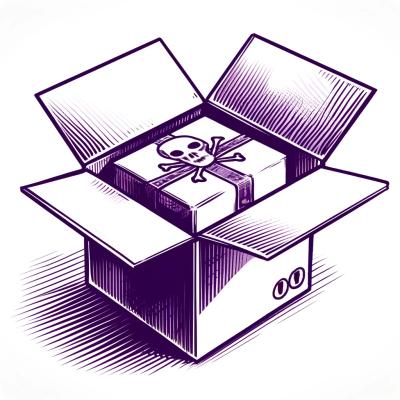
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
react-remote-resource
Advanced tools
Intuitive remote data management in React
react-remote-resource simplifies the integration of remote resources, usually api endpoints, into React applications, reducing boilerplate and data over-fetching.
Whenever a resource is used it will check the internal cache for a valid data entry. If a valid entry is found, the resource will return the data from the cache and the data is ready to use. If no valid entry is found, the load
function, which returns a Promise, will be invoked and thrown. The nearest RemoteResourceBoundary
, using Suspsense
under the hood, will catch the Promise and render the fallback
until all outstanding Promises resolve. If any of the Promises reject, the RemoteResourceBoundary
calls renderError
and onLoadError
(if provided) otherwise it returns the children
. This provides an intuitive way to use data from remote resources throughout your app without over-fetching, or the headache and boilerplate of Redux or some other data management library.
npm install react-remote-resource --save
// or
yarn add react-remote-resource
import {
createRemoteResource,
useResourceState,
RemoteResourceBoundary
} from "react-remote-resource";
const userResource = createRemoteResource({
load: userId => fetchJson(`/api/users/${userId}`),
invalidateAfter: 60 * 60 * 1000 // 1 hour
});
const tweetsResource = createRemoteResource({
load: userId => fetchJson(`/api/users/${userId}/tweets`),
invalidateAfter: 10000 // 10 seconds
});
const UserInfo = ({ userId }) => {
const [user] = useResourceState(userResource, [userId]);
const [tweets] = useResourceState(tweetsResource, [userId]);
return (
<div>
<img src={user.imageUrl} />
<h1>
{user.name} | Tweets: {tweets.length}
</h1>
<p>{user.bio}</p>
</div>
);
};
const Tweets = ({ userId }) => {
const [tweets] = useResourceState(tweetsResource, [userId]);
return (
<ul>
{tweets.map(tweet => (
<li key={tweet.id}>
<article>
<p>{tweet.message}</p>
<footer>
<small>{tweet.date}</small>
</footer>
</article>
</li>
))}
</ul>
);
};
const UserProfile = ({ userId }) => (
<RemoteResourceBoundary
fallback={<p>Loading...</p>}
renderError={error => <p>{error}</p>}
>
<UserInfo userId={userId} />
<Tweets userId={userId} />
</RemoteResourceBoundary>
);
createRemoteResource
A function that takes a config object and returns a resource.
const useProduct = createRemoteResource({
// Required: A Promise-returing function that resolves with the data or rejects if fails
load: id => fetch(`/api/products/${id}`).then(response => response.json()),
// Optional: A Promise-returing function that resolves with the data or rejects if fails
// Default: () => Promise.resolve()
save: product =>
? fetch(`/api/products/${product.id}`, { method: "PUT", body: JSON.stringify(product) }).then(response => response.json())
: fetch("/api/products", { method: "POST", body: JSON.stringify(product) }).then(response => response.json()),
// Optional: A Promise-returning function
// Default: () => Promise.resolve()
delete: product => fetch(`/api/products/${product.id}`, { method: "DELETE" }),
// Optional: The amount of time in milliseconds since the last update in which the cache is considered stale.
// Default: 300000 (5 minutes)
invalidateAfter: 10000,
// Optional: A function that creates an entry id from the arguments given to the hook
// Default: args => args.join("-") || "INDEX"
createEntryId: id => id.toString().toUpperCase(),
// Optional: The value to fall back to if no data has been fetched
initialValue: []
});
The return value from createRemoteResource
has the following shape:
{
id: string,
createEntryId: (...args: Array<any>) => string,
initialValue: any,
invalidateAfter: number,
load: (...args: Array<any>) => Promise<any>,
save: (...args: Array<any>) => Promise<any>,
delete: (...args: Array<any>) => Promise<any>,
getEntry: string => Immutable.RecordOf<{
id: string,
data: Maybe<any>,
updatedAt: Maybe<number>, // Unix timestamp
loadPromise: Maybe<Promise<any>>
}>,
onChange: (() => void) => void, // Allows for subscribing to state changes. Basically a wrapper around store.subscribe.
