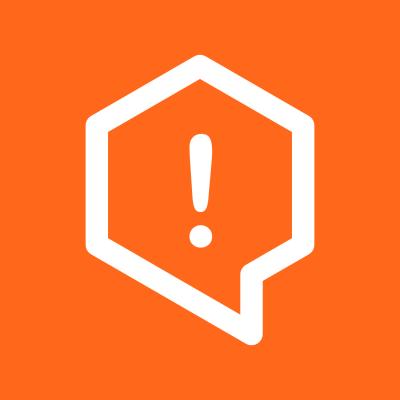
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
react-joyride
Advanced tools
react-joyride is a React component that helps you create guided tours for your web applications. It allows you to highlight elements, provide step-by-step instructions, and enhance user onboarding experiences.
Creating a Basic Tour
This code demonstrates how to create a basic tour with react-joyride. It defines a series of steps, each with a target element and content, and then renders the Joyride component with these steps.
import React from 'react';
import Joyride from 'react-joyride';
const App = () => {
const steps = [
{
target: '.my-first-step',
content: 'This is my first step!'
},
{
target: '.my-second-step',
content: 'This is my second step!'
}
];
return (
<div>
<Joyride steps={steps} />
<div className="my-first-step">First Step</div>
<div className="my-second-step">Second Step</div>
</div>
);
};
export default App;
Customizing Tour Appearance
This code shows how to customize the appearance of the tour. You can change the primary color, z-index, and other styles to match your application's design.
import React from 'react';
import Joyride, { STATUS } from 'react-joyride';
const App = () => {
const steps = [
{
target: '.my-first-step',
content: 'This is my first step!',
styles: {
options: {
zIndex: 10000,
}
}
}
];
return (
<div>
<Joyride
steps={steps}
styles={{
options: {
primaryColor: '#e91e63',
zIndex: 10000,
}
}}
/>
<div className="my-first-step">First Step</div>
</div>
);
};
export default App;
Controlling Tour Programmatically
This code demonstrates how to control the tour programmatically. You can start the tour with a button click and handle the tour's status changes using a callback function.
import React, { useState } from 'react';
import Joyride, { STATUS } from 'react-joyride';
const App = () => {
const [run, setRun] = useState(false);
const steps = [
{
target: '.my-first-step',
content: 'This is my first step!'
}
];
const handleJoyrideCallback = (data) => {
const { status } = data;
if ([STATUS.FINISHED, STATUS.SKIPPED].includes(status)) {
setRun(false);
}
};
return (
<div>
<button onClick={() => setRun(true)}>Start Tour</button>
<Joyride
steps={steps}
run={run}
callback={handleJoyrideCallback}
/>
<div className="my-first-step">First Step</div>
</div>
);
};
export default App;
reactour is another React library for creating guided tours. It offers a simple API and is highly customizable. Compared to react-joyride, reactour is more lightweight but may lack some advanced features.
shepherd.js is a JavaScript library for creating guided tours that can be used with React. It provides a more flexible and powerful API compared to react-joyride, but it requires more setup and configuration.
intro.js is a standalone JavaScript library for creating step-by-step guides and feature introductions. It can be integrated with React and offers a wide range of customization options. It is more feature-rich but also more complex to use compared to react-joyride.
View the demo here.
npm install --save react-joyride
Include Joyride
in the parent component before anything else.
var react = require('react');
var Joyride = require('react-joyride');
var App = React.createClass({
render: function () {
return (
<div className="app">
<Joyride ref="joyride" steps={this.state.steps} debug={true} ... />
<YourComponents .../>
</div>
);
}
...
});
Don't forget to pass a ref
to the component.
If your are using SCSS (and you should):
@include '../path/to/node-modules/react-joyride/lib/styles/react-joyride'
Or include this directly in your html:
<link rel="stylesheet" href="react-joyride/lib/styles/react-joyride.css" type="text/css">
Add a custom function to include steps and/or tooltips in your parent component
addSteps: function (steps) {
let joyride = this.refs.joyride;
if (!Array.isArray(steps)) {
steps = [steps];
}
if (!steps.length) {
return false;
}
this.setState(function(currentState) {
currentState.steps = currentState.steps.concat(joyride.parseSteps(steps));
return currentState;
});
}
addTooltip: function (data) {
this.refs.joyride.addTooltip(data);
}
Add steps after your components are mounted.
componentDidMount: function () {
this.addSteps({...}); // or this.addTooltip({...});
// or using props in your child components
this.props.addSteps({...});
}
...
render: function () {
return (
<Joyride ref="joyride" .../>
<ChildComponent addSteps={this.addSteps} addTooltip={this.addTooltip} />
);
}
Or you can start the tour after a criteria is met
componentDidUpdate (prevProps, prevState) {
if (!prevState.ready && this.state.ready) {
this.refs.joyride.start();
}
},
Please refer to the source code of the demo if you need a practical example.
