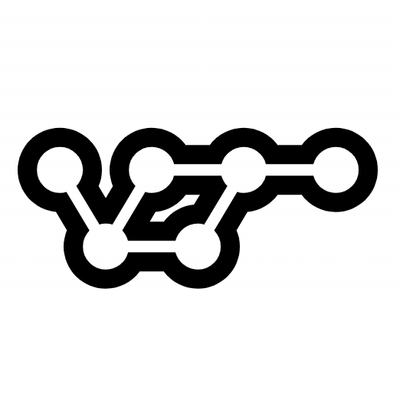
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-alert-tbc
Advanced tools
A simple react alert (toaster style) component.
You can see a live demo here.
$ npm install react-alert
To use it, you have to import the AlertContainer
component, like this:
import React, {Component} from 'react'
import AlertContainer from 'react-alert'
export default class App extends Component {
alertOptions = {
offset: 14,
position: 'bottom left',
theme: 'dark',
time: 5000,
transition: 'scale'
}
showAlert = () => {
this.msg.show('Some text or component', {
time: 2000,
type: 'success',
icon: <img src="path/to/some/img/32x32.png" />
})
}
render () {
return (
<div>
<AlertContainer ref={a => this.msg = a} {...this.alertOptions} />
<button onClick={this.showAlert}>Show Alert</button>
</div>
)
}
}
Once you have the reference of the AlertContainer
you can call the following methods:
// show an alert
this.msg.show('Some message or component')
// show an info alert
this.msg.info('Some info message or component')
// show a success alert
this.msg.success('Some success message or component')
// show an error alert
this.msg.error('Some error message or component')
// removes all alerts from the page
this.msg.removeAll()
The AlertContainer
component accepts the following props:
{
// defaults
offset: 14, // the offset of the alert from the page border, can be any number
position: 'bottom left', // the position of the alert, can be [bottom left, bottom right, top left, top right]
theme: 'dark', // the color theme of the alert, can be [dark, light]
time: 5000, // the time in miliseconds to the alert close itself, use 0 to prevent auto close (apply to all alerts)
transition: 'scale' // the transition animation, can be [scale, fade]
}
When you call show
, info
, success
and error
method, you can include the following options as a second parameter:
{
time: 0, // the time in miliseconds to the alert close itself, use 0 to prevent auto close (apply to this alert only), default is 5000
icon: <img src="path/to/some/img/32x32.png" />, // the icon to show in the alert, if none is given the default of each type will be showed
onClose: () => {} // the function called when message is closed
}
When you call the show
method, you can additionally include the info
option:
{
type: 'info' // the alert type, can be [info, success, error], default is info
}
You can also use a React Component to show an alert message, like this:
// show an alert with a React Component as content
this.msg.show(<AComponent aProp="some message" />)
FAQs
A simple react alert component
The npm package react-alert-tbc receives a total of 0 weekly downloads. As such, react-alert-tbc popularity was classified as not popular.
We found that react-alert-tbc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.