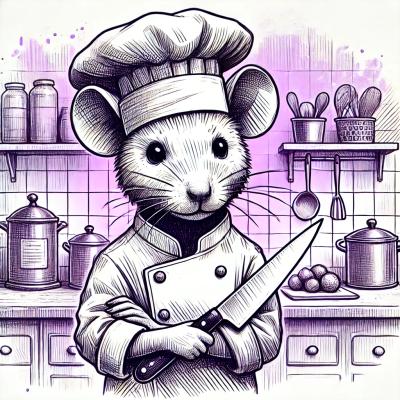
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
react-image-gallery
Advanced tools
React carousel image gallery component with thumbnail and mobile support
react-image-gallery is a flexible and customizable image gallery component for React applications. It allows developers to create responsive and interactive image galleries with features like slide shows, thumbnails, and full-screen mode.
Basic Image Gallery
This code sample demonstrates how to create a basic image gallery using react-image-gallery. The gallery displays a series of images with corresponding thumbnails.
import React from 'react';
import ImageGallery from 'react-image-gallery';
const images = [
{
original: 'https://example.com/image1.jpg',
thumbnail: 'https://example.com/thumb1.jpg',
},
{
original: 'https://example.com/image2.jpg',
thumbnail: 'https://example.com/thumb2.jpg',
},
{
original: 'https://example.com/image3.jpg',
thumbnail: 'https://example.com/thumb3.jpg',
},
];
const MyGallery = () => (
<ImageGallery items={images} />
);
export default MyGallery;
Auto Play Slide Show
This code sample shows how to enable the auto-play feature in the image gallery. The gallery will automatically cycle through the images.
import React from 'react';
import ImageGallery from 'react-image-gallery';
const images = [
{
original: 'https://example.com/image1.jpg',
thumbnail: 'https://example.com/thumb1.jpg',
},
{
original: 'https://example.com/image2.jpg',
thumbnail: 'https://example.com/thumb2.jpg',
},
{
original: 'https://example.com/image3.jpg',
thumbnail: 'https://example.com/thumb3.jpg',
},
];
const MyGallery = () => (
<ImageGallery items={images} autoPlay={true} />
);
export default MyGallery;
Full-Screen Mode
This code sample demonstrates how to enable the full-screen mode in the image gallery. Users can view images in full-screen by clicking the full-screen button.
import React from 'react';
import ImageGallery from 'react-image-gallery';
const images = [
{
original: 'https://example.com/image1.jpg',
thumbnail: 'https://example.com/thumb1.jpg',
},
{
original: 'https://example.com/image2.jpg',
thumbnail: 'https://example.com/thumb2.jpg',
},
{
original: 'https://example.com/image3.jpg',
thumbnail: 'https://example.com/thumb3.jpg',
},
];
const MyGallery = () => (
<ImageGallery items={images} showFullscreenButton={true} />
);
export default MyGallery;
react-photo-gallery is a React component for creating responsive image galleries. It offers a more grid-based layout compared to react-image-gallery and is optimized for performance with features like lazy loading.
react-images is a lightbox component for displaying images in a modal. It focuses on providing a simple and customizable lightbox experience, whereas react-image-gallery offers a more comprehensive gallery solution.
react-slick is a carousel component for React. It is highly customizable and supports various types of content, not just images. It is more versatile in terms of content types compared to react-image-gallery.
linxtion.com/demo/react-image-gallery
React image gallery is a React component for building image galleries and carousels
React Image Gallery requires React 16.0.0 or later.
npm install react-image-gallery
# SCSS
@import "~react-image-gallery/styles/scss/image-gallery.scss";
# CSS
@import "~react-image-gallery/styles/css/image-gallery.css";
Need more example? See example/app.js
import ImageGallery from "react-image-gallery";
const images = [
{
original: "https://picsum.photos/id/1018/1000/600/",
thumbnail: "https://picsum.photos/id/1018/250/150/",
},
{
original: "https://picsum.photos/id/1015/1000/600/",
thumbnail: "https://picsum.photos/id/1015/250/150/",
},
{
original: "https://picsum.photos/id/1019/1000/600/",
thumbnail: "https://picsum.photos/id/1019/250/150/",
},
];
class MyGallery extends React.Component {
render() {
return <ImageGallery items={images} />;
}
}
items
: (required) Array of objects, see example above,
original
- image src urlthumbnail
- image thumbnail src urlfullscreen
- image for fullscreen (defaults to original)originalHeight
- image height (html5 attribute)originalWidth
- image width (html5 attribute)loading
- image loading. Either "lazy" or "eager" (html5 attribute)thumbnailHeight
- image height (html5 attribute)thumbnailWidth
- image width (html5 attribute)thumbnailLoading
- image loading. Either "lazy" or "eager" (html5 attribute)originalClass
- custom image classthumbnailClass
- custom thumbnail classrenderItem
- Function for custom rendering a specific slide (see renderItem below)renderThumbInner
- Function for custom thumbnail renderer (see renderThumbInner below)originalAlt
- image altthumbnailAlt
- thumbnail image altoriginalTitle
- image titlethumbnailTitle
- thumbnail image titlethumbnailLabel
- label for thumbnaildescription
- description for imagesrcSet
- image srcset (html5 attribute)sizes
- image sizes (html5 attribute)bulletClass
