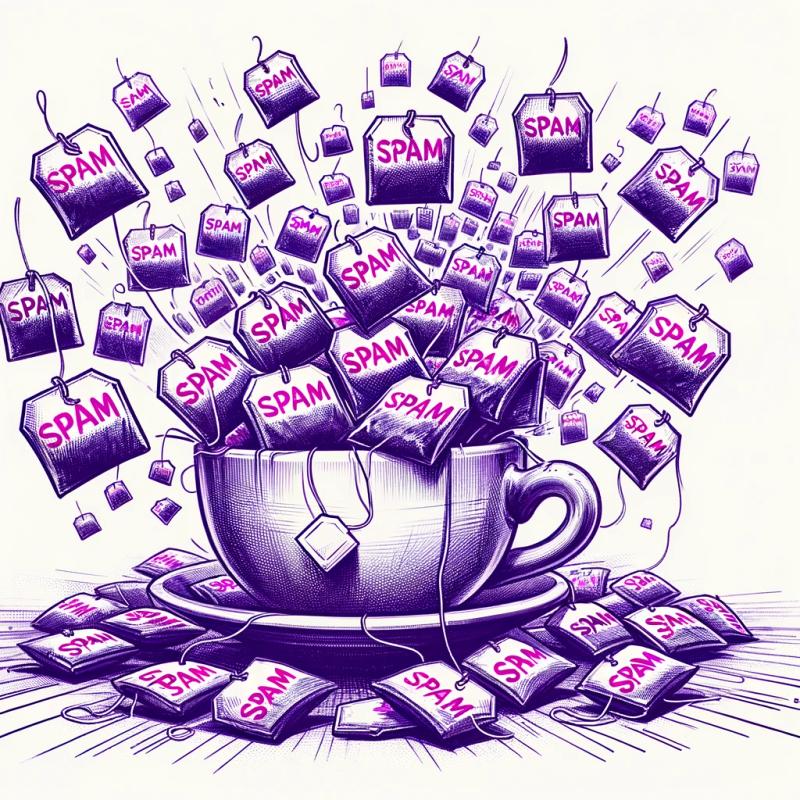
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-morphing-modal
Advanced tools
Readme
$ npm install --save react-morphing-modal
//or
$ yarn add react-morphing-modal
The library expose 2 ways to display the modal:
getTriggerProps
andopen
. For the basic use casegetTriggerProps
is fine. But for most of the cases usingopen
is the way to go. Please look at the api for more details.
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps } = useModal();
return (
<div>
<button {...getTriggerProps()}>Show modal</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
If you just want to open the modal you can stick with getTriggerProps
.
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
const Button = props => (
<button {...props.getTriggerProps()}>Show modal</button>
);
function App() {
const { modalProps, getTriggerProps } = useModal();
return (
<div>
<Button getTriggerProps={getTriggerProps} />
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
Most of the time you need to perform different task when a user click a button like calling an api. In that case
use the open
method as follow.
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
const Button = ({ openModal }) => {
const btnRef = useRef(null);
function handleClick() {
// do some complicated stuff
openModal(btnRef);
}
return (
<button ref={btnRef} onClick={handleClick}>
Show modal
</button>
);
};
function App() {
const { modalProps, open } = useModal();
return (
<div>
<Button openModal={open} />
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal, open } = useModal();
const triggerRef = useRef(null);
const handleTrigger3 = () => open(triggerRef);
return (
<div>
<button {...getTriggerProps()}>Trigger 1</button>
<button {...getTriggerProps()}>Trigger 2</button>
<button ref={triggerRef} onClick={handleTrigger3}>
Trigger 3
</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal();
return (
<div>
<button {...getTriggerProps('trigger1')}>Trigger 1</button>
<button {...getTriggerProps('trigger2')}>Trigger 2</button>
{/* You can also pass an object */}
<button {...getTriggerProps({ id: 'trigger3' })}>Trigger 3</button>
<span>{activeModal}</span>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal({
onOpen() {
console.log('onOpen');
},
onClose() {
console.log('onClose');
},
});
return (
<div>
<button {...getTriggerProps()}>Trigger</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal();
return (
<div>
<button
{...getTriggerProps({
onOpen: () => console.log('open'),
onClose: () => console.log('close'),
})}
>
Trigger
</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
By default, the modal background is the same as the trigger one. However, you are free to define yours.
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal({
background: '#666',
});
return (
<div>
<button {...getTriggerProps()}>Trigger</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal();
return (
<div>
<button
{...getTriggerProps({
background: '#666',
})}
>
Trigger
</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
By default, the onClick
event is used on the trigger.
