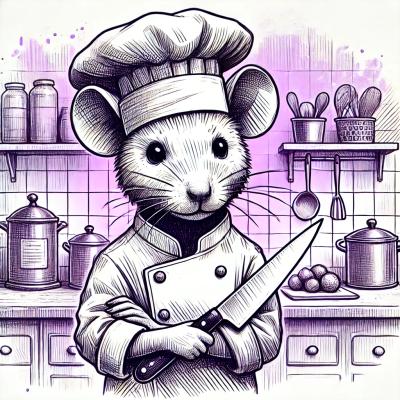
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
typeof-arguments
Advanced tools
typeof-arguments
is a module that validates arguments' types passed to the enclosing function.
of-type
package that checks whether the given value is of particular type (typeof-arguments
is based on of-type
package).npm install typeof-arguments
var args = require('typeof-arguments');
args(arguments,types[,callback])
arguments
[Object]arguments
objecttypes
[Array] of [String|RegExp] items.types[2]
specifies the expected type(s) of arguments[2]
, etc.'null'
, 'undefined'
, or any value equal to constructor.name
, eg. 'string'
, 'number'
, 'regexp'
, 'array'
, 'object'
, 'boolean'
,'buffer'
, etc.types
[String] is case insensitive: 'String'
, 'string'
, 'StRiNg'
checks if the arguments
item is of type [String]. For types
[RegExp] case insensitivity use i
flag, eg.: /String/
, /string/i
, /sTrInG/i
types
[String] can contain multiple allowed types, separated with |
, eg: 'array|object'
, 'boolean|number|null|undefined'
, string|number
. For types
[RegExp] multiple values use (x|y)
expression, eg: /(string|number)/i
types
can contain the value: 'arguments'
. It returns true
for the arguments
Objecttypes
can contain the value: 'truthy'
. It returns true
for the arguments
item's values like: "abc"
, true
, 1
, {}
, []
,function(){}
, etc.types
can contain the value: 'falsy'
. It returns true
for the arguments
item's values like: ""
, false
, 0
, null
, undefined
, etc.types
can contain the value: ''
or 'any'
, then it returns true
for the arguments
item of any type. Use it if you do not want to check the type of the particular arguments
item, eg. ['string','any','object|array']
var args = require('typeof-arguments');
hello('hello', "world!");
function hello(paramA,paramB){
args(arguments,['string','string']);
}
callback
[Function] (optional)Invalid argument [0]. The [String] argument has been passed, while the [Number] one is expected.
Invalid argument [2]. The [undefined] argument has been passed, while the argument of the type matching the regular expression: /array|object/i is expected.
Invalid argument [1]. The [Number] <<truthy>> argument has been passed, while the [falsy|String] one is expected.
callback
function.throw
statement!callback
function is executed only if at least one argument passed through the enclosing function is of invalid type.callback
function with the following properties:
index
0
, 1
actual
'[String]'
expected
'[Array]'
, '[Boolean|Number]'
, /array|object/i
message
var args = require('typeof-arguments');
hello(10, "hello!");
function hello(paramA,paramB){
args(arguments,['any','string|number'],(o)=>{
console.error(o.message);
//console.error('Not good! Use ' + o.expected + ' instead of ' + o.actual + ' for argument ' + o.index);
//throw new Error("Aborted! " + o.message);
});
}
The function args()
returns true
when all arguments passed through the enclosing function are of valid types.
The function args()
returns false
when at least one of the arguments passed through the enclosing function is of invalid type.
var args = require('typeof-arguments');
hello("hello","world!");
function hello(paramA,paramB){
var areValid = args(arguments,['string','string']);
if(!areValid) return; //stop executing code if at least one argument is of invalid type
//your code here...
}
var args = require('typeof-arguments');
function hello(paramA,paramB,paramC){
args(arguments,['number|string','any','null|array']);
}
hello("hello", "it's me!", null);
//no errors
hello(10, 20, [1,2,3]);
//no errors
hello(true,20,null);
//Invalid argument [0]. The [Boolean] argument has been passed, while the [Number|String] one is expected.
hello({name:'Paul'},false,/test/);
//Invalid argument [0]. The [Object] argument has been passed, while the [Number|String] one is expected.
//Invalid argument [2]. The [RegExp] argument has been passed, while the [null|Array] one is expected.
hello(10,20,null,30,40,50,60,70);
//no errors
hello(10);
//Invalid argument [2]. The [undefined] argument has been passed, while the [null|Array] one is expected.
var args = require('typeof-arguments');
function hello(paramA,paramB){
args(arguments,['truthy|string',/(regexp|falsy)/i]);
}
hello();
//Invalid argument [0]. The [undefined] <<falsy>> argument has been passed, while the [truthy|String] one is expected.
hello('','');
//Invalid argument [0]. The [String] <<falsy>> argument has been passed, while the [truthy|String] one is expected.
hello(1,0);
//no errors
hello(0,1);
//Invalid argument [0]. The [Number] <<falsy>> argument has been passed, while the [truthy|String] one is expected.
//Invalid argument [1]. The [Number] <<truthy>> argument has been passed, while the argument of the type matching the regular expression: /(regexp|falsy)/i is expected.
hello([1,2,3],/test/);
//no errors
hello('hello',null);
//no errors
var args = require('typeof-arguments');
function hello(paramA,paramB,paramC){
args(arguments,[/date|object|array/i,/^html.*element$/i,/^html(ul|li)element/i]);
}
var div = document.createElement('DIV');
var ul = document.createElement('UL');
var li = document.createElement('LI');
var a = document.createElement('A');
hello([1,2,3],null);
//Invalid argument [1]. The [null] argument has been passed, while the argument of the type matching the regular expression: /^html.*element$/i is expected.
hello([1,2,3],div,ol);
//no errors
hello([1,2,3],div,ul);
//no errors
hello(new Date(),a,div);
//Invalid argument [2]. The [HTMLDivElement] argument has been passed, while the argument of the type matching the regular expression: /^html[uo]listelement/i is expected.
var args = require('typeof-arguments');
function hello(paramA,paramB){
args(arguments,['arguments|falsy',/((syntax|type)error)|falsy/i]);
}
function returnArguments(){
return arguments;
}
hello(null,new TypeError());
//no errors
hello(false,new SyntaxError());
//no errors
hello(0,new Error());
//Invalid argument [1]. The [Error] argument has been passed, while the argument of the type matching the regular expression: /((syntax|type)error)|boolean/i is expected.
hello(1,false);
//Invalid argument [0]. The [Number] <<truthy>> argument has been passed, while the [arguments|falsy] one is expected.
hello(returnArguments,new TypeError());
//Invalid argument [0]. The [Function] <<truthy>> argument has been passed, while the [arguments|falsy] one is expected.
hello(returnArguments(),new TypeError());
//no errors
FAQs
Validate the type of arguments passed through the function.
The npm package typeof-arguments receives a total of 3,809 weekly downloads. As such, typeof-arguments popularity was classified as popular.
We found that typeof-arguments demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.