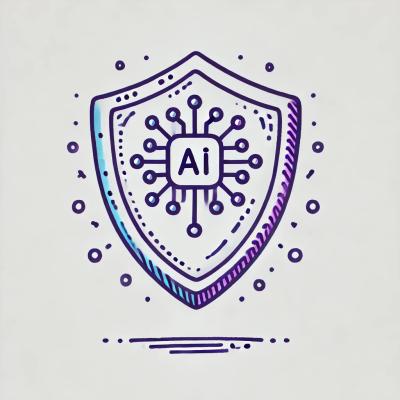
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
react-map-gl
Advanced tools
react-map-gl is a React wrapper for Mapbox GL JS, which is a powerful library for interactive, customizable maps. It allows developers to integrate Mapbox maps into their React applications with ease, providing a range of features for map rendering, user interaction, and data visualization.
Basic Map Rendering
This code demonstrates how to render a basic map using react-map-gl. It sets up a map centered on a specific latitude and longitude with a given zoom level. The map style is set to 'streets-v11' from Mapbox, and the viewport state is managed to allow for user interaction.
```jsx
import React from 'react';
import ReactMapGL from 'react-map-gl';
const Map = () => {
const [viewport, setViewport] = React.useState({
latitude: 37.7577,
longitude: -122.4376,
zoom: 8
});
return (
<ReactMapGL
{...viewport}
width="100%"
height="100%"
mapStyle="mapbox://styles/mapbox/streets-v11"
onViewportChange={nextViewport => setViewport(nextViewport)}
mapboxApiAccessToken={process.env.REACT_APP_MAPBOX_TOKEN}
/>
);
};
export default Map;
```
Adding Markers
This code sample shows how to add a marker to the map. The Marker component is used to place a marker at a specific latitude and longitude. In this example, a simple red dot is used as the marker.
```jsx
import React from 'react';
import ReactMapGL, { Marker } from 'react-map-gl';
const MapWithMarkers = () => {
const [viewport, setViewport] = React.useState({
latitude: 37.7577,
longitude: -122.4376,
zoom: 8
});
return (
<ReactMapGL
{...viewport}
width="100%"
height="100%"
mapStyle="mapbox://styles/mapbox/streets-v11"
onViewportChange={nextViewport => setViewport(nextViewport)}
mapboxApiAccessToken={process.env.REACT_APP_MAPBOX_TOKEN}
>
<Marker latitude={37.7577} longitude={-122.4376}>
<div style={{ backgroundColor: 'red', width: '10px', height: '10px', borderRadius: '50%' }} />
</Marker>
</ReactMapGL>
);
};
export default MapWithMarkers;
```
Handling Map Events
This example demonstrates how to handle map events, such as clicks. The handleClick function is triggered when the map is clicked, displaying an alert with the coordinates of the click.
```jsx
import React from 'react';
import ReactMapGL from 'react-map-gl';
const MapWithEvents = () => {
const [viewport, setViewport] = React.useState({
latitude: 37.7577,
longitude: -122.4376,
zoom: 8
});
const handleClick = (event) => {
alert(`Clicked at ${event.lngLat}`);
};
return (
<ReactMapGL
{...viewport}
width="100%"
height="100%"
mapStyle="mapbox://styles/mapbox/streets-v11"
onViewportChange={nextViewport => setViewport(nextViewport)}
mapboxApiAccessToken={process.env.REACT_APP_MAPBOX_TOKEN}
onClick={handleClick}
/>
);
};
export default MapWithEvents;
```
Leaflet is a popular open-source JavaScript library for interactive maps. It is lightweight and easy to use, with a wide range of plugins available for additional functionality. Compared to react-map-gl, Leaflet is more lightweight but may not offer the same level of performance and customization as Mapbox GL JS.
google-maps-react is a library for integrating Google Maps into React applications. It provides a set of React components for Google Maps, allowing for easy map rendering and interaction. While it offers robust features and the reliability of Google Maps, it may not provide the same level of customization and styling options as react-map-gl.
react-leaflet is a React wrapper for Leaflet, providing a set of React components for Leaflet maps. It combines the simplicity of Leaflet with the power of React, making it easy to create interactive maps in React applications. Compared to react-map-gl, react-leaflet is more lightweight but may not offer the same advanced features and performance.
react-map-gl
is a suite of React components designed to provide a React API for mapbox-gl or maplibre-gl. More information in the online documentation.
See our Design Philosophy.
Using react-map-gl
requires react >= 16.3
.
# Using Maplibre
npm install react-map-gl maplibre-gl
or
# Using Mapbox
npm install react-map-gl mapbox-gl
// Using Maplibre
import * as React from 'react';
import Map from 'react-map-gl/maplibre';
function App() {
return (
<Map
initialViewState={{
longitude: -122.4,
latitude: 37.8,
zoom: 14
}}
style={{width: 600, height: 400}}
mapStyle="https://api.maptiler.com/maps/streets/style.json?key=<Maptiler access token>"
/>
);
}
or
// Using Mapbox
import * as React from 'react';
import Map from 'react-map-gl';
function App() {
return (
<Map
mapboxAccessToken="<Mapbox access token>"
initialViewState={{
longitude: -100,
latitude: 40,
zoom: 3.5
}}
style={{width: 600, height: 400}}
mapStyle="mapbox://styles/mapbox/streets-v9"
/>
);
}
Learn more with in our Getting Started guide.
Starting with v2.0, mapbox-gl requires a Mapbox token for any usage, with or without the Mapbox data service. See about Mapbox tokens for your options.
To show maps from a service such as Mapbox you will need to register on their website in order to retrieve an access token required by the map component, which will be used to identify you and start serving up map tiles. The service will be free until a certain level of traffic is exceeded.
There are several ways to provide a token to your app, as showcased in some of the example folders:
mapboxAccessToken
prop to the map componentMapboxAccessToken
environment variable (or set REACT_APP_MAPBOX_ACCESS_TOKEN
if you are using Create React App)?access_token=TOKEN
See contribution guide.
react-map-gl is part of vis.gl, an OpenJS Foundation project.
Development is also supported by
FAQs
React components for MapLibre GL JS and Mapbox GL JS
The npm package react-map-gl receives a total of 333,864 weekly downloads. As such, react-map-gl popularity was classified as popular.
We found that react-map-gl demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.