React Countdown Circle Timer

React countdown timer component in a circle shape with color and progress animation.
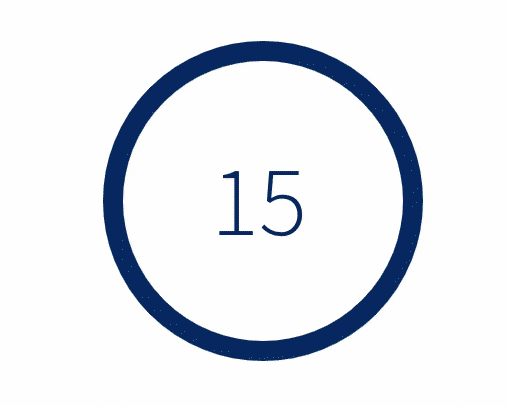
- Performance optimized with single
requestAnimationFrame
loop to animate color and progress (no setInterval
used) - Transition between colors during the countdown
- Support for linear gradient
- Fully customizable content in the center of the circle
a11y
support- Built-in and ready-to-use TypeScript type definitions.
Installation
yarn add react-countdown-circle-timer
or
npm install react-countdown-circle-timer
Migrating from v1.x.x to v2.x.x?
There are a few small API changes to consider before switching to v2.x.x. Read Migrate to v2.x.x docs for more info.
Demo
Check the demo on CodeSandbox to get started

Basic usage
import { CountdownCircleTimer } from 'react-countdown-circle-timer'
const UrgeWithPleasureComponent = () => (
<CountdownCircleTimer
isPlaying
duration={10}
colors={[['#004777', 0.33], ['#F7B801', 0.33], ['#A30000']]}
>
{({ remainingTime }) => remainingTime}
</CountdownCircleTimer>
)
Props
Refer to the list of props
Recipes
Restart timer at any given time
Pass a key
prop to CountdownCircleTimer
and change the key
when the timer should be restarted.
Repeat timer when countdown is completed
Return an array from onComplete
handler, which indicates if the animation should be repeated. Example:
const UrgeWithPleasureComponent = () => (
<CountdownCircleTimer
onComplete={() => {
// do your stuff here
return [true, 1500] // repeat animation in 1.5 seconds
}}
isPlaying
duration={10}
colors={[['#A30000']]}
/>
)
Set the initial remaining time different then the duration provided
Pass the remaining time to initialRemainingTime
prop. Example:
const UrgeWithPleasureComponent = () => (
<CountdownCircleTimer
isPlaying
duration={60}
initialRemainingTime={15}
colors={[['#A30000']]}
/>
)
In the example above, the countdown will start at 15 seconds (one quarter before it's done) and it will animate for 15 seconds until reaches 0.
Slide down time animation
Check the CodeSandbox below to find out how you can implement it yourself

Days, hours, minutes, seconds countdown
Check the demo below for one possible solution
