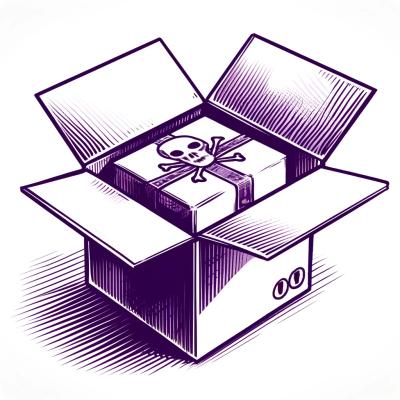
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
twitter-api-v2
Advanced tools
The twitter-api-v2 npm package is a comprehensive library for interacting with the Twitter API v2. It allows developers to perform a wide range of actions such as posting tweets, retrieving user information, managing direct messages, and more. The package is designed to be easy to use and supports both REST and streaming APIs.
Posting a Tweet
This feature allows you to post a tweet to your Twitter account. The code sample demonstrates how to initialize the TwitterApi client with an access token and post a tweet saying 'Hello, world!'.
const { TwitterApi } = require('twitter-api-v2');
const client = new TwitterApi('YOUR_ACCESS_TOKEN');
async function postTweet() {
const tweet = await client.v2.tweet('Hello, world!');
console.log(tweet);
}
postTweet();
Fetching User Information
This feature allows you to fetch information about a specific Twitter user by their username. The code sample shows how to retrieve and log the user information for the 'TwitterDev' account.
const { TwitterApi } = require('twitter-api-v2');
const client = new TwitterApi('YOUR_ACCESS_TOKEN');
async function getUserInfo() {
const user = await client.v2.userByUsername('TwitterDev');
console.log(user);
}
getUserInfo();
Streaming Tweets
This feature allows you to stream tweets in real-time based on certain criteria. The code sample demonstrates how to set up a stream to listen for tweets and log the tweet data as it comes in.
const { TwitterApi } = require('twitter-api-v2');
const client = new TwitterApi('YOUR_ACCESS_TOKEN');
async function streamTweets() {
const stream = await client.v2.searchStream({ 'tweet.fields': ['author_id'] });
for await (const { data } of stream) {
console.log(data);
}
}
streamTweets();
The 'twit' package is another popular library for interacting with the Twitter API. It supports both REST and streaming APIs, similar to twitter-api-v2. However, 'twit' is designed for the older Twitter API v1.1, whereas twitter-api-v2 is specifically for the newer API v2.
The 'twitter-lite' package is a lightweight alternative for interacting with the Twitter API. It supports both REST and streaming APIs and is designed to be minimalistic and efficient. While it can be used with both API v1.1 and v2, it may lack some of the more advanced features and ease of use provided by twitter-api-v2.
The 'node-twitter-api' package provides a simple interface for interacting with the Twitter API. It supports basic functionalities such as posting tweets and fetching user information. However, it is less comprehensive and may not support all the features available in twitter-api-v2.
WIP
Twitter api v2 (and v1 in the future) client for node
The main libraries (twit/twitter) were not updated in a while
I don't think a Twitter library need many dependencies
They caused me some frustration:
import TwitterApi, { TwitterErrors } from 'twitter-api-v2';
// bearer token auth (with V2)
const twitterClient = new TwitterApi('<YOUR_APP_USER_TOKEN>');
// token auth
const twitterClient = new TwitterApi({
appKey: '<YOUR-TWITTER-APP-TOKEN>',
appSecret: '<YOUR-TWITTER-APP-SECERT>',
accesToken: '<YOUR-TWITTER-APP-TOKEN>',
accessSecret: '<YOUR-TWITTER-APP-SECERT>',
});
// link auth
const twitterClient = new TwitterApi({
appKey: '<YOUR-TWITTER-APP-TOKEN>',
appSecret: '<YOUR-TWITTER-APP-SECERT>',
});
const authLink = await twitterClient.generateAuthLink();
// ... redirected to https://website.com?oauth_token=XXX&oauth_verifier=XXX
const { usertoken, userSecret } = twitterClient.login('<THE_OAUTH_TOKEN>', '<THE_OAUTH_VERIFIER>');
// Tell typescript it's a readonly app
const twitterClient = new TwitterApi(xxx).readOnly;
// Search for tweets
const tweets = await twitterClient.tweets.search('nodeJS', { max_results: 100 });
// Or do it your way (however, with no result typed)
const tweets = await twitterClient.v2.get('tweets/search/recent', {query: 'nodeJS', max_results: '100'});
const tweets = await twitterClient.get('https://api.twitter.com/2/tweets/search/recent?query=nodeJS&max_results=100');
// Auto-paginate
// (also checks if rate limits will be enough after the first request)
const manyTweets = await twitterClient.tweets.search('nodeJS').fetchLast(10000);
// Manage errors
try {
const manyTweets = await twitterClient.tweets.search('nodeJS').fetchLast(100000000);
} catch(e) {
if (e.errorCode === TwitterErrors.RATE_LIMIT_EXCEEDED) {
console.log('please try again later!');
} else {
throw e;
}
}
FAQs
Strongly typed, full-featured, light, versatile yet powerful Twitter API v1.1 and v2 client for Node.js.
The npm package twitter-api-v2 receives a total of 87,077 weekly downloads. As such, twitter-api-v2 popularity was classified as popular.
We found that twitter-api-v2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.