Vanilla JavaScript Carousel

Tiny (1Kb gzipped) JavaScript carousel with all the basic features.
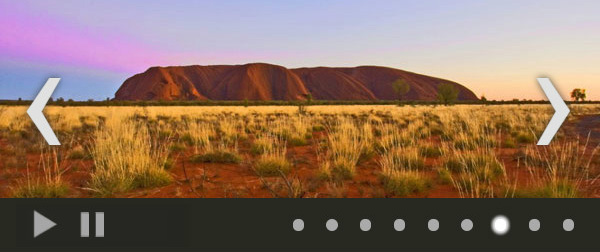
— Inspired by the blazing fast, lightweight, cross-platform and crazy popular Vanilla JS framework.
Demo
Carousel
Installation
-
Via NPM:
npm install --save vanilla-js-carousel
or in case you love shortcuts:
npm i --S vanilla-js-carousel
-
Old school:
<script src="dist/vanilla-js-carousel.min.js"></script>
Usage
-
Include the CSS and feel free to edit it or write your own:
<link rel="stylesheet" href="dist/vanilla-js-carousel.css" />
-
Write some markup:
<div class="js-Carousel" id="carousel">
<ul>
<li><img src="image-1.jpg" alt=""></li>
<li><img src="image-2.jpg" alt=""></li>
<li><img src="image-3.jpg" alt=""></li>
</ul>
</div>
-
If you installed via NPM:
const Carousel = require("vanilla-js-carousel");
-
Initialize the carousel:
var carousel = new Carousel({
elem: 'carousel',
autoplay: false,
infinite: true,
interval: 1500,
initial: 0,
dots: true,
arrows: true,
buttons: false,
btnStopText: 'Pause'
});
carousel.show(2);
carousel.next();
Options
Settings
Option | Type | Default | Description |
---|
elem | string | carousel | The id of the carousel container in the HTML markup |
interval | int | 3000 | Auto play interval in milliseconds |
initial | int | 0 | Index of the slide to start on |
autoplay | boolean | false | Enables auto play of slides |
infinite | boolean | false | Enables infinite mode |
dots | boolean | true | Display navigation dots |
arrows | boolean | true | Display navigation arrows (<prev>/<next>) |
buttons | boolean | true | Display navigation buttons (<stop>/<play>) |
Button titles
Option | Type | Default | Description |
---|
btnPlayText | string | Play | Text for <play> button |
btnStopText | string | Stop | Text for <stop> button |
arrPrevText | string | ‹ | Text for <prev> arrow |
arrNextText | string | › | Text for <next> arrow |
Methods
Method | Argument | Description |
---|
.show(index) | index: int | Moves the carousel to slide by index |
.live() | | Returns the current slide's index |
.prev() | | Triggers previous slide |
.next() | | Triggers next slide |
.play() | | Starts the autoplay |
.stop() | | Stops the autoplay |
Run the tests
npm test
Browser support and dependencies
Browser | Support | Dependencies |
---|
Chrome | yes | - |
Firefox | yes | - |
Safari | yes | - |
Opera | yes | - |
IE | yes* | Polyfill for .classList in IE9 |
* IE9 and up
License
Free. Unlicense.