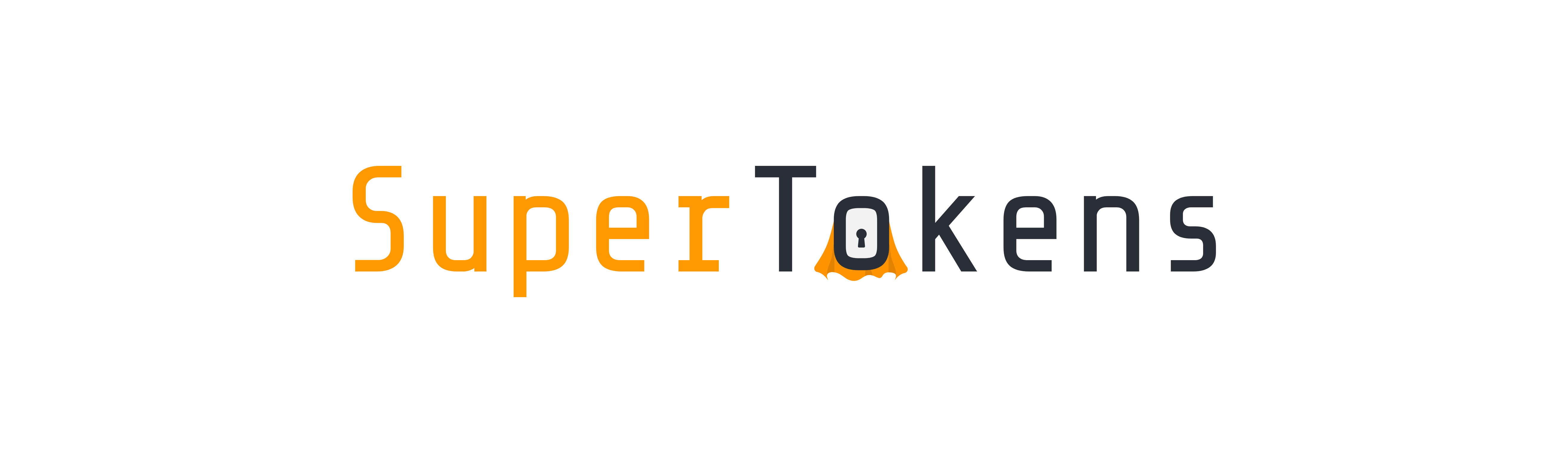

This library implements the frontend part of user session management for websites. You can use this to make http(s) API calls to your backend that require an authenticated user.
Features:
- When you make an API call, and if the access token has expired, this library will automatically take care of refreshing the token for you. After successfully refreshing it, it will call your API with the new token again and return its response.
- Takes care of race conditions mentioned in the footer of this blog post.
Installation
To get started, you just need to do:
npm i --save supertokens-website
Usage
This library is to be used instead of fetch in places where the API requires authentication.
import * as SuperTokensRequest from "supertokens-website";
SuperTokensRequest.init(refreshTokenURL, sessionExpiredStatusCode)
- To be called at least once before any http request is made from your frontend that uses this library. For example, if your website is a single page ReactJS app, then you can call this in the constructor of the root component.
SuperTokensRequest.init("/api/refreshtoken", 440)
SuperTokensRequest.get(url, config)
- send a GET request to this url - to be used only with your app's APIs
SuperTokensRequest.get("/someAPI", config).then(response => {
}).catch(err => {
});
SuperTokensRequest.post(url, config)
- send a POST request to this url - to be used only with your app's APIs
SuperTokensRequest.post("/someAPI", config).then(response => {
}).catch(err => {
});
SuperTokensRequest.delete(url, config)
- send a DELETE request to this url - to be used only with your app's APIs
SuperTokensRequest.delete("/someAPI", config).then(response => {
}).catch(err => {
});
SuperTokensRequest.put(url, config)
- send a PUT request to this url - to be used only with your app's APIs
SuperTokensRequest.put("/someAPI", config).then(response => {
}).catch(err => {
});
SuperTokensRequest.doRequest(func)
- use this function to send a request using any other http method that is not mentioned above
SuperTokensRequest.doRequest(() => fetch(...)).then(response => {
}).catch(err => {
});
SuperTokensRequest.attemptRefreshingSession()
- use this function when you want to manually refresh the session.
SuperTokensRequest.attemptRefreshingSession().then(success => {
if (success) {
} else {
}
}).catch(err => {
});
Example code & Demo
You can play around with the demo project that uses this and the supertokens-node-mysql-ref-jwt library. The demo demonstrates how this package behaves when it detects auth token theft (and the best part - you are the hacker here, muahahaha!)
Making changes
This library is written in TypeScript (TS). When you make any changes to the .ts files in the root folder, run the following command to compile to .js:
tsc -p tsconfig.json
Support, questions and bugs
For now, we are most reachable via team@supertokens.io, via the GitHub issues feature and our Discord server.
Authors
Created with :heart: by the folks at SuperTokens. We are a startup passionate about security and solving software challenges in a way that's helpful for everyone! Please feel free to give us feedback at team@supertokens.io, until our website is ready :grinning: