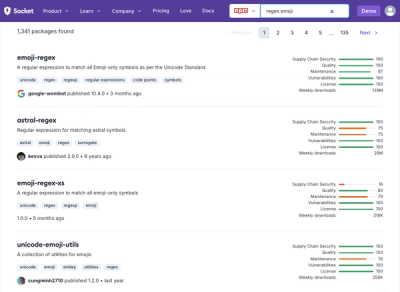
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
A node.js wrapper for Valve's Steam Web API. Also supports the methods provided for TF2/TF2Beta/Portal.
Use of the API requires an API key, obtainable here.
This implementation is not supported, endorsed, or created by Valve - I'm just a fan. This is just a wrapper - all of Valve's terms and conditions for using their API still apply, see the Steam community developer page for additional information.
$ npm install steam-web
All methods accept a single options object. The key names match the query string parameters specified in the valve documentation. See usage and the valve documentation for any additional params.
All methods can be passed a .apiVersion
property that overrides the default api version in the url. Some methods (such as getSchema) will support different games on different versions (TF2 is only supported on v0001, CSGO is only supported on v0002). You can use this property to change the version for the api request if you are not getting back the expected response.
If using JSON for results (default), the result will automatically be parsed into a json object before being passed to the callback. Any other formats will return the raw data (xml or vdf).
var steam = require('steam-web');
var s = new steam({
apiKey: 'XXXXXXXXXXXXXXXX',
format: 'json' //optional ['json', 'xml', 'vdf']
});
s.getNewsForApp({
appid: 440,
count: 3,
maxlength: 300,
callback: function(err, data) {
console.log(data);
}
})
s.getGlobalAchievementPercentagesForApp({
gameid: 440,
callback: function(err, data) {
console.log(data);
}
});
s.getPlayerSummaries({
steamids: ['76561198037414410', '76561197960435530'],
callback: function(err, data) {
console.log(data);
}
})
s.getFriendList({
steamid: '76561197960435530',
relationship: 'all', //'all' or 'friend'
callback: function(err,data) {
console.log(data);
},
})
s.getSchema({
gameid: 440,
callback: function(err, data) {
console.log(data);
}
})
s.getPlayerItems({
gameid: 440,
steamid: '76561197960435530',
callback: function(err, data) {
console.log(data);
}
})
s.getAssetPrices({
appid: 440, //can also use gameid instead for convenience
callback: function(err,data) {
console.log(data);
}
})
s.getPlayerAchievements({
gameid: 440,
steamid: '76561197960435530',
l: 'en',
callback: function(err,data) {
console.log(data);
}
})
s.getRecentlyPlayedGames({
steamid: '76561197960435530',
callback: function(err,data) {
console.log(data)
}
})
s.getOwnedGames({
steamid: '76561197960435530',
callback: function(err,data) {
console.log(data)
}
})
s.getUserStatsForGame({
steamid: '76561197963506690',
appid: 730,
callback: function(err,data) {
console.log(data);
}
})
s.getGlobalStatsForGame({
appid: 17740,
name: ['global.map.emp_isle'], // can also pass a single string
count: 1, // or you can let the module work it out for you
callback: function(err,data) {
console.log(data);
}
})
s.isPlayingSharedGame({
steamid: '76561198120639625',
appid_playing: 730,
callback: function(err,data) {
console.log(data);
}
})
s.getSchemaForGame({
appid: 730,
callback: function(err,data) {
console.log(data);
}
})
s.getPlayerBans({
steamids: ['76561198120639625'], // can also pass a single string
callback: function(err,data) {
console.log(data);
}
})
s.getAppList({
callback: function(err, data) {
console.log(data);
}
})
s.getServersAtAddress({
addr: '193.192.58.116',
callback: function(err, data) {
console.log(data);
}
})
s.upToDateCheck({
version: 100,
appid: 440,
callback: function(err, data) {
console.log(data);
}
})
s.getUserGroupList({
steamid: '76561197960435530',
callback: function(err, data) {
console.log(data);
}
})
s.resolveVanityURL({
vanityurl: 'vincegogh',
callback: function(err, data) {
console.log(data);
}
})
s.getNumberOfCurrentPlayers({
appid: 440,
callback: function(err, data) {
console.log(data);
}
})
s.getSteamLevel({
steamid: '76561197960435530',
callback: function(err, data) {
console.log(data);
}
})
s.getBadges({
steamid: '76561197960435530',
callback: function(err, data) {
console.log(data);
}
})
s.getCommunityBadgeProgress({
steamid: '76561197960435530',
badgeid: 2,
callback: function(err, data) {
console.log(data);
}
})
s.getServerInfo({
callback: function(err, data) {
console.log(data);
}
})
s.getSupportedAPIList({
callback: function(err, data) {
console.log(data);
}
})
s.getSchemaURL({
appid: 440,
callback: function(err, data) {
console.log(data);
}
})
s.getStoreMetadata({
appid: 440,
callback: function(err, data) {
console.log(data);
}
})
s.getStoreStatus({
appid: 440,
callback: function(err, data) {
console.log(data);
}
})
There are two ways to use getAssetClassInfo. By default, the Steam API wants a query string formatted as: ?classid0=1234&classid1=5678&class_count=2
As such, you can either manually generate the keys and call the method like this:
s.getAssetClassInfo({
appid: 440, //can also use gameid instead for convenience
classid0: '16891096',
classid1: 151,
class_count: 2,
callback: function(err,data) {
console.log(data);
}
})
OR, we have provided a convenience property so you can just pass an array of ids (when using the convenience property, you don't need to pass class_count either)
s.getAssetClassInfo({
appid: 440, //can also use gameid instead for convenience
classIds: ['16891096',151],
callback: function(err,data) {
console.log(data);
}
})
$ npm test
$ npm run format
$ npm run lint
$ npm run docs
$ npm run coverage
$ npm run integrate
FAQs
A wrapper for the Steam Web API.
The npm package steam-web receives a total of 4,960 weekly downloads. As such, steam-web popularity was classified as popular.
We found that steam-web demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.