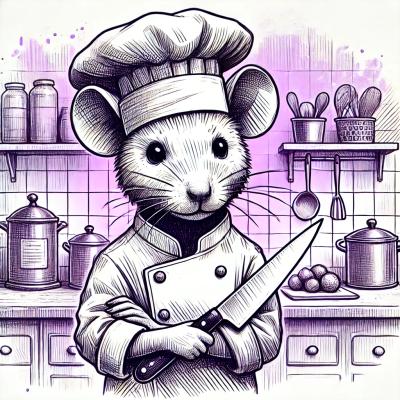
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
react-quill
Advanced tools
react-quill is a React component for Quill, a powerful rich text editor. It provides a customizable and extensible interface for creating and editing content with a variety of formatting options.
Basic Usage
This code demonstrates the basic usage of react-quill. It imports the necessary modules, sets up a state to hold the editor's content, and renders the ReactQuill component.
import React, { useState } from 'react';
import ReactQuill from 'react-quill';
import 'react-quill/dist/quill.snow.css';
function MyEditor() {
const [value, setValue] = useState('');
return (
<ReactQuill value={value} onChange={setValue} />
);
}
export default MyEditor;
Custom Toolbar
This code demonstrates how to create a custom toolbar for the react-quill editor. It defines a CustomToolbar component and uses it in the MyEditor component, specifying the custom toolbar in the modules prop.
import React, { useState } from 'react';
import ReactQuill, { Quill } from 'react-quill';
import 'react-quill/dist/quill.snow.css';
const CustomToolbar = () => (
<div id="toolbar">
<button className="ql-bold">Bold</button>
<button className="ql-italic">Italic</button>
<select className="ql-color">
<option value="red"></option>
<option value="green"></option>
<option value="blue"></option>
</select>
</div>
);
function MyEditor() {
const [value, setValue] = useState('');
return (
<div>
<CustomToolbar />
<ReactQuill value={value} onChange={setValue} modules={{ toolbar: '#toolbar' }} />
</div>
);
}
export default MyEditor;
Formats and Modules
This code demonstrates how to specify custom formats and modules for the react-quill editor. It defines the formats and modules arrays and passes them as props to the ReactQuill component.
import React, { useState } from 'react';
import ReactQuill from 'react-quill';
import 'react-quill/dist/quill.snow.css';
const formats = [
'header', 'bold', 'italic', 'underline', 'strike', 'blockquote',
'list', 'bullet', 'indent',
'link', 'image'
];
const modules = {
toolbar: [
[{ 'header': '1' }, { 'header': '2' }, { 'font': [] }],
[{ 'list': 'ordered'}, { 'list': 'bullet' }],
['bold', 'italic', 'underline', 'strike', 'blockquote'],
['link', 'image'],
[{ 'align': [] }]
]
};
function MyEditor() {
const [value, setValue] = useState('');
return (
<ReactQuill value={value} onChange={setValue} modules={modules} formats={formats} />
);
}
export default MyEditor;
draft-js is a framework for building rich text editors in React, developed by Facebook. It provides a more programmatic approach to managing editor state and content, allowing for extensive customization and control over the editor's behavior. Compared to react-quill, draft-js requires more setup and configuration but offers greater flexibility.
slate is another highly customizable framework for building rich text editors in React. It provides a more modern and flexible approach to handling editor state and content, with a focus on immutability and functional programming. Slate is more complex to set up compared to react-quill but offers extensive customization options and control over the editor's behavior.
ckeditor4-react is a React integration for CKEditor 4, a popular WYSIWYG editor. It offers a wide range of features and plugins for content editing, similar to react-quill. CKEditor 4 provides a more traditional WYSIWYG editing experience and is highly configurable, making it a good alternative for users looking for a feature-rich editor.
See the live demo.
Warning: The project is still in alpha stage. Use with caution.
Use straight away:
var React = require('react');
var ReactQuill = require('react-quill');
var MyComponent = React.createClass({
/* ... */
render: function() {
return (
<ReactQuill value={this.state.value} />
);
}
});
Customize a few settings:
var MyComponent = React.createClass({
/* ... */
onTextChange: function(value) {
this.setState({ text:value });
},
render: function() {
return (
<ReactQuill theme="snow"
value={this.state.text}
onChange={this.onTextChange} />
);
}
});
Custom controls:
var MyComponent = React.createClass({
/* ... */
render: function() {
return (
<ReactQuill>
<ReactQuill.Toolbar key="toolbar"
ref="toolbar"
items={ReactQuill.Toolbar.defaultItems} />
<div key="editor"
ref="editor"
className="quill-contents"
dangerouslySetInnerHTML={{__html:this.getEditorContents()}} />
</ReactQuill>
);
}
});
Mixing in:
var MyComponent = React.createClass({
mixins: [ ReactQuill.Mixin ],
componentDidMount: function() {
var editor = this.createEditor(
this.getEditorElement(),
this.getEditorConfig()
);
this.setState({ editor:editor });
},
componentWillReceiveProps: function(nextProps) {
if ('value' in nextProps && nextProps.value !== this.props.value) {
this.setEditorContents(this.state.editor, nextProps.value);
}
},
/* ... */
});
See component.js for a fully fleshed-out example.
ReactQuill
accepts a few props:
id
: ID to be applied to the DOM element.
className
: Classes to be applied to the DOM element.
value
: Value for the editor as a controlled component.
defaultValue
: Initial value for the editor as an uncontrolled component.
readOnly
: If true, the editor won't allow changing its contents.
toolbar
: A list of toolbar items to use as custom configuration for the toolbar. Defaults (that also double as reference) are available as ReactQuill.Toolbar.defaultItems
and ReactQuill.Toolbar.defaultColors
. See also the Toolbar module over the Quill documentation for more information on the inner workings.
formats
: An array of formats to be enabled during editing. All implemented formats are enabled by default. See Formats for a list.
styles
: An object with custom CSS styles to be added to the editor. See configuration for details.
theme
: The name of the theme to apply to the editor. Defaults to base
.
pollInterval
: Interval in ms between checks for local changes in editor contents.
onChange(value)
: Called back with the new contents of the editor after change.
You can run the automated test suite:
$ npm test
And build a minificated version of the source:
$ npm run build
More tasks are available on the Makefile:
lint: lints the source
spec: runs the test specs
coverage: runs the code coverage test
test: lint, spec and coverage threshold test
build: builds the minified version
QuillToolbar
propType.QuillToolbar
QuillComponent
only accepting a single child.The MIT License (MIT)
Copyright (c) 2015, zenoamaro zenoamaro@gmail.com
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
v0.1.0
FAQs
The Quill rich-text editor as a React component.
The npm package react-quill receives a total of 312,740 weekly downloads. As such, react-quill popularity was classified as popular.
We found that react-quill demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.