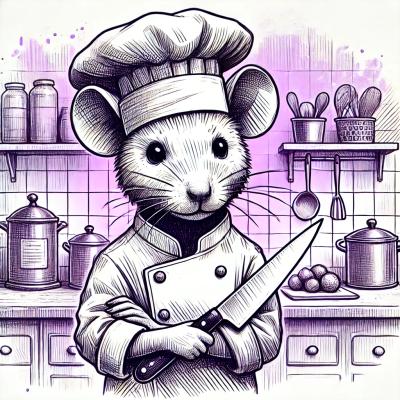
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
string-natural-compare
Advanced tools
Compare alphanumeric strings the same way a human would, using a natural order algorithm
The string-natural-compare npm package provides a way to compare strings in a human-friendly manner, often referred to as 'natural order' comparison. This is particularly useful for sorting lists of strings that include numbers, where you want the numbers to be sorted in numerical order rather than lexicographical order.
Basic Natural Comparison
This feature allows you to compare two strings in a natural order. In this example, 'file10' is compared to 'file2', and the result is 1, indicating that 'file10' comes after 'file2' in natural order.
const naturalCompare = require('string-natural-compare');
const result = naturalCompare('file10', 'file2');
console.log(result); // Output: 1
Sorting an Array
This feature allows you to sort an array of strings in natural order. In this example, the array ['file10', 'file2', 'file1'] is sorted to ['file1', 'file2', 'file10'].
const naturalCompare = require('string-natural-compare');
const files = ['file10', 'file2', 'file1'];
files.sort(naturalCompare);
console.log(files); // Output: ['file1', 'file2', 'file10']
Case-Insensitive Comparison
This feature allows you to perform a case-insensitive natural comparison. In this example, 'File10' is compared to 'file2' with case insensitivity, and the result is 1, indicating that 'File10' comes after 'file2' in natural order.
const naturalCompare = require('string-natural-compare');
const result = naturalCompare('File10', 'file2', { caseInsensitive: true });
console.log(result); // Output: 1
The natural-compare-lite package provides similar functionality for natural order string comparison but is designed to be a lighter and faster alternative. It lacks some of the additional options available in string-natural-compare, such as case insensitivity.
The alphanum-sort package offers alphanumeric sorting of arrays, which is similar to natural order comparison. It is optimized for performance and can handle large arrays efficiently. However, it does not provide as many customization options as string-natural-compare.
Compare alphanumeric strings the same way a human would, using a natural order algorithm
Important: The API has changed between v1 and v2. If upgrading, check out the migration guide. If using the Bower module (or v1 npm module), see the v1 API.
Standard sorting: Natural order sorting:
img1.png img1.png
img10.png img2.png
img12.png img10.png
img2.png img12.png
This module provides two functions:
naturalCompare
naturalCompare.caseInsensitive
(alias: naturalCompare.i
)These functions return a number indicating whether one string should come before, after, or is the same as another string.
They can be easily used with the native .sort()
array method.
This module uses a performant and robust algorithm to compare alphanumeric strings. It can compare strings containing any size of number and is heavily tested with a custom benchmark suite to make sure that it is as fast as possible, even when a custom alphabet has been configured.
npm install string-natural-compare --save
bower install string-natural-compare
Include the script in your HTML (drop the ".min" to use the development version):
<script src="/bower_components/string-natural-compare/natural-compare.min.js"></script>
Note: IE8 and lower not supported.
var naturalCompare = require('string-natural-compare');
// Simple case-sensitive sorting
var a = ['z1.doc', 'z10.doc', 'z17.doc', 'z2.doc', 'z23.doc', 'z3.doc'];
a.sort(naturalCompare);
// -> ['z1.doc', 'z2.doc', 'z3.doc', 'z10.doc', 'z17.doc', 'z23.doc']
// Simple case-insensitive sorting
var a = ['B', 'C', 'a', 'd'];
a.sort(naturalCompare.caseInsensitive); // alias: a.sort(naturalCompare.i);
// -> ['a', 'B', 'C', 'd']
// Note:
['a', 'A'].sort(naturalCompare.caseInsensitive); // -> ['a', 'A']
['A', 'a'].sort(naturalCompare.caseInsensitive); // -> ['A', 'a']
// Compare strings containing large numbers
naturalCompare(
'1165874568735487968325787328996865',
'265812277985321589735871687040841'
);
// -> 1 (a number > 0)
// In most cases we want to sort an array of objects
var a = [
{street: '350 5th Ave', room: 'A-1021'},
{street: '350 5th Ave', room: 'A-21046-b'}
];
// Sort by street (case-insensitive), then by room (case-sensitive)
a.sort(function(a, b) {
return (
naturalCompare.caseInsensitive(a.street, b.street) ||
naturalCompare(a.room, b.room)
);
});
// When text transformation is needed or when doing a case-insensitive sort on a
// large array, it is best for performance to pre-compute the transformed text
// and store it in that object. This way, the text transformation will not be
// needed for every comparison when sorting.
var a = [
{make: 'Audi', model: 'R8'},
{make: 'Porsche', model: '911 Turbo S'}
];
// Sort by make, then by model (both case-insensitive)
a.forEach(function(car) {
car.sortKey = (car.make + ' ' + car.model).toLowerCase();
});
a.sort(function(a, b) {
return naturalCompare(a.sortKey, b.sortKey);
});
It is possible to configure a custom alphabet to achieve a desired character ordering.
// Estonian alphabet
naturalCompare.alphabet = 'ABDEFGHIJKLMNOPRSŠZŽTUVÕÄÖÜXYabdefghijklmnoprsšzžtuvõäöüxy';
['t', 'z', 'x', 'õ'].sort(naturalCompare);
// -> ['z', 't', 'õ', 'x']
// Russian alphabet
naturalCompare.alphabet = 'АБВГДЕЁЖЗИЙКЛМНОПРСТУФХЦЧШЩЪЫЬЭЮЯабвгдеёжзийклмнопрстуфхцчшщъыьэюя';
['Ё', 'А', 'б', 'Б'].sort(naturalCompare);
// -> ['А', 'Б', 'Ё', 'б']
Note: Putting numbers in the custom alphabet can cause undefined behaviour.
The module must now be required like so:
var naturalCompare = require('string-natural-compare');
Then the following replacements need to be made:
String.naturalCompare
→ naturalCompare
String.naturalCaseCompare
→ naturalCompare.caseInsensitive
(or the alias naturalCompare.i
)String.alphabet
→ naturalCompare.alphabet
FAQs
Compare alphanumeric strings the same way a human would, using a natural order algorithm
The npm package string-natural-compare receives a total of 3,492,131 weekly downloads. As such, string-natural-compare popularity was classified as popular.
We found that string-natural-compare demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.