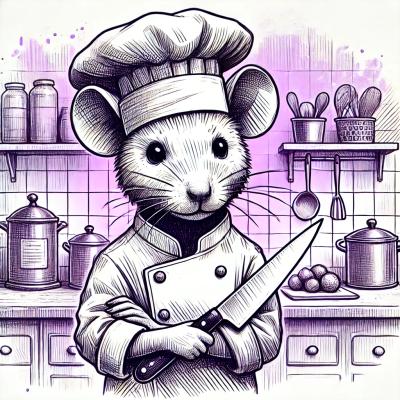
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
react-virtualized
Advanced tools
The react-virtualized npm package provides efficient rendering of large lists and tabular data by only rendering the items that are currently visible within the viewport. This can significantly improve the performance of applications that need to display large amounts of data.
List
The List component allows you to render large lists of items efficiently. It only renders the rows that are currently visible to the user, based on the scroll position.
{"import { List } from 'react-virtualized';
function MyList({ list }) {
return (
<List
width={300}
height={300}
rowCount={list.length}
rowHeight={20}
rowRenderer={({ index, key, style }) => (
<div key={key} style={style}>
{list[index]}
</div>
)}
/>
);
}"}
Table
The Table component is used for rendering large data sets in a tabular format. Similar to List, it only renders the rows that are visible in the viewport.
{"import { Column, Table } from 'react-virtualized';
function MyTable({ list }) {
return (
<Table
width={1000}
height={300}
headerHeight={20}
rowHeight={30}
rowCount={list.length}
rowGetter={({ index }) => list[index]}
>
<Column label='Name' dataKey='name' width={100} />
<Column label='Age' dataKey='age' width={200} />
</Table>
);
}"}
Grid
The Grid component can render a virtualized grid of cells, which is useful for displaying data in a spreadsheet-like format. It renders only the cells that are currently in the viewport.
{"import { Grid } from 'react-virtualized';
function MyGrid({ columnCount, rowCount }) {
return (
<Grid
columnCount={columnCount}
columnWidth={100}
height={300}
rowCount={rowCount}
rowHeight={30}
width={300}
cellRenderer={({ columnIndex, key, rowIndex, style }) => (
<div key={key} style={style}>
{`R${rowIndex}, C${columnIndex}`}
</div>
)}
/>
);
}"}
InfiniteLoader
The InfiniteLoader component works with List, Table, or Grid to load more items as the user scrolls. It's useful for implementing 'infinite scroll' features where more data is fetched as needed.
{"import { InfiniteLoader, List } from 'react-virtualized';
function MyInfiniteList({ list, loadMoreRows }) {
const isRowLoaded = ({ index }) => !!list[index];
return (
<InfiniteLoader
isRowLoaded={isRowLoaded}
loadMoreRows={loadMoreRows}
rowCount={list.length}
>
{({ onRowsRendered, registerChild }) => (
<List
ref={registerChild}
onRowsRendered={onRowsRendered}
width={300}
height={300}
rowCount={list.length}
rowHeight={20}
rowRenderer={({ index, key, style }) => (
<div key={key} style={style}>
{list[index]}
</div>
)}
/>
)}
</InfiniteLoader>
);
}"}
React-window is a complete rewrite of react-virtualized by the same author. It offers similar functionality but with a smaller and faster core. It's designed to be more approachable and easier to use than react-virtualized.
React-infinite is another package for rendering large lists of elements within a scrolling container. It differs from react-virtualized in its API and the way it handles infinite loading, but it also aims to provide efficient rendering for large lists.
React-virtuoso is a virtual list component with a simple API that supports variable-sized items and sticky headers. It provides a different approach to virtualization compared to react-virtualized, focusing on simplicity and automatic handling of item heights.
React-list is a versatile infinite scroll React component. It offers several modes for rendering lists, including simple, variable, and uniform heights. It's a simpler alternative to react-virtualized with fewer features but can be easier to integrate in some cases.
Demos available here: http://bvaughn.github.io/react-virtualized/
Install react-virtualized
using npm.
npm install react-virtualized --save
import React from 'react';
import ReactDOM from 'react-dom';
import { VirtualScroll } from 'react-virtualized';
// List data as an array of strings
const list = [
'Brian Vaughn'
// And so on...
];
// Render your list
ReactDOM.render(
<VirtualScroll
width={300}
height={300}
rowsCount={list.size}
rowHeight={20}
rowRenderer={index => list[index]}
/>,
document.getElementById('example')
);
import React from 'react';
import ReactDOM from 'react-dom';
import { FlexTable, FlexColumn } from 'react-virtualized';
// Table data as a array of objects
const list = [
{ name: 'Brian Vaughn', description: 'Software engineer'
// And so on...
];
// Render your table
ReactDOM.render(
<FlexTable
width={300}
height={300}
headerHeight={20}
rowHeight={30}
rowsCount={list.length}
rowGetter={index => list[index]}
>
<FlexColumn
label='Name'
dataKey='name'
width={100}
/>
<FlexColumn
width={200}
label='Description'
dataKey='description'
/>
</FlexTable>,
document.getElementById('example')
);
Use GitHub issues for requests.
I actively welcome pull requests; learn how to contribute.
Changes are tracked in the changelog.
react-virtualized is available under the MIT License.
FAQs
React components for efficiently rendering large, scrollable lists and tabular data
The npm package react-virtualized receives a total of 808,774 weekly downloads. As such, react-virtualized popularity was classified as popular.
We found that react-virtualized demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.