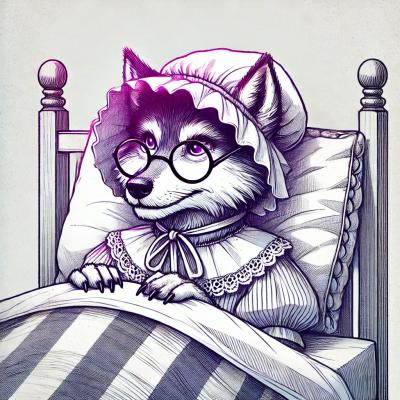
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
react-final-form
Advanced tools
react-final-form is a framework-agnostic form state management library for React. It provides a simple and flexible way to manage form state, validation, and submission handling in React applications.
Form State Management
This feature allows you to manage the state of your form fields. The code sample demonstrates a simple form with two fields, 'firstName' and 'lastName', and a submit button. The form state is managed and displayed in real-time.
const MyForm = () => (
<Form
onSubmit={formValues => {
console.log(formValues);
}}
render={({ handleSubmit, form, submitting, pristine, values }) => (
<form onSubmit={handleSubmit}>
<div>
<label>First Name</label>
<Field name="firstName" component="input" type="text" placeholder="First Name" />
</div>
<div>
<label>Last Name</label>
<Field name="lastName" component="input" type="text" placeholder="Last Name" />
</div>
<div>
<button type="submit" disabled={submitting || pristine}>
Submit
</button>
</div>
<pre>{JSON.stringify(values, 0, 2)}</pre>
</form>
)}
/>
);
Field-Level Validation
This feature allows you to add validation to individual fields. The code sample shows how to add a 'required' validation to the 'firstName' and 'lastName' fields.
const required = value => (value ? undefined : 'Required');
const MyForm = () => (
<Form
onSubmit={formValues => {
console.log(formValues);
}}
render={({ handleSubmit }) => (
<form onSubmit={handleSubmit}>
<div>
<label>First Name</label>
<Field name="firstName" component="input" type="text" placeholder="First Name" validate={required} />
</div>
<div>
<label>Last Name</label>
<Field name="lastName" component="input" type="text" placeholder="Last Name" validate={required} />
</div>
<div>
<button type="submit">
Submit
</button>
</div>
</form>
)}
/>
);
Form-Level Validation
This feature allows you to add validation to the entire form. The code sample demonstrates how to validate the 'firstName' and 'lastName' fields at the form level.
const validate = values => {
const errors = {};
if (!values.firstName) {
errors.firstName = 'Required';
}
if (!values.lastName) {
errors.lastName = 'Required';
}
return errors;
};
const MyForm = () => (
<Form
onSubmit={formValues => {
console.log(formValues);
}}
validate={validate}
render={({ handleSubmit }) => (
<form onSubmit={handleSubmit}>
<div>
<label>First Name</label>
<Field name="firstName" component="input" type="text" placeholder="First Name" />
</div>
<div>
<label>Last Name</label>
<Field name="lastName" component="input" type="text" placeholder="Last Name" />
</div>
<div>
<button type="submit">
Submit
</button>
</div>
</form>
)}
/>
);
Formik is a popular form library for React that provides a higher-level API for managing form state, validation, and submission. It is similar to react-final-form but offers more built-in hooks and components for easier integration.
React Hook Form is a performant, flexible, and extensible form library for React. It uses hooks to manage form state and validation, making it a lightweight alternative to react-final-form with a focus on performance.
Redux Form is a form state management library that integrates with Redux. It is similar to react-final-form but is more tightly coupled with Redux, making it suitable for applications that already use Redux for state management.
FAQs
🏁 High performance subscription-based form state management for React
The npm package react-final-form receives a total of 206,883 weekly downloads. As such, react-final-form popularity was classified as popular.
We found that react-final-form demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.