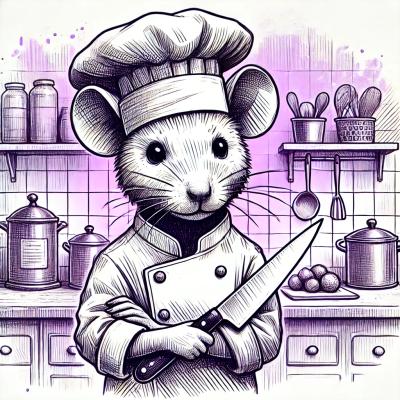
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
svelte-jsoneditor
Advanced tools
A web-based tool to view, edit, format, transform, and validate JSON
The library is written with Svelte, but can be used in any framework (React, Angular, plain JavaScript).
npm install
See the /examples section for some full examples.
Create a JSONEditor with two-way binding bind:json
:
<script>
import { JSONEditor } from 'svelte-jsoneditor'
let json = {
'array': [1, 2, 3],
'boolean': true,
'color': '#82b92c',
'null': null,
'number': 123,
'object': {'a': 'b', 'c': 'd'},
'string': 'Hello World'
}
</script>
<div>
<JSONEditor bind:json />
</div>
Or one-way binding:
<script>
import { JSONEditor } from 'svelte-jsoneditor'
let json = {
text: 'Hello World'
}
function onChange(content) {
console.log('onChange: ', content)
json = content.json
}
</script>
<div>
<JSONEditor
json={json}
onChange={onChange}
/>
</div>
Load as ES module:
<!DOCTYPE html>
<html lang="en">
<head>
<title>JSONEditor</title>
</head>
<body>
<div id="jsoneditor"></div>
<script type="module">
import { JSONEditor } from 'svelte-jsoneditor/dist/jsoneditor.mjs'
const editor = new JSONEditor({
target: document.getElementById('jsoneditor'),
props: {
json: {
text: 'Hello World'
},
onChange: (content) => {
console.log('onChange', content)
}
}
})
</script>
</body>
</html>
Or using UMD (exposed as window.jsoneditor.JSONEditor
):
<!DOCTYPE html>
<html lang="en">
<head>
<title>JSONEditor</title>
<script src="svelte-jsoneditor/dist/jsoneditor.js"></script>
</head>
<body>
<div id="jsoneditor"></div>
<script>
const editor = new JSONEditor({
target: document.getElementById('jsoneditor'),
props: {
json: {
text: 'Hello World'
},
onChange: (content) => {
console.log('onChange', content)
}
}
})
</script>
</body>
</html>
Svelte component:
<script>
import { JSONEditor } from 'svelte-jsoneditor'
</script>
<div>
<JSONEditor json={json} />
</div>
JavasScript class:
import { JSONEditor } from 'svelte-jsoneditor'
const editor = new JSONEditor({
target: document.getElementById('jsoneditor'),
props: {
json,
onChange: (content) => {
console.log('onChange', content)
}
}
})
json
Pass the JSON document to be rendered in the JSONEditormode: 'edit' | 'view'
Open the editor in editable mode ('edit'
) or
readonly ('view'
). Default is 'edit'
mainMenuBar: boolean
Show the main menu bar. Default value is true
.validator: function (json): ValidationError[]
. Validate the JSON document.
For example use the built-in JSON Schema validator powered by Ajv:
import { createAjvValidator } from 'svelte-jsoneditor'
const validator = createAjvValidator(schema, schemaRefs)
onChange({ json: JSON | undefined, text: string | undefined})
.
Callback which is invoked on every change made in the JSON document.onClassName(path: Array.<string|number>, value: any): string | undefined
.
Add a custom class name to specific nodes, based on their path and/or value.onFocus()
callback fired when the editor got focus.onBlur()
callback fired when the editor lost focus.get(): { json?: JSON, text?: string }
Get the current JSON documentset({ json?: JSON, text?: string })
Replace the current JSON document. Will reset the state of the editor.update({ json?: JSON, text?: string })
Update the loaded JSON document, keeping the state of the editor (like expanded objects).patch(operations: JSONPatchDocument)
Apply a JSON patch document to update the contents of the JSON document.scrollTo(path: Array.<string|number>)
Scroll the editor vertically such that the specified path comes into view. The path will be expanded when needed.destroy()
. Destroy the editor, remove it from the DOM.Clone the git repository
Install dependencies:
npm install
Start watcher:
npm start
Build the library:
npm run build
Run unit tests:
npm test
Run linter:
npm run lint
Released under the ISC license.
FAQs
A web-based tool to view, edit, format, transform, and validate JSON
The npm package svelte-jsoneditor receives a total of 2,234 weekly downloads. As such, svelte-jsoneditor popularity was classified as popular.
We found that svelte-jsoneditor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.