- extra class for the bullet of the iteminfinite
: Boolean, default true
lazyLoad
: Boolean, default false
showNav
: Boolean, default true
showThumbnails
: Boolean, default true
thumbnailPosition
: String, default bottom
top, right, bottom, left
showFullscreenButton
: Boolean, default true
useBrowserFullscreen
: Boolean, default true
useTranslate3D
: Boolean, default true
translate
instead of translate3d
css property to transition slidesshowPlayButton
: Boolean, default true
isRTL
: Boolean, default false
showBullets
: Boolean, default false
showIndex
: Boolean, default false
autoPlay
: Boolean, default false
disableThumbnailScroll
: Boolean, default false
disableKeyDown
: Boolean, default false
disableSwipe
: Boolean, default false
disableThumbnailSwipe
: Boolean, default false
onErrorImageURL
: String, default undefined
indexSeparator
: String, default ' / '
, ignored if showIndex
is false
slideDuration
: Number, default 450
swipingTransitionDuration
: Number, default 0
slideInterval
: Number, default 3000
slideOnThumbnailOver
: Boolean, default false
flickThreshold
: Number (float), default 0.4
swipeThreshold
: Number, default 30
stopPropagation
: Boolean, default false
startIndex
: Number, default 0
onImageError
: Function, callback(event)
onThumbnailError
: Function, callback(event)
onThumbnailClick
: Function, callback(event, index)
onBulletClick
: Function, callback(event, index)
onImageLoad
: Function, callback(event)
onSlide
: Function, callback(currentIndex)
onBeforeSlide
: Function, callback(nextIndex)
onScreenChange
: Function, callback(boolean)
onPause
: Function, callback(currentIndex)
onPlay
: Function, callback(currentIndex)
onClick
: Function, callback(event)
onTouchMove
: Function, callback(event) on gallery slide
onTouchEnd
: Function, callback(event) on gallery slide
onTouchStart
: Function, callback(event) on gallery slide
onMouseOver
: Function, callback(event) on gallery slide
onMouseLeave
: Function, callback(event) on gallery slide
additionalClass
: String,
renderCustomControls
: Function, custom controls rendering
_renderCustomControls() {
return <a href='' className='image-gallery-custom-action' onClick={this._customAction.bind(this)}/>
}
renderItem
: Function, custom item rendering
[{thumbnail: '...', renderItem: this.myRenderItem}]
ImageGallery
to completely override renderItem
, see source for renderItem implementationrenderThumbInner
: Function, custom thumbnail rendering
[{thumbnail: '...', renderThumbInner: this.myRenderThumbInner}]
ImageGallery
to completely override _renderThumbInner
, see source for referencerenderLeftNav
: Function, custom left nav component
<LeftNav />
onClick
callback that will slide to the previous itemdisabled
boolean for when the nav is disabledrenderLeftNav: (onClick, disabled) => (
<LeftNav onClick={onClick} disabled={disabled} />
);
renderRightNav
: Function, custom right nav component
<RightNav />
onClick
callback that will slide to the next itemdisabled
boolean for when the nav is disabledrenderRightNav: (onClick, disabled) => (
<RightNav onClick={onClick} disabled={disabled} />
);
renderPlayPauseButton
: Function, play pause button component
<PlayPause />
onClick
callback that will toggle play/pauseisPlaying
boolean for when gallery is playingrenderPlayPauseButton: (onClick, isPlaying) => (
<PlayPause onClick={onClick} isPlaying={isPlaying} />
);
renderFullscreenButton
: Function, custom fullscreen button component
<Fullscreen />
onClick
callback that will toggle fullscreenisFullscreen
argument for when fullscreen is active renderFullscreenButton: (onClick, isFullscreen) => (
<Fullscreen onClick={onClick} isFullscreen={isFullscreen} />
),
useWindowKeyDown
: Boolean, default true
true
, listens to keydown events on window (window.addEventListener)false
, listens to keydown events on image gallery element (imageGalleryElement.addEventListener)The following functions can be accessed using refs
play()
: plays the slidespause()
: pauses the slidestoggleFullScreen()
: toggles full screenslideToIndex(index)
: slides to a specific indexgetCurrentIndex()
: returns the current indexEach pull request (PR) should be specific and isolated to the issue you're trying to fix. Please do not stack features, chores, refactors, or enhancements in one PR. Describe your feature/implementation in the PR. If you're unsure whether it's useful or if it involves a major change, please open an issue first and seek feedback.
git clone https://github.com/xiaolin/react-image-gallery.git
cd react-image-gallery
npm install --global yarn
yarn install
yarn start
Then open localhost:8001
in a browser.
MIT
FAQs
React carousel image gallery component with thumbnail and mobile support
The npm package react-image-gallery receives a total of 168,758 weekly downloads. As such, react-image-gallery popularity was classified as popular.
We found that react-image-gallery demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.