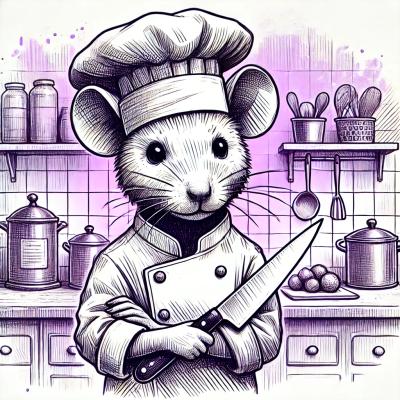
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
ts-json-schema-generator
Advanced tools
Generate JSON schema from your Typescript sources
The ts-json-schema-generator package is a tool that generates JSON schemas from TypeScript types. It is useful for ensuring that your TypeScript types and JSON schemas stay in sync, which can be particularly helpful for validating JSON data against TypeScript interfaces or types.
Generate JSON Schema from TypeScript Interface
This feature allows you to generate a JSON schema from a TypeScript interface. You need to specify the path to your TypeScript file and the type you want to generate the schema for.
const { createGenerator } = require('ts-json-schema-generator');
const config = {
path: 'path/to/your/file.ts',
tsconfig: 'path/to/your/tsconfig.json',
type: '*', // Or specify a particular type
};
const schema = createGenerator(config).createSchema(config.type);
console.log(JSON.stringify(schema, null, 2));
Generate JSON Schema for Specific Type
This feature allows you to generate a JSON schema for a specific TypeScript type. You need to specify the path to your TypeScript file and the type you want to generate the schema for.
const { createGenerator } = require('ts-json-schema-generator');
const config = {
path: 'path/to/your/file.ts',
tsconfig: 'path/to/your/tsconfig.json',
type: 'MyType', // Specify the type you want to generate the schema for
};
const schema = createGenerator(config).createSchema(config.type);
console.log(JSON.stringify(schema, null, 2));
Generate JSON Schema with Custom Settings
This feature allows you to generate a JSON schema with custom settings. You can specify various options such as exposing all types, referencing the top-level schema, and using extended JSDoc annotations.
const { createGenerator } = require('ts-json-schema-generator');
const config = {
path: 'path/to/your/file.ts',
tsconfig: 'path/to/your/tsconfig.json',
type: 'MyType',
expose: 'all', // Expose all types
topRef: true, // Reference the top-level schema
jsDoc: 'extended', // Use extended JSDoc annotations
};
const schema = createGenerator(config).createSchema(config.type);
console.log(JSON.stringify(schema, null, 2));
The typescript-json-schema package generates JSON schemas from TypeScript types. It is similar to ts-json-schema-generator but offers different configuration options and may have different performance characteristics.
The json-schema-to-typescript package converts JSON schemas to TypeScript interfaces. While it performs the reverse operation of ts-json-schema-generator, it is often used in conjunction with it to ensure consistency between JSON schemas and TypeScript types.
The ajv package is a JSON schema validator that can be used to validate JSON data against JSON schemas. While it does not generate schemas from TypeScript types, it is often used alongside ts-json-schema-generator to validate data against the generated schemas.
Extended version of https://github.com/xiag-ag/typescript-to-json-schema.
Inspired by YousefED/typescript-json-schema
. Here's the differences list:
typeChecker.getTypeAtLocation()
(so probably it keeps correct type aliases)definitions
section in the JSON schemaThis project is made possible by a community of contributors. We welcome contributions of any kind (issues, code, documentation, examples, tests,...). Please read our code of conduct.
Run the schema generator with npx:
npx ts-json-schema-generator --path 'my/project/**/*.ts' --type 'My.Type.Name'
Or install the package and then run it
npm install --save ts-json-schema-generator
./node_modules/.bin/ts-json-schema-generator --path 'my/project/**/*.ts' --type 'My.Type.Name'
Note that different platforms (e.g. Windows) may use different path separators so you may have to adjust the command above.
Also note that you need to quote paths with *
as otherwise the shell will expand the paths and therefore only pass the first path to the generator.
By default, the command-line generator will use the tsconfig.json
file in the current working directory, or the first parent directory that contains a tsconfig.json
file up to the root of the filesystem. If you want to use a different tsconfig.json
file, you can use the --tsconfig
option. In particular, if you need to use different compilation options for types, you may want to create a separate tsconfig.json
file for the schema generation only.
-p, --path <path> Source file path
-t, --type <name> Type name
-i, --id <name> $id for generated schema
-f, --tsconfig <path> Custom tsconfig.json path
-e, --expose <expose> Type exposing (choices: "all", "none", "export", default: "export")
-j, --jsDoc <extended> Read JsDoc annotations (choices: "none", "basic", "extended", default: "extended")
--markdown-description Generate `markdownDescription` in addition to `description`.
--functions <functions> How to handle functions. `fail` will throw an error. `comment` will add a comment. `hide` will treat the function like a NeverType or HiddenType.
