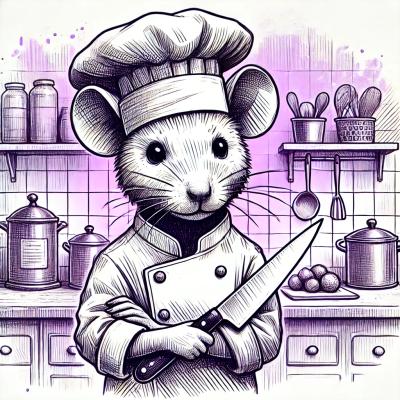
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
split-on-first
Advanced tools
The split-on-first npm package is a simple utility that allows you to split a string on the first occurrence of a specified separator. This is particularly useful when you need to parse strings where only the first separator is significant and subsequent separators are part of the remaining substring.
Splitting a string on the first occurrence of a separator
This function splits the string 'a,b,c,d' on the first comma. The result is an array with two elements: the substring before the first comma ('a') and the substring after the first comma ('b,c,d').
"a,b,c,d".splitOnFirst(',')
The 'split' package offers functionality to split strings based on a delimiter, but it splits on every occurrence of the delimiter, not just the first. This makes 'split-on-first' more suitable for cases where only the first delimiter is significant.
This is the native JavaScript split method available on string instances. It also splits on every occurrence of the specified separator. Unlike 'split-on-first', it does not limit the split to the first occurrence, which can lead to different use cases.
Split a string on the first occurrence of a given separator
This is similar to String#split()
, but that one splits on all the occurrences, not just the first one.
$ npm install split-on-first
import splitOnFirst from 'split-on-first';
splitOnFirst('a-b-c', '-');
//=> ['a', 'b-c']
splitOnFirst('key:value:value2', ':');
//=> ['key', 'value:value2']
splitOnFirst('a---b---c', '---');
//=> ['a', 'b---c']
splitOnFirst('a-b-c', '+');
//=> []
splitOnFirst('abc', '');
//=> []
Type: string
The string to split.
Type: string
The separator to split on.
FAQs
Split a string on the first occurance of a given separator
The npm package split-on-first receives a total of 3,192,729 weekly downloads. As such, split-on-first popularity was classified as popular.
We found that split-on-first demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.