dispatch: ({ type: string }) => void // store.dispatch that adds `resourceId` to the action payload
};
useResourceState
A React hook that takes a resource and an optional array of arguments and returns a tuple, very much like React's useState
. The second item in the tuple works like useState
in that it sets the in-memory state of the resource. Unlike useState
, however, the state is not local to the component. Any other components that are using the state of that same resource get updated immediately with the new state! Under the hood react-remote-resource
implements a redux store. Every resource get its own state and there can be multiple entries for each resource, depending on the arguments you pass into useResourceState
and the optional createEntryId
option that you can pass into createRemoteResource
. By default every new combination of arguments will create a new entry in the store.
import { useResourceState } from "react-remote-resource";
const ProductCategory = ({ categoryId }) => {
const [categoriesById] = useResourceState(categoriesResource);
const [products] = useResourceState(productsResource, [categoryId]);
return (
<section>
<header>
<h1>{categoriesById[categoryId].name}</h1>
</header>
<ul>
{products.map(product => (
<li key={product.id}>
<Link to={`/products/${product.id}`}>{product.name}</Link>
</li>
))}
</ul>
</section>
);
};
This hook is very powerful. Let's walk through what happens when it is used:
load
function of the categoriesResource
and productsResource
will be invoked and promises thrown.RemoteResourceBoundary
will handle the error. If both promises resolve, the categoriesById
and products
data will be available to use (as the first item in the tuple).useState
, will persist in memory. If a component unmounts and remounts the state will be the same as when you left it.useResourceActions
A React hook that takes a resource and an optional array of arguments and returns an object literal with the following methods:
set
: A function that takes either the new state to set for this entry or a function that takes the current state of the entry and returns what should be set as the new state for the entry.
refresh
: A function that allows you to bypass the check against the updatedAt
timestamp and immediately refetch the data. Note: the promise will not be thrown for this action.
save
: The save
function that was defined with createRemoteResource
. Note: this will be undefined
if you did not define a save
function with createRemoteResource
.
delete
: The delete
function that was defined with createRemoteResource
. Note: this will be undefined
if you did not define a delete
function with createRemoteResource
.
Note: the array of arguments that are provided as the second argument will be spread as the initial arguments to the save
and delete
actions. For example, for the actions below, both save
and delete
inside of actions would receive categoryId
as the first parameter when invoked.
import { useResourceState, useResourceActions } from "react-remote-resource";
const ProductCategory = ({ categoryId }) => {
const [categoriesById] = useResourceState(categoriesState);
const [products] = useResourceState(productsResource, [categoryId]);
const actions = useResourceActions(productsResource, [categoryId]);
return (
<section>
<header>
<h1>{categoriesById[categoryId].name}</h1>
<button onClick={actions.refresh}>Refresh Products</button>
</header>
<ul>
{products.map(product => (
<li key={product.id}>
<Link to={`/products/${product.id}`}>{product.name}</Link>
</li>
))}
</ul>
</section>
);
};
RemoteResourceBoundary
Uses Suspense
under the hood to catch any thrown promises and render the fallback
while they are pending. Will also catch any errors that occur in the promise from a resource's load
function and renderError
and call onLoadError
if provided.
const UserProfile = ({ userId }) => (
<RemoteResourceBoundary
/* Optional: A React node that will show while any thrown promises are pending. `null` by default. */
fallback={<p>Loading...</p>}
/* Optional: A callback that is invoked when any thrown promise rejects */
onLoadError={error => {
logError(error);
}}
/* Required: A render prop that receives the error and a function to clear the error, which allows the children to re-render and attempt loading again */
renderError={(error, clearError) => (
<div>
<p>{error}</p>
<button onClick={clearError}>Try again</button>
</div>
)}
>
<UserInfo userId={userId} />
<Tweets userId={userId} />
</RemoteResourceBoundary>
);
useSuspense
A hook that takes a promise returning function. It will throw the returned promise as long as it is pending.
import { useSuspense, useResourceActions } from "react-remote-resource";
import userResource from "../resources/user";
const SaveButton = ({ onClick }) => (
<button onClick={useSuspense(onClick)}>Save</button>
);
const UserForm = () => {
const actions = useResourceActions(userResource);
return (
<div>
...Your form fields
<Suspense fallback={<p>Saving...</p>}>
<SaveButton onClick={actions.save} />
</Suspense>
</div>
);
};
FAQs
Intuitive remote data management in React
The npm package react-remote-resource receives a total of 7 weekly downloads. As such, react-remote-resource popularity was classified as not popular.
We found that react-remote-resource demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.