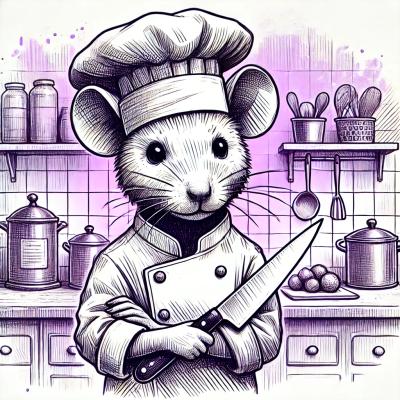
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
redux-awaiter
Advanced tools
A Redux middleware for giving opportunities to await and receive actions in anywhere
Redux Awaiter is a Redux middleware for giving opportunities to await and receive actions in anywhere.
Redux Awaiter is designed to await and receive Redux actions in React components and help us manage local state conveniently.
It's inspired by Redux Saga, but the problems they solve are totally different.
class UserListView extends React.PureComponent {
state = { loading: false };
async componentDidMount() {
const { actions: { fetchUserList } } = this.props;
fetchUserList();
this.setState(state => ({ ...state, loading: true })); // start loading
await take('RECEIVE_USER_LIST'); // reducers will update `users` in redux store
this.setState(state => ({ ...state, loading: false })); // receive data, stop loading
}
render() {
const { users } = this.props; // `users` is mapped from redux store
const { loading } = this.state;
return (
<Spin loading={loading}>
<ul>
{users.map(({ id, name }) => <li key={id}>{name}</li>)}
</ul>
</Spin>
);
}
}
npm i redux-awaiter -D
Redux Awaiter is written in TypeScript.
The internal type definition is based on flux standard action.
type ActionType = string;
interface BaseAction {
type: ActionType;
}
interface Action<P, M = {}> extends BaseAction {
payload: P;
meta?: M;
error?: true;
}
A pattern is to determine whether an action is matching with another.
type Pattern<P = {}, M = {}> = string | RegExp | ((action: Action<P, M>) => boolean);
import { createStore, applyMiddleware } from 'redux';
import { createAwaiterMiddleware } from 'redux-awaiter';
const awaiterMiddleware = createAwaiterMiddleware();
const store = createStore(rootReducer, applyMiddleware(
// other middlewares (e.g. routerMiddleware)
awaiterMiddleware,
));
const take: <P = {}, M = {}>(pattern: Pattern<P, M>) => Promise<Action<P, M>>;
take
receives a pattern as its single argument, and returns a Promise which contains the first matching action.
const action = await take('RECEIVE_DATA'); // action.type should be RECEIVE_DATA
const takeAllOf: <P = {}, M = {}>(patterns: Pattern<P, M>[]) => Promise<Action<P, M>[]>;
takeAllOf
receives an array of patterns as its single argument, and returns a Promise which contains an array of actions correspond to patterns.
Internally, takeAllOf(patterns)
is the same with Promise.all(patterns.map(take))
.
If you need to await and receive multiple actions in specific order, use multiple await take()
instead.
const [{ payload: articles }, { payload: users }] = await takeAllOf(['RECEIVE_ARTICLES', 'RECEIVE_USERS']);
const takeOneOf: <P = {}, M = {}>(patterns: Pattern<P, M>[]) => Promise<Action<P, M>>;
takeOneOf
receives an array of patterns as its single argument, and returns a Promise which contains the first action matched with one of patterns.
Internally, takeOneOf(patterns)
is the same with Promise.race(patterns.map(take))
.
const { type } = await takeOneOf(['FETCH_USER_SUCCESS', 'FETCH_USER_FAILURE']);
if (type === 'FETCH_USER_SUCCESS') {
// success
} else {
// failure
}
You might not need takeOneOf
:
const { type } = await take(/^FETCH_USER/);
Avoid relying on props MUTATION!
This may cause unexpected behaviors, or make your components difficult to maintain.
async componentDidMount() {
const { data } = this.props // this.props.data is mapped from redux store.
// dispatch an action and do some async call (e.g. xhr, fetch)
await take('RECEIVE_DATA'); // receive new data and update redux store by reducer
// DANGER: this.props.data is MUTATED!
console.assert(this.props.data === data); // Assertion failed!
}
FAQs
A Redux middleware for giving opportunities to await and receive actions in anywhere
The npm package redux-awaiter receives a total of 0 weekly downloads. As such, redux-awaiter popularity was classified as not popular.
We found that redux-awaiter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.