Satpam
Satpam is a wrapper for some nodejs validator libraries, I made Satpam
so it's easy to create
custom validator with parameters and custom validation messages.

Quick Usage
var satpam = require('satpam');
var rules = {
name : ['required']
officeEmail : ['email'],
phone : ['required', 'numeric']
};
var input = {
name: 'Sendy',
title: 'Lord',
officeEmail: 'invalid email',
phone: 'hi there123'
};
var result = satpam.validate(rules, input);
if (result.success === true) {
} else {
result.messages.officeEmail.email === 'OfficeEmail must be email';
result.messages.phone.number === 'Phone must be numeric';
result.messages.messageArray[0] = 'OfficeEmail must be email';
result.messages.messageArray[1] = 'Phone must be numeric';
}
Satpam instance
Satpam has create
method to create new validator instance.
- Each instance will have cloned validation rules and messages, so it's safe to add or override validation rule without affecting other validator instances or the global satpam validator.
- The cloned validation rules and messages will be based on the current state of the global satpam validator. See Custom Rules
var satpam = require('satpam');
var validatorOne = satpam.create();
var validatorTwo = satpam.create();
Available Rules
-
required
-
numeric
-
email
-
image
-
alpha
-
alphanumeric
-
date
-
url
-
string
-
nonBlank
-
maxLength:<length>
-
minLength:<length>
-
maxValue:<max value>
-
minValue:<min value>
-
memberOf:$1
Use object notation for defining this rule
examples
-
beginWith:$1
Use object notation for defining this rule
examples
-
regex:$1:$2
$1
is the pattern, $2
is the regex flags
examples
Custom rules
Add custom rules globally, it will affect every Validator
instance(s) that
is created after the custom rules addition, but not the old instance(s).
var satpam = require('satpam');
var oldValidator = satpam.create();
satpam.addCustomValidation('must-be-ironman', function(val) {
return val === 'ironman';
});
satpam.setValidationMessage('must-be-ironman', 'Not ironman D:');
satpam.addCustomValidation('range:$1:$2', function(val, ruleObj) {
return val >= ruleObj.params[0] && val <= ruleObj.params[1];
});
satpam.setValidationMessage('range:$1:$2', '<%= propertyName %> must between <%= ruleParams[0] %> and <%= ruleParams[1] %>');
var newValidator = satpam.create();
TODOs
- Better documentation.
- Add more validation rules.
- Validate file types.
More examples
Here
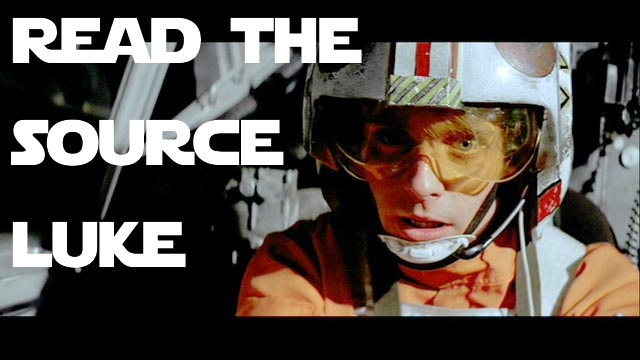