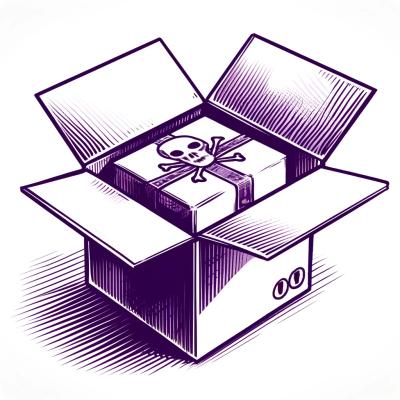
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
react-native-bluetooth-state-manager
Advanced tools
The only purpose of this library is to manage the Bluetooth state. Not more, not less.
If you need further functionality like connecting and communicating to a device, please look at react-native-ble-plx.
Using Yarn: (recommended)
yarn add react-native-bluetooth-state-manager
Using npm:
npm install react-native-bluetooth-state-manager --save
Beginning from 0.60 you don't need to link it anymore. For more see here.
iOS
For iOS you just need to install the pods.
cd ios/
pod install
cd ..
Run react-native link react-native-bluetooth-state-manager
Append the following lines to your ios/Podfile
:
target '<your-project>' do
...
+ pod 'RNBluetoothStateManager', :path => '../node_modules/react-native-bluetooth-state-manager'
end
Libraries
➜ Add Files to [your project's name]
node_modules
➜ react-native-bluetooth-state-manager
and add RNBluetoothStateManager.xcodeproj
libRNBluetoothStateManager.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)<android/settings.gradle
:...
include ':app'
+ include ':react-native-bluetooth-state-manager'
+ project(':react-native-bluetooth-state-manager').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-bluetooth-state-manager/android')
android/app/build.gradle
:dependencies {
+ compile project(':react-native-bluetooth-state-manager')
...
compile "com.facebook.react:react-native:+" // From node_modules
}
android/app/src/main/java/[...]/MainApplication.java
+ import de.patwoz.rn.bluetoothstatemanager.RNBluetoothStateManagerPackage;
public class MainApplication extends Application implements ReactApplication {
// ...
private final ReactNativeHost mReactNativeHost = new ReactNativeHost(this) {
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
+ new RNBluetoothStateManagerPackage()
);
}
};
}
import BluetoothStateManager from 'react-native-bluetooth-state-manager';
iOS
You must provide a short description why you need access to bluetooth in your app. Otherwise your app will crash when requesting for bluetooth:
This app has crashed because it attempted to access privacy-sensitive data without a usage description. The app's Info.plist must contain an NSBluetoothAlwaysUsageDescription key with a string value explaining to the user how the app uses this data.
An example you will find in example/app/ExampleWithApi.js
Method | Return Type | OS | Description |
---|---|---|---|
getState() | Promise<String> | Android, iOS | Returns the current state of the bluetooth service. |
onStateChange(listener, emitCurrentState) | Subscription | Android, iOS | Listen for bluetooth state changes. |
openSettings() | Promise<null> | Android, iOS | Opens the bluetooth settings. Please see below for more details. |
requestToEnable() | Promise<Boolean> | Android | Show a dialog that allows the user to turn on Bluetooth. |
enable() | Promise<null> | Android | Enables Bluetooth without further user interaction. |
disable() | Promise<null> | Android | Disables Bluetooth without further user interaction. |
Important: To use enable()
and disable()
on android, you have to add BLUETOOTH_ADMIN
permission to your AndroidManifest.xml
:
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
+ <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
</manifest>
Returns the current state of the bluetooth service.
BluetoothStateManager.getState().then((bluetoothState) => {
switch (bluetoothState) {
case 'Unknown':
case 'Resetting':
case 'Unsupported':
case 'Unauthorized':
case 'PoweredOff':
case 'PoweredOn':
default:
break;
}
});
Listen for bluetooth state changes.
BluetoothStateManager.onStateChange((bluetoothState) => {
// do something...
}, true /*=emitCurrentState*/);
Opens the bluetooth settings.
Tested:
Opens the settings page of the app. Please see here.
