three-pathfinding
Navigation mesh toolkit for ThreeJS, based on PatrolJS. Computes paths between points on a 3D nav mesh, supports multiple zones, and clamps movement vectors for FPS controls. To learn how to create a navigation mesh using Blender, see Creating a Nav Mesh.
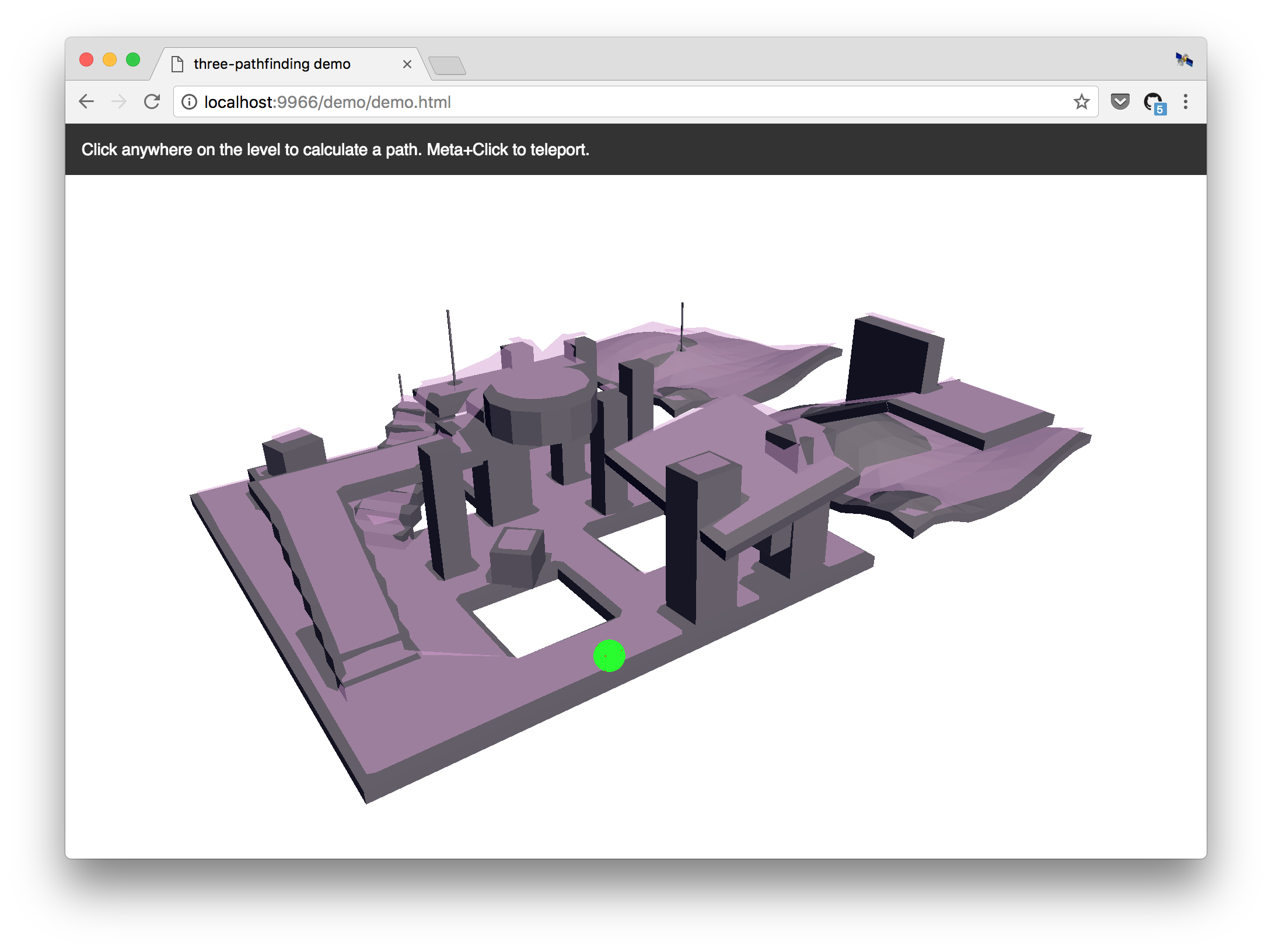
Quickstart
Installation
npm install --save three-pathfinding
Example
const Pathfinder = require('three-pathfinding');
const pathfinder = new Pathfinder();
const ZONE = 'level1';
pathfinder.setZoneData(ZONE, Pathfinder.createZone(mesh.geometry));
const groupID = pathfinder.getGroup(ZONE, a);
const path = pathfinder.findPath(a, b, ZONE, groupID);
Running the demo locally
git clone https://github.com/donmccurdy/three-pathfinding.git
cd three-pathfinding
npm install
npm run dev
The demo will start at http://localhost:9966/demo/demo.html.
API
Table of Contents
Path
Defines an instance of the pathfinding module, with one or more zones.
setZoneData
Sets data for the given zone.
Parameters
getGroup
Returns closest node group ID for given position.
Parameters
zoneID
stringposition
THREE.Vector3
Returns number
getRandomNode
Returns a random node within a given range of a given position.
Parameters
Returns Node
getClosestNode
Returns the closest node to the target position.
Parameters
position
THREE.Vector3zoneID
stringgroupID
numbercheckPolygon
boolean (optional, default false
)
Returns Node
findPath
Returns a path between given start and end points. If a complete path
cannot be found, will return the nearest endpoint available.
Parameters
startPosition
THREE.Vector3 Start position.targetPosition
THREE.Vector3 Destination.zoneID
string ID of current zone.groupID
number Current group ID.
Returns Array<THREE.Vector3> Array of points defining the path.
clampStep
Clamps a step along the navmesh, given start and desired endpoint. May be
used to constrain first-person / WASD controls.
Parameters
start
THREE.Vector3end
THREE.Vector3 Desired endpoint.node
NodezoneID
stringgroupID
numberendTarget
THREE.Vector3 Updated endpoint.
Returns Node Updated node.
createZone
(Static) Builds a zone/node set from navigation mesh geometry.
Parameters
Returns Zone
Zone
Defines a zone of interconnected groups on a navigation mesh.
Properties
Group
Defines a group within a navigation mesh.
Node
Defines a node (or polygon) within a group.
Properties
Thanks to