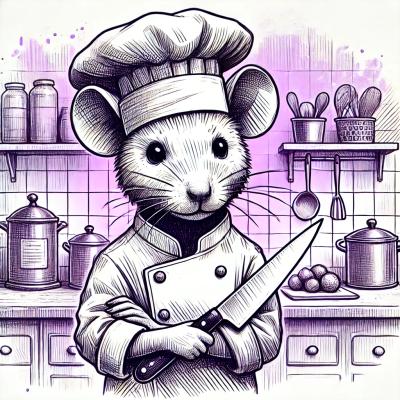
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The tippy.js npm package is a highly customizable tooltip and popover library powered by Popper.js. It is used to create and manage tooltips, popovers, and dropdowns with various effects, themes, and customization options.
Basic Tooltip
This code attaches a basic tooltip with the text 'Tooltip text' to the element with the ID 'myButton'.
tippy('#myButton', { content: 'Tooltip text' });
HTML Content
This code attaches a tooltip that uses HTML content from the element with the ID 'myTemplate' to the element with the ID 'myButton'.
tippy('#myButton', { content: document.querySelector('#myTemplate') });
Interactive Tooltip
This code creates an interactive tooltip that allows users to interact with the content inside the tooltip.
tippy('#myButton', { content: 'Interactive tooltip', interactive: true });
Placement Options
This code positions the tooltip on the right side of the element with the ID 'myButton'.
tippy('#myButton', { content: 'Tooltip on the right', placement: 'right' });
Animation Effects
This code adds a 'scale' animation effect to the tooltip shown on the element with the ID 'myButton'.
tippy('#myButton', { content: 'Animated tooltip', animation: 'scale' });
Popper.js is the core engine behind tippy.js and is responsible for the positioning logic. It can be used independently for creating tooltips, but it requires more setup compared to tippy.js.
Tooltip.js is another wrapper around Popper.js that provides a simpler API for creating tooltips. It is less feature-rich and customizable than tippy.js.
React-tooltip is a tooltip component for React applications. It offers a different API tailored to React and has its own set of features and customization options, making it a good alternative for React projects.
Hint.css is a CSS-only tooltip library that requires no JavaScript. It is simpler and has fewer features compared to tippy.js, but it is lightweight and can be a good choice for basic tooltip functionality.
Tippy.js is a highly customizable vanilla JS tooltip/popover library powered by Popper.js.
https://atomiks.github.io/tippyjs/
npm install tippy.js
CDN: https://unpkg.com/tippy.js/dist/
title
attribute containing the tooltip content.<button title="Tooltip">Text</button>
<button title="Another tooltip">Text</button>
tippy.all.min.js
script in your document, which automatically injects Tippy's CSS into head
.<script src="https://unpkg.com/tippy.js@2/dist/tippy.all.min.js"></script>
tippy()
with a CSS selector to give them a nice tooltip!<script>
tippy('button')
</script>
<!DOCTYPE html>
<html>
<head><title>Tippy Example</title></head>
<body>
<!-- Elements with `title` attributes -->
<button title="Tooltip">Text</button>
<button title="Another tooltip">Text</button>
<!-- Include Tippy -->
<script src="https://unpkg.com/tippy.js@2/dist/tippy.all.min.js"></script>
<!-- Initialize tooltips by calling the `tippy` function with a CSS selector -->
<script>
tippy('button')
</script>
</body>
</html>
View the docs for details on all of the options you can supply to customize tooltips to suit your needs.
tippy(reference [, options]) => collection object
tippy.one(reference [, options]) => Tippy instance
(v2.5+)
reference
can be:
HTMLElement
, SVGElement
)NodeList
Object
(virtual reference)tippy(reference, {
// Available v2.3+ - If true, HTML can be injected in the title attribute
allowTitleHTML: true,
// If true, the tooltip's background fill will be animated (material effect)
animateFill: true,
// The type of animation to use
animation: 'shift-away', // 'shift-toward', 'fade', 'scale', 'perspective'
// Which element to append the tooltip to
appendTo: document.body, // Element or Function that returns an element
// Whether to display the arrow. Disables the animateFill option
arrow: false,
// Transforms the arrow element to make it larger, wider, skinnier, offset, etc.
arrowTransform: '', // CSS syntax: 'scaleX(0.5)', 'scale(2)', 'translateX(5px)' etc.
