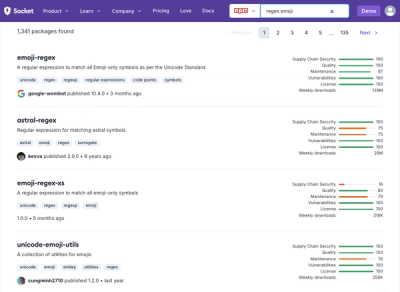
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
storyblok-js-client
Advanced tools
The storyblok-js-client is a JavaScript client for interacting with the Storyblok API. It allows developers to easily manage content, perform CRUD operations, and interact with the Storyblok content delivery and management APIs.
Initialize the Client
This feature allows you to initialize the Storyblok client with your access token, which is required to authenticate and interact with the Storyblok API.
const StoryblokClient = require('storyblok-js-client');
const client = new StoryblokClient({
accessToken: 'your-access-token'
});
Get a Story
This feature allows you to fetch a specific story from the Storyblok API. In this example, it fetches the 'home' story.
client.get('cdn/stories/home', {}).then(response => {
console.log(response.data.story);
}).catch(error => {
console.error(error);
});
Create a Story
This feature allows you to create a new story in your Storyblok space. The example demonstrates creating a story with a name, slug, and content.
client.post('spaces/your-space-id/stories', {
story: {
name: 'New Story',
slug: 'new-story',
content: {
component: 'page',
body: []
}
}
}).then(response => {
console.log(response.data.story);
}).catch(error => {
console.error(error);
});
Update a Story
This feature allows you to update an existing story in your Storyblok space. The example shows how to update the name, slug, and content of a story.
client.put('spaces/your-space-id/stories/your-story-id', {
story: {
name: 'Updated Story',
slug: 'updated-story',
content: {
component: 'page',
body: []
}
}
}).then(response => {
console.log(response.data.story);
}).catch(error => {
console.error(error);
});
Delete a Story
This feature allows you to delete a story from your Storyblok space. The example demonstrates how to delete a story by its ID.
client.delete('spaces/your-space-id/stories/your-story-id').then(response => {
console.log('Story deleted');
}).catch(error => {
console.error(error);
});
Contentful is a content management platform that allows you to create, manage, and distribute content to any platform. It offers a JavaScript client similar to storyblok-js-client for interacting with its API. Contentful provides a more extensive set of features and integrations, but Storyblok is known for its visual editor and ease of use.
Prismic is a headless CMS that offers a JavaScript client for interacting with its API. It provides similar functionalities to storyblok-js-client, such as fetching and managing content. Prismic is known for its flexible content modeling and integration capabilities.
Strapi is an open-source headless CMS that provides a JavaScript SDK for interacting with its API. It offers similar functionalities to storyblok-js-client, including content management and CRUD operations. Strapi is highly customizable and can be self-hosted, providing more control over the CMS environment.
This client is a thin wrapper for the Storyblok API's to use in Node.js and the browser.
npm install storyblok-js-client
Storyblok
Parameters
config
Object
accessToken
String, The preview token you can find in your space dashboard at https://app.storyblok.comcache
Object
type
String, none
or memory
endpoint
optional)Example
// 1. Require the Storyblok client
const StoryblokClient = require('storyblok-js-client');
// 2. Initialize the client with the preview token
// from your space dashboard at https://app.storyblok.com
let Storyblok = new StoryblokClient({
accessToken: 'xf5dRMMjltLzKOcNgMaU9Att'
});
The Storyblok client comes with a caching mechanism.
When initializing the Storyblok client you can define a cache provider for caching the requests in memory.
To clear the cache you can call Storyblok.flushCache();
.
let Storyblok = new StoryblokClient({
privateToken: 'xf5dRMMjltLzKOcNgMaU9Att',
cache: {
type: 'memory'
}
});
Storyblok#get
Parameters
[return]
Promise, Object response
path
String, Path (can be cdn/stories
, cdn/stories/*
, cdn/tags
, cdn/datasources
, cdn/links
)options
Object, Options can be found in the API documentation.Example
Storyblok
.get('cdn/stories/home', {
version: 'draft'
})
.then((response) => {
console.log(response);
})
.catch((error) => {
console.log(error);
});
Storyblok#flushCache
Parameters
[return]
Object, returns the Storyblok clientExample
Storyblok.flushCache();
Fork me on Github
FAQs
Universal JavaScript SDK for Storyblok's API
We found that storyblok-js-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.