputElement | customize input element | function(): Element | - |
showAction | actions trigger the dropdown to show | String[]? | - |
autoFocus | focus select after mount | boolean | - |
autoClearSearchValue | auto clear search input value when multiple select is selected/deselected | boolean | true |
prefix | specify the select prefix icon or text | ReactNode | - |
suffixIcon | specify the select arrow icon | ReactNode | - |
clearIcon | specify the clear icon | ReactNode | - |
removeIcon | specify the remove icon | ReactNode | - |
menuItemSelectedIcon | specify the item selected icon | ReactNode | (props: MenuItemProps) => ReactNode | - |
dropdownRender | render custom dropdown menu | (menu: React.Node) => ReactNode | - |
loading | show loading icon in arrow | boolean | false |
virtual | Disable virtual scroll | boolean | true |
direction | direction of dropdown | 'ltr' | 'rtl' | 'ltr' |
optionRender | Custom rendering options | (oriOption: FlattenOptionData<BaseOptionType> , info: { index: number }) => React.ReactNode | - |
labelRender | Custom rendering label | (props: LabelInValueType) => React.ReactNode | - |
maxCount | The max number of items can be selected | number | - |
name | description | parameters | return |
---|---|---|---|
focus | focus select programmably | - | - |
blur | blur select programmably | - | - |
name | description | type | default |
---|---|---|---|
className | additional class to option | String | '' |
disabled | no effect for click or keydown for this item | boolean | false |
key | if react want you to set key, then key is same as value, you can omit value | String/number | - |
value | default filter by this attribute. if react want you to set key, then key is same as value, you can omit value | String/number | - |
title | if you are not satisfied with auto-generated title which is show while hovering on selected value, you can customize it with this property | String | - |
name | description | type | default |
---|---|---|---|
label | group label | String/React.Element | - |
key | - | String | - |
value | default filter by this attribute. if react want you to set key, then key is same as value, you can omit value | String | - |
className | same as Option props | String | '' |
title | same as Option props | String | - |
npm install
npm start
local example: http://localhost:9001/
online example: http://select.react-component.now.sh/
npm test
npm run coverage
rc-select is released under the MIT license.
FAQs
React Select
The npm package rc-select receives a total of 1,106,809 weekly downloads. As such, rc-select popularity was classified as popular.
We found that rc-select demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.