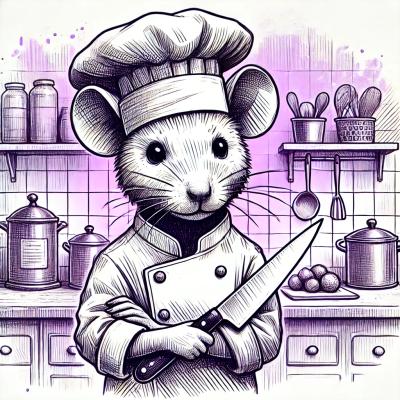
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
replace-css-names
Advanced tools
Replace all CSS class name's and id's based on given matches array.
Read data from the provided key "data". Replace all class names and ids with provided replacement. Each current output are written to the output path.
Extract class and id names from css file
const ReplaceCssNames = require('replace-css-names');
const fs = require("fs");
gulp.task('replace:css', async (done) => {
/**
* Target file to write
*/
const output = `${__dirname}/replaced.css`;
/**
* Remove old file
*/
if(fs.existsSync(output))
{
fs.unlinkSync(output);
}
/**
* Get matches from file
*/
const extractedFromCss = `${__dirname}/extracted-names-for-css`;
if (!fs.existsSync(extractedFromCss))
{
throw new Error(`The required file cannot be found: ${extractedFromCss}`);
}
let extractedMatchesCss = [];
/**
* From string back to array
*/
try {
extractedMatchesCss = fs.readFileSync(extractedFromCss, 'UTF-8');
extractedMatchesCss = JSON.parse(extractedMatchesCss);
}
catch (e) {
throw new Error(e);
}
const files = [
`public/css/packages.css`,
`public/css/packages.less.css`,
`public/css/app.css`,
];
const replace = (c = 0) =>
{
return new Promise( async (resolve, reject) =>
{
const cssString = fs.readFileSync(files[c], 'UTF-8');
await new ReplaceCssNames(
{
path: files[c],
output,
logger: {
logging: true,
prefix: 'Replace',
displayFilename: true,
displayPercentage: true,
type: 'bar',
barBg: 'bgCyan'
},
type: 'css',
matches: extractedMatchesCss,
data: cssString,
restModulo: 10000,
restTime: 200,
displayResult: false,
ignore: [],
}
);
c++;
if (undefined !== files[c])
{
await replace(c);
return resolve(true);
}
else
{
resolve(true);
}
})
}
await replace();
done();
});
gulp.task('obfuscate:css',
gulp.series(
[
'replace:css'
]
)
);
const ReplaceCssNames = require('replace-css-names');
const fs = require("fs");
/**
* Target path
*/
const output = `${__dirname}/replaced.css`;
/**
* Remove old file
*/
if(fs.existsSync(output))
{
fs.unlinkSync(output);
}
/**
* Get matches
*/
const matchesFile = `${__dirname}/extracted-names-for-css`;
if(!fs.existsSync(matchesFile))
{
throw new Error(`The required file cannot be found: ${matchesFile}`);
}
let matches = [];
try{
matches = fs.readFileSync(matchesFile, 'UTF-8');
matches = JSON.parse(matches);
}
catch(e)
{
throw new Error(e);
}
const files = [
`public/css/packages.css`,
`public/css/packages.less.css`,
`public/css/app.css`,
];
const replace = async (c = 0) =>
{
const cssString = fs.readFileSync(files[c], 'UTF-8');
await new ReplaceCssNames(
{
path: files[c],
output,
logger: {
logging: true,
prefix: 'Replace',
displayFilename: true,
displayPercentage: true,
type: 'bar',
barBg: 'bgCyan'
},
type: 'css',
matches,
data: cssString,
restModulo: 10000,
restTime: 200,
displayResult: false,
ignore: [],
}
);
c++;
if(undefined !== files[c])
{
await replace(c);
}
}
replace();
gulp.task('replace:react', async (done) =>
{
/**
* Target path
*/
const output = `${__dirname}/public/_App.js`;
/**
* Remove old replace file
*/
if (fs.existsSync(output))
{
fs.unlinkSync(output);
}
/**
* Get matches
*/
const extractedFromJs = `${__dirname}/extracted-names-for-js`;
if (!fs.existsSync(extractedFromJs))
{
throw new Error(`The required file cannot be found: ${extractedFromJs}`);
}
let extractedMatchesJs = [];
try {
extractedMatchesJs = fs.readFileSync(extractedFromJs, 'UTF-8');
extractedMatchesJs = JSON.parse(extractedMatchesJs);