// The type of arrow. 'sharp' is a triangle and 'round' is an SVG shape
arrowType: 'sharp', // 'round'
// The tooltip's Popper instance is not created until it is shown for the first
// time by default to increase performance
createPopperInstanceOnInit: false,
// Delays showing/hiding a tooltip after a trigger event was fired, in ms
delay: 0, // Number or Array [show, hide] e.g. [100, 500]
// How far the tooltip is from its reference element in pixels
distance: 10,
// The transition duration
duration: [350, 300], // Number or Array [show, hide]
// If true, whenever the title attribute on the reference changes, the tooltip
// will automatically be updated
dynamicTitle: false,
// If true, the tooltip will flip (change its placement) if there is not enough
// room in the viewport to display it
flip: true,
// The behavior of flipping. Use an array of placement strings, such as
// ['right', 'bottom'] for the tooltip to flip to the bottom from the right
// if there is not enough room
flipBehavior: 'flip', // 'clockwise', 'counterclockwise', Array
// Whether to follow the user's mouse cursor or not
followCursor: false,
// Upon clicking the reference element, the tooltip will hide.
// Disable this if you are using it on an input for a focus trigger
// Use 'persistent' to prevent the tooltip from closing on body OR reference
// click
hideOnClick: true, // false, 'persistent'
// Specifies that the tooltip should have HTML content injected into it.
// A selector string indicates that a template should be cloned, whereas
// a DOM element indicates it should be directly appended to the tooltip
html: false, // 'selector', DOM Element
// Adds an inertial slingshot effect to the animation. TIP! Use a show duration
// that is twice as long as hide, such as `duration: [600, 300]`
inertia: false,
// If true, the tooltip becomes interactive and won't close when hovered over
// or clicked
interactive: false,
// Specifies the size in pixels of the invisible border around an interactive
// tooltip that prevents it from closing. Useful to prevent the tooltip
// from closing from clumsy mouse movements
interactiveBorder: 2,
// Available v2.2+ - If false, the tooltip won't update its position (or flip)
// when scrolling
livePlacement: true,
// The maximum width of the tooltip. Add units such as px or rem
// Avoid exceeding 300px due to mobile devices, or don't specify it at all
maxWidth: '',
// If true, multiple tooltips can be on the page when triggered by clicks
multiple: false,
// Offsets the tooltip popper in 2 dimensions. Similar to the distance option,
// but applies to the parent popper element instead of the tooltip
offset: 0, // '50, 20' = 50px x-axis offset, 20px y-axis offset
// Callback invoked when the tooltip fully transitions out
onHidden(instance) {},
// Callback invoked when the tooltip begins to transition out
onHide(instance) {},
// Callback invoked when the tooltip begins to transition in
onShow(instance) {},
// Callback invoked when the tooltip has fully transitioned in
onShown(instance) {},
// If true, data-tippy-* attributes will be disabled for increased performance
performance: false,
// The placement of the tooltip in relation to its reference
placement: 'top', // 'bottom', 'left', 'right', 'top-start', 'top-end', etc.
// Popper.js options. Allows more control over tooltip positioning and behavior
popperOptions: {},
// The size of the tooltip
size: 'regular', // 'small', 'large'
// If true, the tooltip's position will be updated on each animation frame so
// the tooltip will stick to its reference element if it moves
sticky: false,
// Available v2.1+ - CSS selector string used for event delegation
target: null, // e.g. '.className'
// The theme, which is applied to the tooltip element as a class name, i.e.
// 'dark-theme'. Add multiple themes by separating each by a space, such as
// 'dark custom'
theme: 'dark',
// Changes trigger behavior on touch devices. It will change it from a tap
// to show and a tap off to hide, to a touch-and-hold to show, and a release
// to hide
touchHold: false,
// The events on the reference element which cause the tooltip to show
trigger: 'mouseenter focus', // 'click', 'manual'
// Transition duration applied to the Popper element to transition between
// position updates
updateDuration: 350,
// The z-index of the popper
zIndex: 9999
})
const instance = tippy.one(reference)
instance.show([duration])
- show the tippy, optional duration argument in msinstance.hide([duration])
- hide the tippy, optional duration argument in msinstance.enable()
- enable the tippy to allow it to show or hideinstance.disable()
- disable the tippy to prevent it from showing or hidinginstance.destroy()
- destroy the tippy, remove listeners and restore title attributeFAQs
The complete tooltip, popover, dropdown, and menu solution for the web
The npm package tippy.js receives a total of 2,064,046 weekly downloads. As such, tippy.js popularity was classified as popular.
We found that tippy.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.