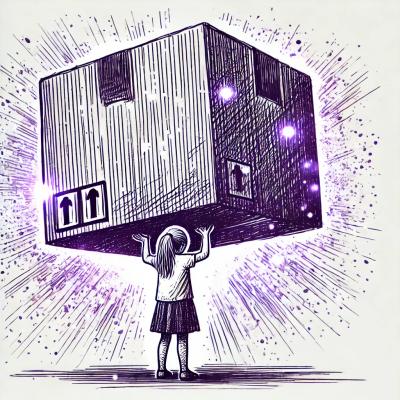
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
The 'subarg' npm package allows you to parse command-line arguments with nested sub-arguments. This is particularly useful for complex command-line interfaces where arguments can have their own sub-arguments.
Basic Parsing
This feature allows you to parse command-line arguments into a structured object. The `process.argv.slice(2)` part skips the first two default arguments (node and script path).
const subarg = require('subarg');
const argv = subarg(process.argv.slice(2));
console.log(argv);
Nested Arguments
This feature allows you to parse nested sub-arguments. For example, `--foo [--bar baz]` will be parsed into an object where `foo` is an array containing an object with `bar` set to `baz`.
const subarg = require('subarg');
const argv = subarg(process.argv.slice(2));
console.log(argv);
// Example: node script.js --foo [--bar baz]
Default Values
This feature allows you to set default values for arguments. If an argument is not provided, it will take the default value specified.
const subarg = require('subarg');
const argv = subarg(process.argv.slice(2), { default: { foo: 'default' } });
console.log(argv);
// Example: node script.js
Minimist is a lightweight package for parsing command-line arguments. It is simpler than subarg and does not support nested sub-arguments. It is useful for straightforward argument parsing.
Yargs is a more feature-rich package for parsing command-line arguments. It supports nested arguments, command handling, and more. It is more complex than subarg but offers a broader range of functionalities.
Commander is another powerful package for building command-line interfaces. It supports nested commands and options, making it comparable to subarg in terms of handling complex argument structures.
parse arguments with recursive contexts using minimist
This module is useful if you need to pass arguments into a piece of code without coordinating ahead of time with the main program, like with a plugin system.
var subarg = require('subarg');
var argv = subarg(process.argv.slice(2));
console.log(argv);
Contexts are denoted with square brackets:
$ node example/show.js rawr --beep [ boop -a 3 ] -n4 --robots [ -x 8 -y 6 ]
{ _: [ 'rawr' ],
beep: { _: [ 'boop' ], a: 3 },
n: 4,
robots: { _: [], x: 8, y: 6 } }
var subarg = require('subarg')
Parse the arguments array args
, passing opts
to
minimist.
An opening [
in the args
array creates a new context and a ]
closes a
context. Contexts may be nested.
With npm do:
npm install subarg
MIT
FAQs
parse arguments with recursive contexts
The npm package subarg receives a total of 1,312,091 weekly downloads. As such, subarg popularity was classified as popular.
We found that subarg demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.