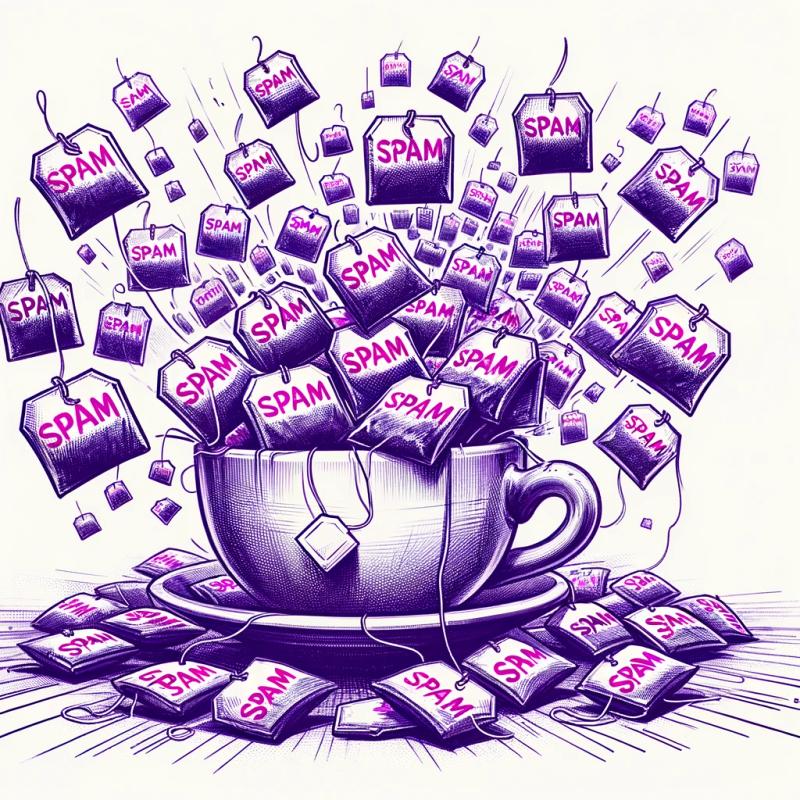
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
tiny-typed-emitter
Advanced tools
Readme
Have your events and their listeners type-checked with no overhead.
Simply add the dependency using npm:
$ npm i tiny-typed-emitter
or using yarn:
$ yarn add tiny-typed-emitter
import { TypedEmitter } from 'tiny-typed-emitter';
interface MyClassEvents {
'added': (el: string, wasNew: boolean) => void;
'deleted': (deletedCount: number) => void;
}
EventEmitter
:
class MyClass extends TypedEmitter<MyClassEvents> {
constructor() {
super();
}
}
const emitter = new TypedEmitter<MyClassEvent>();
To use with generic events interface:
interface MyClassEvents<T> {
'added': (el: T, wasNew: boolean) => void;
}
class MyClass<T> extends TypedEmitter<MyClassEvents<T>> {
}
The type of eventNames()
is a superset of the actual event names to make
subclasses of a TypedEmitter
that introduce different events type
compatible. For example the following is possible:
class Animal<E extends ListenerSignature<E>=ListenerSignature<unknown>> extends TypedEmitter<{spawn: () => void} & E> {
constructor() {
super();
}
}
class Frog<E extends ListenerSignature<E>> extends Animal<{jump: () => void} & E> {
}
class Bird<E extends ListenerSignature<E>> extends Animal<{fly: () => void} & E> {
}
const animals: Animal[] = [new Frog(), new Bird()];
Library adds no overhead. All it does is it simply reexports renamed EventEmitter
with customized typings.
You can check lib/index.js to see the exported code.
FAQs
Fully type-checked EventEmitter
The npm package tiny-typed-emitter receives a total of 220,581 weekly downloads. As such, tiny-typed-emitter popularity was classified as popular.
We found that tiny-typed-emitter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.