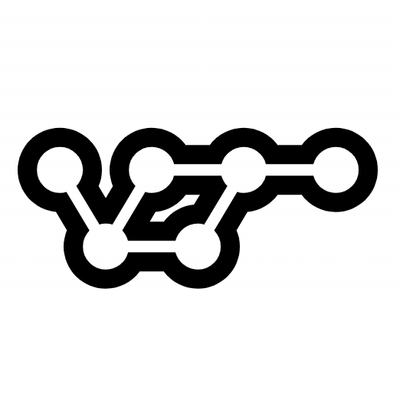
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-native-pdf-lib
Advanced tools
$ npm install react-native-pdf-lib --save
$ react-native link react-native-pdf-lib
build.gradle
file:
android {
...
dexOptions {
jumboMode = true
}
...
}
Libraries
➜ Add Files to [your project's name]
node_modules
➜ react-native-pdf-lib
and add RNPdfLib.xcodeproj
libRNPdfLib.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)<android/app/src/main/java/[...]/MainActivity.java
import com.reactlibrary.PdfLibPackage;
to the imports at the top of the filenew PdfLibPackage()
to the list returned by the getPackages()
methodandroid/settings.gradle
:
include ':react-native-pdf-lib'
project(':react-native-pdf-lib').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-pdf-lib/android')
android/app/build.gradle
:
compile project(':react-native-pdf-lib')
build.gradle
file:
android {
...
// Add this section:
dexOptions {
jumboMode = true
}
...
}
The API is currently in active development, and all aspects of it are subject to breaking changes in future releases.
// Import from 'react-native-pdf-lib'
import PDFLib, { PDFDocument, PDFPage } from 'react-native-pdf-lib';
// Create a PDF page with text and rectangles
const page1 = PDFPage
.create()
.setMediaBox(200, 200)
.addText('You can add text and rectangles to the PDF!', {
color: '#007386',
position: { x: 5, y: 235 },
})
.addRectangle(25, 25, 150, 150, { color: '#FF99CC' })
.addRectangle(50, 50, 100, 100, { color: '#33CCFF' })
.addRectangle(75, 75, 50, 50, { color: '#99FFCC' });
// Create a PDF page with text and an image
const jpgPath = // Path to a JPG image on the file system...
const page2 = PDFPage
.create()
.setMediaBox(250, 250)
.addText('You can add JPG images too!')
.addImage(jpgPath, 'jpg', {
x: 5,
y: 125,
width: 200,
height: 100,
});
// Create a new PDF in your app's private Documents directory
const docsDir = await PDFLib.getDocumentsDir();
const pdfPath = `${docsDir}/sample.pdf`;
PDFDocument
.create(path)
.addPages(page1, page2)
.write() // Returns a promise that resolves with the PDF's path
.then(path => {
console.log('PDF created at: ' + path);
// Do stuff with your shiny new PDF!
});
FAQs
A react native library for handling generating PDFs
The npm package react-native-pdf-lib receives a total of 301 weekly downloads. As such, react-native-pdf-lib popularity was classified as not popular.
We found that react-native-pdf-lib demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.