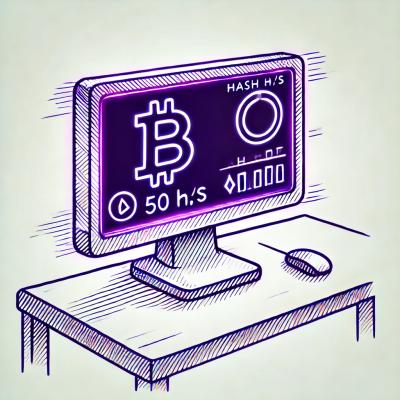
Security News
Research
Supply Chain Attack on Rspack npm Packages Injects Cryptojacking Malware
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
reactive-di
Advanced tools
Definitely complete solution for dependency injection, state-to-css, state-to-dom rendering, data loading, optimistic updates and rollbacks.
Dependency injection with flowtype support, based on ds300 derivablejs. For old browsers needs Map, Observable, Promise and optionally Reflect, Symbol polyfills.
No statics, no singletones, abstract everything, configure everything.
Features:
Compile with babel metadata plugin.
// @flow
import React from 'react'
import ReactDOM from 'react-dom'
import jss from 'jss'
import jssCamel from 'jss-camel-case'
import {Updater, UpdaterStatus, Di, ReactComponentFactory} from 'reactive-di/index'
import {hooks, theme, component, updaters, source} from 'reactive-di/annotations'
const userFixture = {
id: 1,
name: 'John Doe',
email: 'john@example.com'
}
// Fetcher service, could be injected from outside by key 'Fetcher' as is
@source({key: 'Fetcher', construct: false})
class Fetcher {
fetch<V>(url: string): Promise<V> {
// fake fetcher for example
return Promise.resolve((userFixture: any))
}
}
// Create separate updater qeue for user
class UserUpdater extends Updater {}
@source({key: 'User'})
class User {
static Updater: Class<Updater> = UserUpdater
id: number
name: string
email: string
constructor(rec: Object) {
this.id = rec.id
this.name = rec.name
this.email = rec.email
}
}
@hooks(User)
class UserHooks {
_updater: Updater
_fetcher: Fetcher
constructor(fetcher: Fetcher, updater: Updater) {
this._fetcher = fetcher
this._updater = updater
}
onMount(user: User): void {
this._updater.setSingle(
() => this._fetcher.fetch('/user'),
User
)
}
onUnmount(user: User): void {
this._updater.cancel()
}
}
class UserServiceUpdater extends Updater {}
class UserService {
static Updater: Class<Updater> = UserServiceUpdater
_updater: Updater
_fetcher: Fetcher
_user: User
constructor(
fetcher: Fetcher,
updater: Updater,
user: User
) {
this._fetcher = fetcher
this._updater = updater
this._user = user
}
submit: () => void = () => {
this._updater.set([
])
}
changeColor: () => void = () => {
this._updater.setSingle({color: 'green'}, ThemeVars)
}
}
@updaters(User.Updater, UserService.Updater)
class LoadingUpdaterStatus extends UpdaterStatus {}
@updaters(UserService.Updater)
class SavingUpdaterStatus extends UpdaterStatus {}
// Model ThemeVars, could be injected from outside by key 'ThemeVars' as ThemeVarsRec
@source({key: 'ThemeVars'})
class ThemeVars {
color: string
constructor(r?: Object = {}) {
this.color = r.color || 'red'
}
}
// Provide class names and data for jss in __css property
@theme
class UserComponentTheme {
wrapper: string
status: string
name: string
__css: mixed
constructor(vars: ThemeVars) {
this.__css = {
wrapper: {
backgroundColor: `rgb(${vars.color}, 0, 0)`
},
status: {
backgroundColor: 'red'
},
name: {
backgroundColor: 'green'
}
}
}
}
interface UserComponentProps {
children?: mixed;
}
interface UserComponentState {
theme: UserComponentTheme;
user: User;
loading: LoadingUpdaterStatus;
saving: SavingUpdaterStatus;
service: UserService;
}
/* jsx-pragma h */
function UserComponent(
{children}: UserComponentProps,
{theme, user, loading, saving, service}: UserComponentState,
h
): mixed {
if (loading.pending) {
return <div class={theme.wrapper}>Loading...</div>
}
if (loading.error) {
return <div class={theme.wrapper}>Loading error: {loading.error.message}</div>
}
return <div className={theme.wrapper}>
<span className={theme.name}>Name: {user.name}</span>
{children}
<button disabled={saving.pending} onClick={service.submit}>Save</button>
{saving.error
? <div>Saving error: {saving.error.message}, <a href="#" onClick={saving.retry}>Retry</a></div>
: null
}
</div>
}
component()(UserComponent)
jss.use(jssCamel)
const node: HTMLElement = window.document.getElementById('app')
const render = (widget: Function, attrs: ?Object) => ReactDOM.render(React.createElement(widget, attrs), node)
const di = (new Di(
new ReactComponentFactory(React),
(styles) => jss.createStyleSheet(styles)
))
.values({
Fetcher: new Fetcher()
})
render(di.wrapComponent(UserComponent))
More docs and examples coming soon.
FAQs
Reactive dependency injection
The npm package reactive-di receives a total of 14 weekly downloads. As such, reactive-di popularity was classified as not popular.
We found that reactive-di demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.
Security News
Sonar’s acquisition of Tidelift highlights a growing industry shift toward sustainable open source funding, addressing maintainer burnout and critical software dependencies.