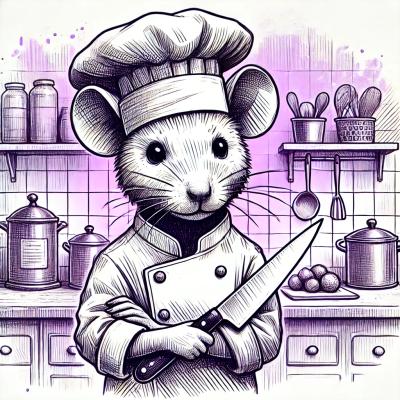
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
redux-jest-helper
Advanced tools
Provides easily testable classes that generate redux-middlewares or -reducers
Provides an easy way to create and test Redux Reducers and Middlewares.
Using yarn you only need to execute
yarn add redux-jest-helper
or if you are using npm, execute
npm install redux-jest-helper --save
Implement a MiddlewareFactory<S>
. The abstract class provides an interface for 3 functions that
can be implemented:
onBeforeAction?: (api: MiddlewareAPI<S>, action: Action) => void
onAfterAction?: (api: MiddlewareAPI<S>, action: Action, prevState: S) => void
onCreateMiddleware?: (api: MiddlewareAPI<S>) => void
import { MiddlewareFactory } from 'redux-jest-helper'
class CounterMiddlewareFactory extends MiddlewareFactory<CounterState> {
onBeforeAction = (api: MiddlewareAPI<CounterState>, action: Action) => {
switch (action.type) {
case 'INCREMENT':
this.onIncrement(action as IncrementAction) // do something before an INCREMENT action
break
}
}
onAfterAction = (api: MiddlewareAPI<CounterState>, action: Action, prevState: CounterState) => {
switch (action.type) {
case 'DECREMENT':
this.afterDecrement(action as DecrementAction) // do something after a DECREMENT action
break
}
}
}
The following example will test, if the given methods are called on the given actions.
import { middlewareTestHelper } from 'redux-jest-helper'
middlewareTestHelper(new CounterMiddlewareFactory(), [
{ actionType: 'INCREMENT', methods: ['onIncrement'] },
{ actionType: 'DECREMENT', methods: ['afterDecrement'] }
])
Now that you tested if the methods are really called when the according action happens you only need to unit-test the methods themselves.
Implement a ReducerFactory<S>
. The abstract class provides an interface for
onAction: (state: S | undefined, action: Action) => S
.
class CounterReducerFactory extends ReducerFactory<CounterState> {
onIncrement = (state: CounterState, action: IncrementAction) => {
return {
...state,
count: state.count + action.amount
}
}
onDecrement = (state: CounterState, action: DecrementAction) => {
return {
...state,
count: state.count - action.amount
}
}
onAction = (state: CounterState = initialState, action: Action) => {
let newState = state
switch (action.type) {
case 'DECREMENT':
newState = this.onDecrement(state, action as DecrementAction)
break
case 'INCREMENT':
newState = this.onIncrement(state, action as IncrementAction)
break
}
return newState
}
}
The following example will test, if the given methods are called on the given actions with the given payload.
import { reducerTestHelper } from 'redux-jest-helper'
describe('reducerTestHelper', () => {
reducerTestHelper(new TestReducerFactory(), initialState, [
{ actionType: 'INCREMENT', actionValue: { amount: 1 }, methods: 'onIncrement' },
{ actionType: 'DECREMENT', actionValue: { amount: 1 }, methods: 'onDecrement' }
])
})
Now that you tested if the methods are really called when the according action happens you only need to unit-test the methods themselves.
const counterReducerFactory = new CounterReducerFactory()
const counterMiddlewareFactory = new CounterMiddlewareFactory()
const rootReducer = combineReducers({
counter: counterReducerFactory.toReducer()
})
createStore(rootReducer, applyMiddleware(counterMiddlewareFactory.toMiddleware()))
FAQs
Provides easily testable classes that generate redux-middlewares or -reducers
We found that redux-jest-helper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.