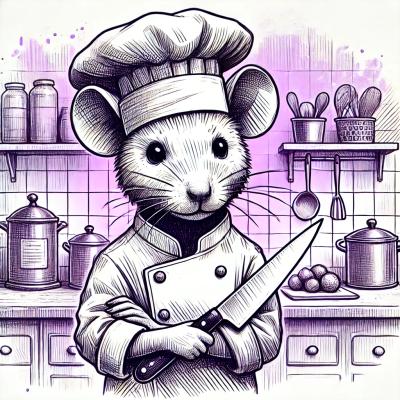
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
typeof-arguments
Advanced tools
typeof-arguments
is a module that validates arguments' types passed to the enclosing function.
of-type
package that checks whether the given value is of particular type (typeof-arguments
is based on of-type
package).typeof-properties
to validate value types of the properties of objects.npm install typeof-arguments
var argType = require('typeof-arguments');
argType(actual,expected[,callback])
actual
[Object]arguments
objectexpected
[Array]expected
item's index coheres with the index of actual
argument item passed through the enclosing function.expected
items indicate the expected types of the coherent actual
arguments passed through the enclosing function.expected
TypesThere are three ways to check the type of the arguments:
null
or undefined
values'null'
, 'undefined'
, or any value equal to constructor.name
, eg: 'string'
, 'number'
, 'regexp'
, 'array'
, 'object'
, 'boolean'
,'buffer'
, etc.'String'
, 'string'
, 'StRiNg'
checks if the argument is of type [String].|
. eg: 'array|object'
checks if the argument is of type [Array] OR
of type [Object].test('Paul',26);
function test(){
argType(arguments,['string', 'number|string|null']);
}
/null/
, /undefined/
, or any value matching the constructor.name
, eg: /String/
, /Number/
, /RegExp/
, /Array/
, /Object/
, /Boolean/
,/Buffer/
, /Promise/
, etc.i
flag, eg: /string/i
, /regexp/i
, /typeerror/i
(x|y)
expression, eg: /String|Number/
, /TypeError|Error/
/(Type|Range|Syntax)Error/
will match TypeError
, RangeError
and SyntaxError
/[A-Z].+/
will match String
, Array
, but will not match undefined
, null
, etc.test('Paul',26);
function test(){
argType(arguments,[/string/i, /num|string|null/i]);
}
null
, undefined
or any constructor object, eg: String
, TypeError
, Promise
, Array
, etc.[String,Object,Array,null]
test('Paul',26);
function test(){
argType(arguments,[String, [Number,String,null]]);
}
'arguments'
or /arguments/
. It returns true
if the argument is defined as the arguments
Object'truthy'
or /truthy/
. It returns true
if the argument has the value like: "abc"
, true
, 1
, {}
, []
,function(){}
, etc.'falsy'
or /falsy/
. It returns true
if the argument has the value like: ""
, false
, 0
, null
, undefined
, etc.''
or 'any'
or /any/
or []
, It returns true
if the argument is of any type.callback
[Function] (optional)Invalid argument [0]. The [Number] argument has been passed, while the argument of type [String] is expected.
Invalid argument [2]. The [null] argument has been passed, while the argument of type matching string expression "boolean" is expected.
Invalid argument [1]. The [null] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy|undefined" is expected.
Invalid argument [0]. The [undefined] argument has been passed, while the argument of type matching regular expression /string/i is expected.
callback
function.throw
statement!callback
function is executed only if at least one argument passed through the enclosing function is of invalid type.callback
function with the following properties:
index
0
, 1
actual
"String"
expected
"Array"
, "Boolean|Number"
, "/array|object/i"
message
var argType = require('typeof-arguments');
hello("Paul", 26);
function hello(name,age){
argType(arguments,[String,'string|number'],(o)=>{
console.error(o.message);
//console.error('Not good! Use ' + o.expected + ' instead of ' + o.actual + ' for argument ' + o.index);
//throw new Error("Aborted! " + o.message);
});
}
The function argType()
returns true
when all arguments passed through the enclosing function are of valid types.
The function argType()
returns false
when at least one of the arguments passed through the enclosing function is of invalid type.
var argType = require('typeof-arguments');
hello("hello","world!");
function hello(paramA,paramB){
var areValid = argType(arguments,['string','string']);
if(!areValid) return; //stop executing code if at least one argument is of invalid type
//your code here...
}
> git clone https://github.com/devrafalko/typeof-arguments.git
> cd typeof-arguments
> npm install
> npm test
> npm test deep //displays error messages
var argType = require('typeof-arguments');
function test(paramA,paramB,paramC){
argType(arguments,['number|string','any','null|array']);
}
test("hello", "it's me!", null);
//no errors
test(10, 20, [1,2,3]);
//no errors
test(true,20,null);
//Invalid argument [0]. The [Boolean] argument has been passed, while the argument of type matching string expression "number|string" is expected.
test({name:'Paul'},false,/test/);
//Invalid argument [0]. The [Object] argument has been passed, while the argument of type matching string expression "number|string" is expected.
//Invalid argument [2]. The [RegExp] argument has been passed, while the argument of type matching string expression "null|array" is expected.
test(10,20,null,30,40,50,60,70);
//no errors
test(10);
//Invalid argument [2]. The [undefined] argument has been passed, while the argument of type matching string expression "null|array" is expected.
var argType = require('typeof-arguments');
function test(paramA,paramB){
argType(arguments,['truthy|string',/(regexp|falsy)/i]);
}
test();
//Invalid argument [0]. The [undefined] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy" is expected.
test('','');
//Invalid argument [0]. The [String] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy" is expected.
test(1,0);
//no errors
test(0,1);
//Invalid argument [0]. The [Number] <<falsy>> argument has been passed, while the argument of type matching string expression "truthy" is expected.
//Invalid argument [1]. The [Number] <<truthy>> argument has been passed, while the argument of type matching regular expression /(regexp|falsy)/i is expected.
test([1,2,3],/test/);
//no errors
test('hello',null);
//no errors
var argType = require('typeof-arguments');
function test(paramA,paramB){
type(arguments,[String,'any','any',Number,/((syntax|type)error)|falsy/i]);
}
test();
//Invalid argument [0]. The [undefined] argument has been passed, while the argument of type [String] is expected.
test('Paul',null,false,10);
//no errors
test('Paul',null,false,10,new TypeError('error'));
//no errors
test('Paul',null,false,10,false);
//no errors
test('Paul');
//Invalid argument [3]. The [undefined] argument has been passed, while the argument of type [Number] is expected.
test('Paul',true,true,10,new Error('error'));
//Invalid argument [4]. The [Error] <<truthy>> argument has been passed, while the argument of type matching regular expression /((syntax|type)error)|falsy/i is expected.
FAQs
Validate the type of arguments passed through the function.
The npm package typeof-arguments receives a total of 3,809 weekly downloads. As such, typeof-arguments popularity was classified as popular.
We found that typeof-arguments demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.