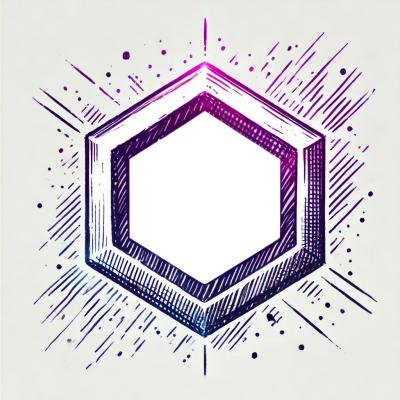
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
react-aria
Advanced tools
The react-aria package provides a collection of React hooks that help you build accessible user interfaces. It abstracts away the complexity of managing ARIA roles, properties, and keyboard interactions, making it easier to create components that are accessible to all users.
Button
The useButton hook provides the necessary ARIA attributes and event handlers to make a button accessible. It manages focus, keyboard interactions, and ARIA roles.
import { useButton } from 'react-aria';
import { useRef } from 'react';
function MyButton(props) {
let ref = useRef();
let { buttonProps } = useButton(props, ref);
return (
<button {...buttonProps} ref={ref}>
{props.children}
</button>
);
}
Checkbox
The useCheckbox hook provides the necessary ARIA attributes and event handlers to make a checkbox accessible. It manages state, keyboard interactions, and ARIA roles.
import { useCheckbox } from 'react-aria';
import { useRef } from 'react';
function MyCheckbox(props) {
let ref = useRef();
let { inputProps } = useCheckbox(props, ref);
return (
<label>
<input {...inputProps} ref={ref} />
{props.children}
</label>
);
}
Dialog
The useDialog hook provides the necessary ARIA attributes and event handlers to make a dialog accessible. It manages focus trapping, keyboard interactions, and ARIA roles.
import { useDialog } from 'react-aria';
import { useRef } from 'react';
function MyDialog(props) {
let ref = useRef();
let { dialogProps } = useDialog(props, ref);
return (
<div {...dialogProps} ref={ref}>
{props.children}
</div>
);
}
react-a11y is a package that provides accessibility warnings in the console during development. It helps developers identify and fix accessibility issues in their React components. Unlike react-aria, it does not provide hooks or components to manage ARIA attributes and interactions.
Reakit is a low-level component library for building accessible web apps. It provides primitive components that can be composed to create more complex UI elements. Reakit focuses on flexibility and composability, similar to react-aria, but offers a different API and set of components.
A set of utility components to help compose ARIA components in React. Please feel free to file an issue or submit a PR if you feel these can be more ARIA compliant β€οΈ
yarn add react-aria
npm install react-aria --save
<script src="https://unpkg.com/react-aria/dist/react-aria.js"></script>
(UMD library exposed as `ReactARIA`)
import { Trigger, Select: { Manager, OptionList, Option } } from 'react-aria'
class SelectMenu extends Component {
state = {
selection: null
}
_handleSelection = (item, e) => {
this.setState({
selection: item.value,
isOpen: false
})
}
render() {
const { isOpen } = this.state
return (
<Manager>
<Trigger
controls="select-menu"
type="menu"
isOpen={isOpen}
onToggle={() => this.setState({ isOpen: !isOpen })}
>
{this.state.selection || 'Select A Dropdown Item'}
</Trigger>
{ isOpen &&
<OptionList
id="select-menu"
onOptionSelection={this._handleSelection}
onRequestClose={() => this.setState({ isOpen: false })}
>
<Option value="apples">Apples</Option>
<Option value="pears">Pears</Option>
<Option value="oranges">Oranges</Option>
</OptionList>
}
</Manager>
)
}
}
import { Tabs: { Manager, TabList, Tab, TabPanel } } from 'react-aria'
class TabsDemo extends Component {
state = {
tabs: [{
id: 't1',
title: 'Bacon π·',
panel: <div>And eggs π</div>
}, {
id: 't2',
title: 'Zombiez π',
panel: <div>Brainz</div>
}, {
id: 't3',
title: 'Samuel π¨πΏ',
panel: <div>Samuel L Jackson</div>
}],
activeId: 't2'
}
render() {
const { tabs, activeId } = this.state
return (
<Manager
activeTabId={activeId}
onChange={id => this.setState({ activeId: id })}
className="tab-set"
>
<TabList className="tab-list">
{tabs.map(({ id, title }) =>
<Tab
key={id}
id={id}
isActive={id === activeId}
className={`tab-list-item ${id === activeId ? 'is-active' : ''}`}
>
{title}
</Tab>
)}
</TabList>
<div className="tab-panels">
{tabs.map(({ id, panel }) =>
<TabPanel
key={id}
controlledBy={id}
isActive={id === activeId}
className="tab-panel"
>
{panel}
</TabPanel>
)}
</div>
</Manager>
)
}
}
clone repo
git clone git@github.com:souporserious/react-aria.git
move into folder
cd ~/react-aria
install dependencies
npm install
run dev mode
npm run dev
open your browser and visit: http://localhost:8080/
Huge thank you to David Clark and all of his ARIA work. Most of the code in here is heavily inspired by what he has done.
FAQs
Spectrum UI components in React
The npm package react-aria receives a total of 0 weekly downloads. As such, react-aria popularity was classified as not popular.
We found that react-aria demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.