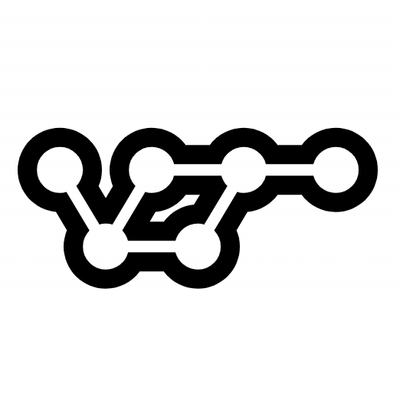
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
npm i ultipicker --save
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { UltipickerModule } from 'ultipicker'; //add this
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
UltipickerModule //add this
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
<ng2-ultipicker-dual [defaultStartDate]="yourDefaultStartDate"
[defaultEndDate]="yourDefaultEndDate">
</ng2-ultipicker-dual>
Input | Type | Default | Required | Description |
---|---|---|---|---|
minStartDate | moment.Moment | null | no | The minimal start date that user can select |
maxEndDate | moment.Moment | null | no | The maximal end date that user can select |
defaultStartDate | moment.Moment | moment() | no | The date of start date picker |
defaultEndDate | moment.Moment | moment() | no | The date of end date picker |
inputDayFormat | string | MM-DD-YYYY | no | The date format in which moment time transforms |
separator | string | ' - ' | no | The separator for readonly input between dates |
dayNames | Array | ['Su', ... 'St'] | no | Can set local day names. Order only when first day is Sunday . First day Monday temporary not supported |
monthNames | Array | ['January', ... 'December'] | no | Can set local names for months |
ranges | Array | See ranges | no | Can set predetermined ranges |
@Input() ranges: Array<Range> = [
{
key: 'Today',
start: moment(),
end: moment()
},
{
key: 'Yesterday',
start: moment().subtract(1, 'days'),
end: moment().subtract(1, 'days')
},
{
key: 'This week',
start: moment().startOf('week'),
end: moment()
},
{
key: 'This month',
start: moment().startOf('month'),
end: moment()
},
{
key: 'This year',
start: moment().startOf('year'),
end: moment()
},
{
key: 'Last week',
start: moment().subtract(1, 'week').startOf('week'),
end: moment().subtract(1, 'week').endOf('week')
},
{
key: 'Last month',
start: moment().subtract(1, 'month').startOf('month'),
end: moment().subtract(1, 'month').endOf('month')
},
{
key: 'Last year',
start: moment().subtract(1, 'year').startOf('year'),
end: moment().subtract(1, 'year').endOf('year')
}
];
Works with Reactive Forms. Just add [formControlName]
.
<ng2-ultipicker-mono [defaultDate]="yourDefaultDate">
</ng2-ultipicker-mono>
Input | Type | Default | Required | Description |
---|---|---|---|---|
minDate | moment.Moment | null | no | The minimal start date that user can select |
maxDate | moment.Moment | null | no | The maximal end date that user can select |
defaultDate | moment.Moment | moment() | no | The default date of picker |
inputDayFormat | string | MM-DD-YYYY | no | The date format in which moment time transforms |
dayNames | Array | ['Su', ... 'St'] | no | Can set local day names. Order only when first day is Sunday . First day Monday temporary not supported |
monthNames | Array | ['January', ... 'December'] | no | Can set local names for months |
autoClose | boolean | false | no | If true closes the picker when user selected the date |
defaultSets | Array | See defaultSets | no | Can set predetermined date sets |
@Input() defaultSets: Array<DefaultSet> = [
{
key: 'Today',
value: moment().startOf('day')
},
{
key: 'Yesterday',
value: moment().subtract(1, 'day').startOf('day')
},
{
key: 'Start of week',
value: moment().startOf('week')
},
{
key: 'Start of month',
value: moment().startOf('month')
}
];
Works with Reactive Forms. Just add [formControlName]
.
FAQs
A fancy datepicker for angular
The npm package ultipicker receives a total of 1 weekly downloads. As such, ultipicker popularity was classified as not popular.
We found that ultipicker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.