}
catch (e) {
throw new Error(e);
}
const files = [
`${__dirname}/build/_App.js`,
];
const replace = (c = 0) =>
{
return new Promise( async (resolve, reject) =>
{
const fileContent = fs.readFileSync(files[c], 'UTF-8');
await new ReplaceCssNames(
{
path: files[c],
output,
logger: {
logging: true,
prefix: 'Replace',
displayFilename: true,
displayPercentage: true,
type: 'arc',
barBg: 'bgCyan',
},
type: 'react',
matches: extractedMatchesJs,
data: fileContent,
restModulo: 10000,
restTime: 200,
displayResult: false,
ignore: [],
attributes: ['defaultClass'],
forceReplace: []
}
);
c++;
if (undefined !== files[c])
{
await replace(c);
return resolve(true);
}
else
{
resolve(true);
}
})
}
await replace();
done();
});
gulp.task('obfuscate:css',
gulp.series(
[
'replace:react'
]
)
);
.flex{display:flex}.flex-row{flex-direction:row}.flex-column{flex-direction:column}.w-100{width:100%}.d-none{display:none}
.k195{display:flex}.z147{flex-direction:row}.f107{flex-direction:column}.o190{width:100%}.s183{display:none}
,{className:"".concat(n," ").concat(d," ").concat(t," ").concat(this.state.mounted?"":"td0"),style:f}),x().createElement("div",i()({className:"area-header"},S(o)&&O({},o)),r),!this.state.isMinified&&x().createElement("div",i()({className:"area-sidebar"},S(c)&&O({},c)),this.state.sidebarData),x().createElement("div",i()({className:"area-content
{className:"".concat(n," ").concat(d," ").concat(t," ").concat(this.state.mounted?"":"td0"),style:f}),x().createElement("div",i()({className:"v107"},S(o)&&O({},o)),r),!this.state.isMinified&&x().createElement("div",i()({className:"d96"},S(c)&&O({},c)),this.state.sidebarData),x().createElement("div",i()({className:"e96 ".
Only Modules there are generated from the base path (not node_modules) are replaced. Modules imported from node_modules are not replaced.
Option | type | Description |
---|---|---|
path | string | Path of the current file |
output | string | Path to store/write current replaced CSS file content |
data | string | Css files content |
type | string | Type of files content. Available types: 'css', 'react' |
matches | array | Array of objects: [ { find: string; replace: string; type: string;}, ... ] |
attributes | array | Array of strings/atrribute names to replace. Default [ 'className', 'id' ]. Only available if type is 'react' |
forceReplace | array | Array of strings. If sensitive match, does not replace a class or id, you can force it ( the forced name has to be availbale in the matches array) |
restModulo | number | Each number of lines should be made a break |
restTime | number | Duration of the break in ms |
displayResult | boolean | Display the replace result in the terminal |
ignore | array | Ignore names to replace |
logger | object | Logger options |
Option | type | Description |
---|---|---|
logging | boolean | Display current process |
prefix | string | Prefix on the logging line |
displayFilename | boolean | Display current processed filename (filename extracted from path) |
displayPercentage | boolean | Display current percentage value |
type | string | Process animation type. Available types: 'spinner', 'bar', 'dots', 'dots2', 'arc', 'line' |
barBg | string | If the animation type is bar, then set the bar's background-color. Background colors are based on the chalk module |
FAQs
Replace all CSS class name's and id's based on given matches array.
We found that replace-css-names demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.