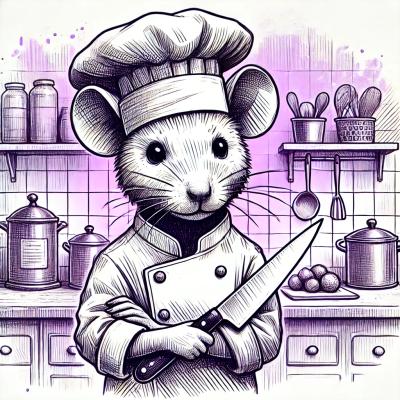
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
npm install @enterdao/landworks-widget --save
import { ListModal, RentModal } from '@enterdao/landworks-widget'
function App() {
const [wallet, setWallet] = useState('');
const [openRent, setOpenRent] = useState(false);
const [openList, setOpenList] = useState(false);
const provider = window.ethereum
? window.ethereum
: window.web3.currentProvider;
const connect = () => {
provider
? provider
.request({ method: 'eth_requestAccounts' })
.then((wallet: string[]) => setWallet(wallet[0]))
: alert('No wallet founds');
};
return (
<div >
<header >
{wallet.length ? (
<>
<RentModal
open={openRent}
onClose={() => setOpenRent(false)}
account={wallet}
assetId={'1'}
referrer={'1'}
provider={provider}
/>
<ListModal
open={openList}
onClose={() => setOpenList(false)}
account={wallet}
tokenId={'2'}
tokenAddress={'0x0000000000000000000000000000000000000000'}
referrer={'1'}
provider={provider}
/>
<b>Address : {wallet}</b>
<div style={{ display: 'flex', gap: '20px' }}>
<button onClick={() => setOpenRent(true)}>
Show rent modal
</button>
<button onClick={() => setOpenList(true)}>
Show list modal
</button>
</div>
</>
) : (
<button onClick={connect}>
Connect wallet
</button>
)}
</header>
</div>
);
}
export default App;
Prop | Description |
---|---|
open: boolean | Boolean, triggering the opening of the modal. |
onClose() | Callback function, called upon modal closing. |
onError(error) | Callback function, called upon error. |
onSuccess(txReceipt) | Callback function, called after contract execution has been mined. |
provider | MetaMask Web3 provider. |
account: string | EVM wallet address, connected to provider . |
referrer: string | EVM wallet address, passed as referrer upon listing and renting. |
Prop | Description |
---|---|
assetId: string | The LandWorks AssetId for which the rent modal will open. |
Prop | Description |
---|---|
tokenAddress: string | Address of the ERC-721 collection |
tokenId: string | Token ID from the ERC-721 collection |
FAQs
## Be able to list and rent from everywhere!
The npm package test-sth receives a total of 0 weekly downloads. As such, test-sth popularity was classified as not popular.
We found that test-sth demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.