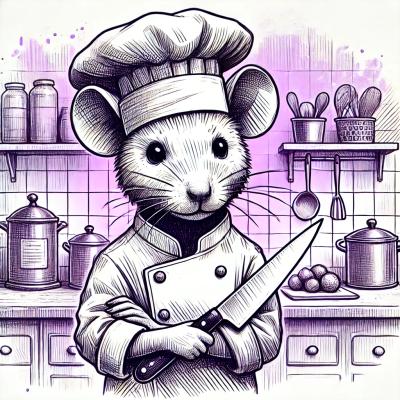
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Run remote commands over a pool of server using SSH.
npm install ssh-pool
var sshPool = require('ssh-pool');
var pool = new sshPool.ConnectionPool(['user@server1', 'user@server2']);
pool.run('hostname')
.then(function (results) {
console.log(results[0].stdout); // 'server1'
console.log(results[1].stdout); // 'server2'
});
Create a new connection to run command on a remote server.
Arguments:
@param {object} options Options
@param {string|object} options.remote Remote
@param {Stream} [options.stdout] Stdout stream
@param {Stream} [options.stderr] Stderr stream
@param {string} [options.key] SSH key
@param {function} [options.log] Log method
The remote can use the shorthand syntax or an object:
// Default user will be deploy and ssh default port.
new Connection({remote: 'localhost'});
// Default ssh port will be used.
new Connection({remote: 'user@localhost'});
// Custom user and custom port.
new Connection({remote: 'user@localhost:22'});
// Object syntax.
new Connection({remote: {user: 'user', host: 'localhost', port: 22}});
The log method is used to log output directly:
var connection = new Connection({
remote: 'localhost',
log: console.log.bind(console)
});
connection.run('pwd');
// Will output:
// Running "pwd" on host "localhost".
// @localhost /my/directory
Run a command on the remote server, you can specify custom childProcess.exec
options. A callback or a promise can be used.
Arguments:
@param {string} command Command
@param {object} [options] Exec options
@param {function} [cb] Callback
@returns {Promise}
connection.run('ls', {env: {NODE_ENV: 'test'}})
.then(function (result) {
result.stdout; // stdout output
result.stderr; // stderr output
result.child; // child object
});
Copy a file or a directory to a remote server, you can specify custom childProcess.exec
options. A callback or a promise can be used.
Arguments:
@param {string} src Source
@param {string} dest Destination
@param {object} [options] Exec Options
@param {function} [cb] Callback
@returns {Promise}
connection.copy('./localfile', '/remote-file', {env: {NODE_ENV: 'test'}})
.then(function (result) {
result.stdout; // stdout output
result.stderr; // stderr output
result.child; // child object
});
Create a new pool of connections and custom options for all connections.
If you use the short syntax, connections will be automatically created, else you can use previous created connections.
// Use shorthand.
var pool = new ConnectionPool(['server1', 'server2']);
// Use previously created connections.
var connection1 = new Connection({remote: 'server1'});
var connection2 = new Connection({remote: 'server2'});
var pool = new ConnectionPool([connection1, connection2]);
Same as connection.run
, except that the command is executed in parallel on each server of the pool.
Arguments:
@param {string} command Command
@param {object} [options] Options
@param {function} [cb] Callback
@returns {Promise}
pool.run('hostname')
.then(function (results) {
// ...
});
Same as connection.copy
, except that the copy is done in parallel on each server of the pool.
Options:
@param {object} [options.direction] Direction of copy
Also all exec options are supported.
Arguments:
@param {string} src Source
@param {string} dest Destination
@param {object} options Options
@param {function} [cb] Callback
@returns {Promise}
pool.copy('./localfile', '/remote-file')
.then(function (results) {
// ...
});
MIT
FAQs
Run remote commands over a pool of server using SSH.
The npm package ssh-pool receives a total of 2,824 weekly downloads. As such, ssh-pool popularity was classified as popular.
We found that ssh-pool demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.