(choices: "fail", "comment", "hide", default: "comment")
--minify Minify generated schema (default: false)
--unstable Do not sort properties
--strict-tuples Do not allow additional items on tuples
--no-top-ref Do not create a top-level $ref definition
--no-type-check Skip type checks to improve performance
--no-ref-encode Do not encode references
-o, --out <file> Set the output file (default: stdout)
--validation-keywords [value] Provide additional validation keywords to include (default: [])
--additional-properties Allow additional properties for objects with no index signature (default: false)
-V, --version output the version number
-h, --help display help for command
// main.js
const tsj = require("ts-json-schema-generator");
const fs = require("fs");
/** @type {import('ts-json-schema-generator/dist/src/Config').Config} */
const config = {
path: "path/to/source/file",
tsconfig: "path/to/tsconfig.json",
type: "*", // Or <type-name> if you want to generate schema for that one type only
};
const outputPath = "path/to/output/file";
const schema = tsj.createGenerator(config).createSchema(config.type);
const schemaString = JSON.stringify(schema, null, 2);
fs.writeFile(outputPath, schemaString, (err) => {
if (err) throw err;
});
Run the schema generator via node main.js
.
Extending the built-in formatting is possible by creating a custom formatter and adding it to the main formatter:
// my-function-formatter.ts
import { BaseType, Definition, FunctionType, SubTypeFormatter } from "ts-json-schema-generator";
import ts from "typescript";
export class MyFunctionTypeFormatter implements SubTypeFormatter {
// You can skip this line if you don't need childTypeFormatter
public constructor(private childTypeFormatter: TypeFormatter) {}
public supportsType(type: BaseType): boolean {
return type instanceof FunctionType;
}
public getDefinition(type: FunctionType): Definition {
// Return a custom schema for the function property.
return {
type: "object",
properties: {
isFunction: {
type: "boolean",
const: true,
},
},
};
}
// If this type does NOT HAVE children, generally all you need is:
public getChildren(type: FunctionType): BaseType[] {
return [];
}
// However, if children ARE supported, you'll need something similar to
// this (see src/TypeFormatter/{Array,Definition,etc}.ts for some examples):
public getChildren(type: FunctionType): BaseType[] {
return this.childTypeFormatter.getChildren(type.getType());
}
}
import { createProgram, createParser, SchemaGenerator, createFormatter } from "ts-json-schema-generator";
import { MyFunctionTypeFormatter } from "./my-function-formatter.ts";
import fs from "fs";
const config = {
path: "path/to/source/file",
tsconfig: "path/to/tsconfig.json",
type: "*", // Or <type-name> if you want to generate schema for that one type only
};
// We configure the formatter an add our custom formatter to it.
const formatter = createFormatter(config, (fmt, circularReferenceTypeFormatter) => {
// If your formatter DOES NOT support children, e.g. getChildren() { return [] }:
fmt.addTypeFormatter(new MyFunctionTypeFormatter());
// If your formatter DOES support children, you'll need this reference too:
fmt.addTypeFormatter(new MyFunctionTypeFormatter(circularReferenceTypeFormatter));
});
const program = createProgram(config);
const parser = createParser(program, config);
const generator = new SchemaGenerator(program, parser, formatter, config);
const schema = generator.createSchema(config.type);
const outputPath = "path/to/output/file";
const schemaString = JSON.stringify(schema, null, 2);
fs.writeFile(outputPath, schemaString, (err) => {
if (err) throw err;
});
Similar to custom formatting, extending the built-in parsing works practically the same way:
// my-constructor-parser.ts
import { Context, StringType, ReferenceType, BaseType, SubNodeParser } from "ts-json-schema-generator";
// use typescript exported by TJS to avoid version conflict
import ts from "ts-json-schema-generator";
export class MyConstructorParser implements SubNodeParser {
supportsNode(node: ts.Node): boolean {
return node.kind === ts.SyntaxKind.ConstructorType;
}
createType(node: ts.Node, context: Context, reference?: ReferenceType): BaseType | undefined {
return new StringType(); // Treat constructors as strings in this example
}
}
import { createProgram, createParser, SchemaGenerator, createFormatter } from "ts-json-schema-generator";
import { MyConstructorParser } from "./my-constructor-parser.ts";
import fs from "fs";
const config = {
path: "path/to/source/file",
tsconfig: "path/to/tsconfig.json",
type: "*", // Or <type-name> if you want to generate schema for that one type only
};
const program = createProgram(config);
// We configure the parser an add our custom parser to it.
const parser = createParser(program, config, (prs) => {
prs.addNodeParser(new MyConstructorParser());
});
const formatter = createFormatter(config);
const generator = new SchemaGenerator(program, parser, formatter, config);
const schema = generator.createSchema(config.type);
const outputPath = "path/to/output/file";
const schemaString = JSON.stringify(schema, null, 2);
fs.writeFile(outputPath, schemaString, (err) => {
if (err) throw err;
});
interface
typesenum
typesunion
, tuple
, type[]
typesDate
, RegExp
, URL
typesstring
, boolean
, number
types"value"
, 123
, true
, false
, null
, undefined
literalstypeof
keyof
Promise<T>
unwraps to T
@format
)npm run --silent run -- --path 'test/valid-data/type-mapped-array/*.ts' --type 'MyObject'
npm run --silent debug -- --path 'test/valid-data/type-mapped-array/*.ts' --type 'MyObject'
And connect via the debugger protocol.
AST Explorer is amazing for developers of this tool!
Publishing is handled by a 2-branch pre-release process, configured in publish-auto.yml
. All changes should be based off the default next
branch, and are published automatically.
next
pre-release tag on NPM. The result can be installed with npm install ts-json-schema-generator@next
next
, please use the squash and merge
strategy.next
into stable
using this compare link.
next
into stable
, please use the create a merge commit
strategy.FAQs
Generate JSON schema from your Typescript sources
The npm package ts-json-schema-generator receives a total of 207,223 weekly downloads. As such, ts-json-schema-generator popularity was classified as popular.
We found that ts-json-schema-generator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.