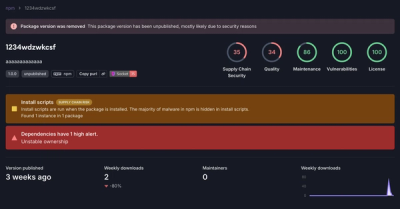
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The steno npm package is a simple, fast, and lightweight library for writing JSON files atomically. It ensures that data is written to disk safely and without corruption, making it ideal for applications that need to frequently update JSON files.
Atomic Write
This feature allows you to write JSON data to a file atomically. The `writeFile` method ensures that the data is written safely, preventing file corruption.
const steno = require('steno');
const fs = require('fs');
const data = { key: 'value' };
steno.writeFile('data.json', JSON.stringify(data), (err) => {
if (err) throw err;
console.log('Data written successfully');
});
Synchronous Write
This feature allows you to write JSON data to a file synchronously. The `writeFileSync` method ensures that the data is written safely and the operation is completed before moving on to the next line of code.
const steno = require('steno');
const fs = require('fs');
const data = { key: 'value' };
try {
steno.writeFileSync('data.json', JSON.stringify(data));
console.log('Data written successfully');
} catch (err) {
console.error('Error writing data:', err);
}
The write-file-atomic package provides similar functionality to steno by ensuring that files are written atomically. It is designed to handle the same use cases, such as preventing file corruption during write operations. However, write-file-atomic offers more configuration options and is more widely used in the community.
The fs-extra package extends the native Node.js fs module with additional methods, including atomic write operations. While it offers a broader range of file system utilities compared to steno, it is also heavier and includes more features than just atomic writes.
Simple file writer with race condition prevention and atomic writing.
Built on graceful-fs and used in lowdb.
Let's say you have a server and want to save data to disk:
var data = { counter: 0 };
server.post('/', function (req, res) {
++data.counter;
fs.writeFile('data.json', JSON.stringify(obj), function (err) {
if (err) throw err;
res.end();
});
})
Now if you have many requests, for example 1000
, there's a risk that you end up with:
// In your server
data.counter === 1000;
// In data.json
data.counter === 865; // ... or any other value
Why? Because, fs.write
doesn't guarantee that the call order will be kept. Also, if the server is killed while data.json
is being written, the file can get corrupted.
server.post('/increment', function (req, res) {
++obj.counter
steno.writeFile('data.json', JSON.stringify(obj), function (err) {
if (err) throw err;
res.end();
})
})
With steno you'll always have the same data in your server and file. And in case of a crash, file integrity will be preserved.
Important: works only in a single instance of Node.
MIT - Typicode
FAQs
Specialized fast async file writer
The npm package steno receives a total of 128,580 weekly downloads. As such, steno popularity was classified as popular.
We found that steno demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.