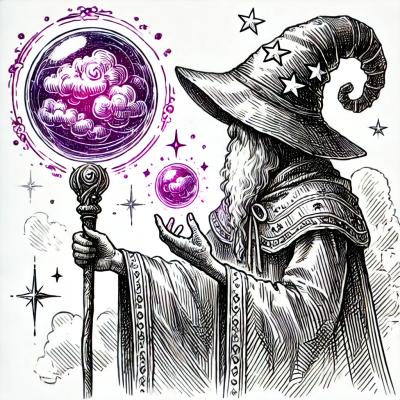
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
The npm package 'redis' is a Node.js client for Redis, a fast, open-source, in-memory key-value data store for use as a database, cache, message broker, and queue. The package allows Node.js applications to interact with Redis servers using an asynchronous, event-driven model.
Connecting to Redis
This code sample demonstrates how to connect to a Redis server using the redis npm package. It requires the package, creates a client, and listens for the 'connect' event to confirm the connection.
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis');
});
Setting and Getting Data
This code sample shows how to set a key-value pair in Redis and then retrieve the value associated with a key. The 'redis.print' callback is used to output the result of the 'set' operation.
client.set('key', 'value', redis.print);
client.get('key', function(err, reply) {
console.log(reply); // prints 'value'
});
Working with Lists
This code sample illustrates how to work with Redis lists by pushing values to the end of a list and then retrieving the entire list.
client.rpush(['list', 'value1', 'value2'], redis.print);
client.lrange('list', 0, -1, function(err, reply) {
console.log(reply); // prints ['value1', 'value2']
});
Publish/Subscribe
This code sample demonstrates the publish/subscribe capabilities of Redis. It creates a subscriber client that listens for messages on a channel and a publisher client that publishes a message to that channel.
const subscriber = redis.createClient();
const publisher = redis.createClient();
subscriber.on('message', function(channel, message) {
console.log('Message: ' + message + ' on channel: ' + channel);
});
subscriber.subscribe('notification');
publisher.publish('notification', 'Hello, World!');
Transactions
This code sample shows how to use Redis transactions to execute multiple commands atomically using the 'multi' and 'exec' methods.
client.multi()
.set('key', 'value')
.incr('counter')
.exec(function(err, replies) {
console.log(replies); // prints results of all commands
});
ioredis is a robust, performance-focused, and full-featured Redis client for Node.js. It supports Redis Cluster, Sentinel, pipelining, Lua scripting, and more. Compared to the 'redis' package, ioredis offers a more modern interface with Promises support and better performance for certain operations.
node-redis is another Redis client for Node.js that is designed to be easy to use. It may not have as many features as 'redis' or 'ioredis', but it provides a straightforward way to interact with Redis servers for simple use cases.
redis-mock is a library that simulates a Redis server for testing purposes. It implements most of the Redis commands and can be used as a drop-in replacement for the 'redis' package during testing, without the need for an actual Redis server.
:warning: Version 4 is still under development and isn't ready for production use. Use at your own risk.
npm install redis@next
(async () => {
const client = require('redis').createClient();
client.on('error', (err) => console.log('Redis Client Error', err));
await client.connect();
await client.set('key', 'value');
const value = await client.get('key');
})();
There is built-in support for all of the out-of-the-box Redis commands. They are exposed using the raw Redis command names (HGET
, HSET
, etc.) and a friendlier camel-cased version (hGet
, hSet
, etc.).
// raw Redis commands
await client.SET('key', 'value');
await client.GET('key');
// friendly JavaScript commands
await client.hSet('key', 'field', 'value');
await client.hGetAll('key');
Modifiers to commands are specified using a JavaScript object:
await client.set('key', 'value', {
EX: 10,
NX: true
});
Replies will be transformed to useful data structures:
await client.hGetAll('key'); // { key1: 'value1', key2: 'value2' }
await client.hKeys('key'); // ['key1', 'key2']
If you want to run commands and arguments that Node Redis doesn't know about (yet!) you can use .sendCommand
:
await client.sendCommand(['SET', 'key', 'value', 'NX']); // 'OK'
await client.sendCommand(['HGETALL', 'key']); // ['key1', 'field1', 'key2', 'field2']
Any command can be run on a new connection by specifying the duplicateConnection
option. The newly created connection is closed when command's Promise
is fulfilled.
This pattern works especially well for blocking commands—such as BLPOP
and BRPOPLPUSH
:
const blPopPromise = client.blPop(
client.commandOptions({ duplicateConnection: true }),
'key'
);
await client.lPush('key', ['1', '2']);
await blPopPromise; // '2'
Subscribing to a channel requires a dedicated Redis connection and is easily handled using events.
await subscriber.subscribe('channel', message => {
console.log(message); // 'message'
});
await subscriber.pSubscribe('channe*', (message, channel) => {
console.log(message, channel); // 'message', 'channel'
});
await publisher.publish('channel', 'message');
You can define Lua scripts to create efficient custom commands:
(async () => {
const client = require('redis').createClient({
scripts: {
add: require('redis/lua-script').defineScript({
NUMBER_OF_KEYS: 1,
SCRIPT:
'local val = redis.pcall("GET", KEYS[1]);' +
'return val + ARGV[1];',
transformArguments(key: string, toAdd: number): Array<string> {
return [key, number.toString()];
},
transformReply(reply: number): number {
return reply;
}
})
}
});
await client.connect();
await client.set('key', '1');
await client.add('key', 2); // 3
})();
If you'd like to contribute, check out the contributing guide.
This repository is licensed under the "MIT" license. See LICENSE.
FAQs
A modern, high performance Redis client
The npm package redis receives a total of 3,326,731 weekly downloads. As such, redis popularity was classified as popular.
We found that redis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.