BluetoothStateManager.openSettings();
Show a dialog that allows the user to turn on Bluetooth. More here: Android documentation.
BluetoothStateManager.requestToEnable().then((result) => {
// result === true -> user accepted to enable bluetooth
// result === false -> user denied to enable bluetooth
});
Enables Bluetooth without further user interaction
BLUETOOTH_ADMIN
permission.BluetoothStateManager.enable().then((result) => {
// do something...
});
Disables Bluetooth without further user interaction
This function is only on android available.
Needs the BLUETOOTH_ADMIN
permission.
BluetoothStateManager.disable().then((result) => {
// do something...
});
Name | Description |
---|---|
EVENT_BLUETOOTH_STATE_CHANGE | Callback for when the bluetooth state changed |
Callback for when the bluetooth state changed
BluetoothStateManager.addEventListener(
BluetoothStateManager.EVENT_BLUETOOTH_STATE_CHANGE,
(bluetoothState) => {
// do something...
}
);
// recommended: use the `onStateChange` function.
The declarative way uses the new context api of React 16.3.
import { BluetoothState } from 'react-native-bluetooth-state-manager';
<BluetoothState>
Name | Value Type | Default value | Description |
---|---|---|---|
emitCurrentState | Boolean | true | If true, current state will be emitted. |
onChange | Function | undefined | Callback which emits the current state (first argument) change and the previous state (second argument). |
children | Function or any | undefined |
<BluetoothState.PoweredOn>
The children
prop of this component will rendered only when bluetooth is turned on.
<BluetoothState.PoweredOff>
The children
prop of this component will rendered only when bluetooth is turned off.
<BluetoothState.Resetting>
The children
prop of this component will rendered only when bluetooth state is changing.
<BluetoothState.Unauthorized>
The children
prop of this component will rendered only when the app doesn't have the permission to use bluetooth.
<BluetoothState.Unsupported>
The children
prop of this component will rendered only when the device doesn't support bluetooth.
<BluetoothState.Unknown>
The children
prop of this component will rendered only when the bluetooth state is unknown.
An example you will find in example/app/ExampleWithDeclarativeApi.js
Each component has access to the same context as shown below.
{
bluetoothState: String,
openSettings: Function,
requestToEnable: Function,
enable: Function,
disable: Function,
}
children
prop as function:<BluetoothState>
{({ bluetoothState, openSettings, requestToEnable, enable, disable }) => {
// show something ...
return <View />;
}}
</BluetoothState>
import { BluetoothState } from 'react-native-bluetooth-state-manager';
<BluetoothState>
<BluetoothState.PoweredOn>
<Text>This will rendered only when bluetooth is turned on.</Text>
</BluetoothState.PoweredOn>
<BluetoothState.PoweredOff>
{({ requestToEnable, openSettings }) => (
<View>
<Text>This will rendered only when bluetooth is turned off.</Text>
<Button
title="This will rendered only when bluetooth is turned off."
onPress={Platform.OS === 'android' ? requestToEnable : openSettings}
/>
</View>
)}
</BluetoothState.PoweredOff>
<BluetoothState.Resetting>
<ActivityIndicator />
</BluetoothState.Resetting>
<BluetoothState.Unauthorized>
<Text>This will rendered only when bluetooth permission is not granted.</Text>
</BluetoothState.Unauthorized>
<BluetoothState.Unsupported>
<Text>This will rendered only when bluetooth is not supported.</Text>
</BluetoothState.Unsupported>
<BluetoothState.Unknown>
<Text>You have a really strange phone.</Text>
</BluetoothState.Unknown>
</BluetoothState>;
Because it's to over bloated for my purpose. In several of my projects I'm working on, I had to integrate several third-party SDK which communicates with different bluetooth devices (on the native side). So the only functionality I needed there (on the javascript side), was to check if the bluetooth is enabled to start the third-party SDK.
MIT
FAQs
Manage the bluetooth state of your device
The npm package react-native-bluetooth-state-manager receives a total of 6,195 weekly downloads. As such, react-native-bluetooth-state-manager popularity was classified as popular.
We found that react-native-bluetooth-state-manager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.