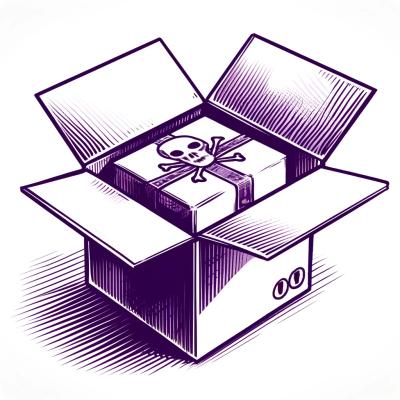
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
simple-boot-front
Advanced tools
Single Page Application Framworks
The reference documentation includes detailed installation instructions as well as a comprehensive getting started guide.
npm install simple-boot-front
Here is a quick teaser of a complete simple-boot-front application in typescript
index.ts
const option = new SimOption(AppRouter)
.setUrlType(UrlType.path)
.setAdvice(Advice);
const simpleApplication = new SimpleApplication(option);
simpleApplication.run();
index.html
<!doctype html>
<html>
<head>...</head>
<body id="app"></body>
</html>
AppRouter.ts
@Sim({scheme: 'layout-router'})
export class AppRouter extends RootRouter {
module = App;
'' = Index;
'ajax' = Ajax;
'intent' = Intents;
'view' = Views;
'exception' = Exception;
'aop' = Aop;
'views' = Views;
async canActivate(url: Url, module: Module): Promise<Module | ConstructorType<Module>> {
if (url.path === 'views' && url.params.get('auth') === 'false') {
return Forbidden;
} else {
return module;
}
}
notFound(url: Url): ConstructorType<Module> {
console.log(url.path)
return Notfound;
}
}
index.ts
@Sim({scheme: 'index'})
export class Index extends Module {
template = html;
data = "default data";
thisData = 5151;
constructor(public v: ViewService, public manager: SimstanceManager, public navigation: Navigation) {
super("index");
}
onInit() {
}
@PostConstruct
public post(renderer: Renderer) {
}
}
index.html
<h1>index</h1>
links:
<ul>
<li>ajax example page: <a router-link="">ajax</a></li>
</ul>
<div class="card mt-2" style="width: 100%;">
<div class="card-body">
<h5 class="card-title">set module</h5>
<div>set variable: {%write(this.title)%}</div>
<div>input module variable: <input type="text" module-event-keyup="changeText"></div>
</div>
</div>
<div class="card mt-2" style="width: 100%;">
<div class="card-body">
<h5>event click change "module-event-click"</h5>
{%write(this.numbers)%}
<button class="btn btn-info" module-event-click="changeData">change</button>
</div>
</div>
<div class="card mt-2" style="width: 100%;">
<div class="card-body">
<h5>default data set "module-value"</h5>
<input type="text" module-value="data">
</div>
</div>
<div class="card mt-2" style="width: 100%;">
<div class="card-body">
<h5>variable isolate "module-isolate"</h5>
<div module-isolate="data">
{%write(this.data)%}
</div>
data link change event <input type="text" module-link="data">
</div>
</div>
<div>
{%write(this.thisData)%}
thisData <button module-event-click="thisDataChange"> this data change</button>
</div>
<div>
<div module-event-click="goto">aaaaaaaaa</div>
</div>
<div>{%write(this.data)%}</div>
<div>
{%
for (let i of this.datas) {
write('<li>' + i + '</li>');
}
%}
</div>
@Sim({scheme: 'index'})
export class Index extends Module { }
@PostConstruct
post(projectService: ProjectService) {
console.log('post Construct and dependency injection')
}
fire($event: MouseEvent, view: View<Element>) {
console.log('fire method')
this.data = RandomUtils.random(0, 100);
}
@Before({property: 'fire'})
before(obj: any, protoType: Function) {
console.log('before', obj, protoType)
}
@After({property: 'fire'})
after(obj: any, protoType: Function) {
console.log('after', obj, protoType)
}
@ExceptionHandler()
public exception0(e: any) {
console.log('Advice Global exception:', e)
}
click() {
this.publish(new Intent('layout://info/data?a=wow&aa=zzz', Math.floor(RandomUtils.random(0, 100))));
// this.publish(new Intent('layout://info/datas', Math.floor(RandomUtils.random(0, 100))));
// this.publish(new Intent('layout://', Math.floor(RandomUtils.random(0, 100)))); // default callback method -> subscribe(i: Intent)
// this.publish(new Intent('://info/datas', Math.floor(RandomUtils.random(0, 100))));
}
@Sim({scheme: 'layout'})
export class App extends Module {
styleImports = [css];
template = html;
info = new AppInfo();
constructor() {
super("app-layout-module");
}
}
export class AppInfo extends Module {
template = '<div><h3>info</h3>{%write(this.datas)%}</div>';
datas = 'default data';
data(i: Intent) {
this.datas = i.data + '->' + i.params.aa
}
}
<button module-event-click="changeData">change</button>
<input module-event-change="changeData">
<input module-event-keyup="changeData">
<input module-event-keydown="changeData">
<input module-event-link="value">
<input module-event-value="value">
<div module-isolate="data">{%write(this.data)%}</div>
<a router-link="ajax" router-active-class="['active']">Ajax</a>
<a router-link="ajax">Ajax</a>
click event intent publish:
<button class="btn btn-primary" module-event-click-intent-publish="['layout://info/data?a=wow&aa=ppp','makeRandom']">publish data</button>
typing:
<input type="text" module-event-keyup-intent-publish="['layout://info/viewSubscribe?a=wow&aa=vvv']">
data(i: Intent) {
this.datas = i.data + '->' + i.params.aa
}
viewSubscribe(i: Intent<View<HTMLInputElement>>) {
this.datas = i.data?.value + '->' + i.params.aa + '-->' + i.event
}
/*[module-selector]*/ h1 {color: burlywood;}
{{module-selector}} h1 { color: burlywood;}
FAQs
front end SPA frameworks
The npm package simple-boot-front receives a total of 9 weekly downloads. As such, simple-boot-front popularity was classified as not popular.
We found that simple-boot-front demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.