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal({
event: 'onDoubleClick',
});
return (
<div>
<button {...getTriggerProps()}>Trigger</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal();
return (
<div>
<button
{...getTriggerProps({
event: 'onDoubleClick',
})}
>
Trigger
</button>
<Modal {...modalProps}>Hello World</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal();
return (
<div>
<button {...getTriggerProps()}>Trigger</button>
<Modal {...modalProps} closeButton={false}>
Hello World
</Modal>
</div>
);
}
import React from 'react';
import { useModal, Modal } from 'react-morphing-modal';
import 'react-morphing-modal/dist/ReactMorphingModal.css';
function App() {
const { modalProps, getTriggerProps, activeModal } = useModal();
return (
<div>
<button {...getTriggerProps()}>Trigger</button>
<Modal {...modalProps} padding={false}>
Hello World
</Modal>
</div>
);
}
import { useModal } from 'react-morphing-modal';
const { open, close, activeModal, modalProps, getTriggerProps } = useModal({
event: 'onClick',
onOpen: () => console.log('will open'),
onClose: () => console.log('will close'),
background: 'purple',
});
Props | Type | Default | Description |
---|---|---|---|
event | string | onClick | Any valid react dom event: onClick, onDoubleClick, etc... |
onOpen | function | - | A function to call when the modal will open |
onClose | function | - | A function to call when the modal will close |
background | string | - | Any valid css background: #fffff, rgb(1,1,1), etc |
open
have 2 signatures
import { useModal } from 'react-morphing-modal';
const { open } = useModal();
// pass a ref to your trigger
const myRef = React.useRef(null);
//somewhere in your app
<MyComponent ref={myRef} />;
open(myRef, 'modalId');
open(myRef, {
id: 'modalId',
onOpen: () => console.log('will open'),
onClose: () => console.log('will close'),
background: 'purple',
});
Props | Type | Default | Description |
---|---|---|---|
id | string | number | symbol | null | - | Specify a modal id. It will be assigned to activeModal |
onOpen | function | - | A function to call when the modal will open |
onClose | function | - | A function to call when the modal will close |
background | string | - | Any valid css background: #fffff, rgb(1,1,1), etc |
close
does not require any options.
import { useModal } from 'react-morphing-modal';
const { close } = useModal();
close();
activeModal
hold the displayed modalId. activeModal
is set to null
if not id has been used.
import { useModal } from 'react-morphing-modal';
const { open, activeModal } = useModal();
open(triggerRef, 'modalId');
console, log(activeModal); // print modalId
modalProps
hold the props that must be passed to the Modal component.
import { useModal, Modal } from 'react-morphing-modal';
const { modalProps } = useModal();
<Modal {...modalProps} />;
getTriggerProps
is a convenient method for the simple use case. Under the hood a ref is created and bound to open
.
getTriggerProps
has also 2 signatures.
import { useModal } from 'react-morphing-modal';
const { getTriggerProps } = useModal();
<button {...trigger('modalId')}>trigger</button>;
<button {...trigger({
id: 'modalId',
event: 'onDoubleClcik'
onOpen: () => console.log('will open'),
onClose: () => console.log('will close'),
background: 'purple'
})}>trigger</button>
Props | Type | Default | Description |
---|---|---|---|
id | string | number | symbol | null | - | Specify a modal id. It will be assigned to activeModal |
event | string | onClick | Any valid react dom event: onClick, onDoubleClick, etc... |
onOpen | function | - | A function to call when the modal will open |
onClose | function | - | A function to call when the modal will close |
background | string | - | Any valid css background: #fffff, rgb(1,1,1), etc |
import { Modal } from 'react-morphing-modal';
<Modal closeButton={true} padding={true}>
hello
</Modal>;
Props | Type | Default | Description |
---|---|---|---|
closeButton | boolean | true | Display a close button |
padding | boolean | true | Remove the default padding. Useful when doing some customisation |
![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|
❌ | ✅ | ✅ | ✅ | ✅ |
You can find the release note for the latest release here
You can browse them all here
Show your ❤️ and support by giving a ⭐. Any suggestions are welcome ! Take a look at the contributing guide.
You can also find me on reactiflux. My pseudo is Fadi.
Licensed under MIT
FAQs
React morphing modal! The easiest way to be fancy!
The npm package react-morphing-modal receives a total of 687 weekly downloads. As such, react-morphing-modal popularity was classified as not popular.
We found that react-morphing-modal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.