You can change the initial options passing props to the component. All optional.
debug {bool}: Console.log Joyride's inner actions. Defaults to false
keyboardNavigation {bool}: Toggle keyboard navigation (esc, space bar, return). Defaults to true
locale {object}: The strings used in the tooltip. Defaults to { back: 'Back', close: 'Close', last: 'Last', next: 'Next', skip: 'Skip' }
resizeDebounce {bool}: Delay the reposition of the current step while the window is being resized. Defaults to false
resizeDebounceDelay {number}: The amount of delay for the resizeDebounce
callback. Defaults to 200
scrollOffset {number}: The scrollTop offset used in scrollToSteps
. Defaults to 20
scrollToSteps {bool}: Scroll the page to the next step if needed. Defaults to true
scrollToFirstStep {bool}: Scroll the page for the first step. Defaults to false
showBackButton {bool}: Display a back button. Defaults to true
showOverlay {bool}: Display an overlay with holes above your steps (for tours only). Defaults to true
showSkipButton {bool}: Display a link to skip the tour. It will trigger the completeCallback
if it was defined. Defaults to false
showStepsProgress {bool}: Display the tour progress in the next button e.g. 2/5 in guided
tours. Defaults to false
steps {array}: The tour's steps. Defaults to []
tooltipOffset {number}: The tooltip offset from the target. Defaults to 30
type {string}: The type of your presentation. It can be guided
(played sequencially with the Next button) or single
. Defaults to guided
completeCallback {function}: It will be called after an user has completed all the steps or skipped the tour and passes two parameters, the steps {array}
and if the tour was skipped {boolean}
. Defaults to undefined
stepCallback {function}: It will be called after each step and passes the completed step {object}
. Defaults to undefined
Example:
<Joyride ref="joyride" steps={this.state.steps} debug={true} type="single"
stepCallback={this._stepCallback} ... />
Call this method to start the tour.
autorun
{boolean} - Starts the tour with the first tooltip opened.Call this method to stop/pause the tour.
Call this method to reset the tour iteration back to 0
restart
{boolean} - Starts the new tour right awayRetrieve the current progress of your tour. The object returned looks like this:
{
index: 2,
percentageComplete: 50,
step: {
title: "...",
text: "...",
selector: "...",
position: "...",
...
}
}}
Parse the incoming steps, check if it's already rendered and returns an array with valid items
steps
{array|object}var steps = this.refs.joyride.parseSteps({
title: 'Title',
text: 'description',
selector: ReactDOM.findDOMNode(this.refs.node),
position: 'top'
});
// steps
[{
title: 'Title',
text: 'description',
selector: '[data-reactid="0.0.1.0"]',
position: 'top'
}]
There are a few usable options but you can pass extra parameters.
title
: The title of the tooltiptext
: The tooltip's body text (required)selector
: The target DOM selector of your feature (required)position
: Relative position of you beacon and tooltip. It can be one of these:top
, top-left
, top-right
, bottom
, bottom-left
, bottom-right
, right
and left
. This defaults to top
.Extra option for standalone tooltips
trigger
: The DOM element that will trigger the tooltipAs of version 1.x you can style tooltips independently with these options: backgroundColor
, borderRadius
, color
, mainColor
, textAlign
and width
.
Also you can style button
, skip
, back
and close
individually using standard style options. And beacon
inner and outer colors.
Example:
{
title: 'First Step',
text: 'Start using the joyride',
selector: '.first-step',
position: 'bottom-left',
style: {
backgroundColor: 'rgba(0, 0, 0, 0.8)',
borderRadius: '0',
color: '#fff',
mainColor: '#ff4456',
textAlign: 'center',
width: '29rem',
beacon: {
inner: '#000',
outer: '#000'
},
button: {
display: 'none'
// or any style attribute
},
skip: {
color: '#f04'
},
...
},
// extra params...
name: 'my-first-step',
parent: 'MyComponentName'
}
$joyride-color
: The base color. Defaults to #f04
$joyride-zindex
: Defaults to 1500
$joyride-overlay-color
: Defaults to rgba(#000, 0.5)
$joyride-beacon-color
: Defaults to $joyride-color
$joyride-beacon-size
: Defaults to 36px
$joyride-hole-border-radius
: Defaults to 4px
$joyride-hole-shadow
: Defaults to 0 0 15px rgba(#000, 0.5)
$joyride-tooltip-arrow-size
: You must use even numbers to avoid half-pixel inconsistencies. Defaults to 28px
$joyride-tooltip-bg-color
: Defaults to #fff
$joyride-tooltip-border-radius
: Defaults to 4px
$joyride-tooltip-color
: The header and text color. Defaults to #555
$joyride-tooltip-font-size
: Defaults to 16px
$joyride-tooltip-padding
: Defaults to 20px
$joyride-tooltip-shadow
: Sass list for drop-shadow. Defaults to (x: 1px, y: 2px, blur: 3px, color: rgba(#000, 0.3))
$joyride-tooltip-width
: Sass list of Mobile / Tablet / Desktop sizes. Defaults to (290px, 360px, 450px)
$joyride-header-color
: Defaults to $joyride-tooltip-header-color
$joyride-header-font-size
: Defaults to 20px
$joyride-header-border-color
: Defaults to $joyride-color
$joyride-header-border-width
: Defaults to 1px
$joyride-button-bg-color
: Defaults to $joyride-color
$joyride-button-color
: Defaults to #fff
$joyride-button-border-radius
: Defaults to 4px
$joyride-back-button-color
: Defaults to $joyride-color
$joyride-skip-button-color
: Defaults to #ccc
$joyride-close
: Sass list for the close button: Defaults to (color: rgba($joyride-tooltip-color, 0.5), size: 30px, top: 10px, right: 10px)
$joyride-close-visible
: Default to true
;Copyright © 2015 Gil Barbara - MIT License
Inspired by react-tour-guide and jquery joyride tour
FAQs
Create guided tours for your apps
The npm package react-joyride receives a total of 250,803 weekly downloads. As such, react-joyride popularity was classified as popular.
We found that react-joyride demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.