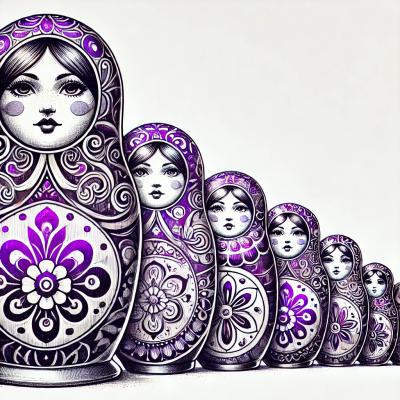
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
vue-class-component
Advanced tools
The vue-class-component package is a library that allows you to define Vue components using ES2015/ES6 class syntax. It provides a more structured and object-oriented approach to building Vue components, making it easier to manage and maintain complex applications.
Class-based Component Definition
This feature allows you to define Vue components using ES6 class syntax. The @Component decorator is used to mark a class as a Vue component.
import { Vue, Component } from 'vue-class-component';
@Component
class MyComponent extends Vue {
message: string = 'Hello, world!';
greet() {
console.log(this.message);
}
}
Lifecycle Hooks
You can define lifecycle hooks as methods within the class. This example shows how to use the created lifecycle hook.
import { Vue, Component } from 'vue-class-component';
@Component
class MyComponent extends Vue {
created() {
console.log('Component created');
}
}
Computed Properties
Computed properties can be defined as getter methods within the class. This example demonstrates a computed property that concatenates first and last names.
import { Vue, Component } from 'vue-class-component';
@Component
class MyComponent extends Vue {
firstName: string = 'John';
lastName: string = 'Doe';
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
Watchers
Watchers can be defined using the @Watch decorator. This example shows how to watch for changes to the 'message' property.
import { Vue, Component, Watch } from 'vue-class-component';
@Component
class MyComponent extends Vue {
message: string = 'Hello';
@Watch('message')
onMessageChanged(newValue: string, oldValue: string) {
console.log(`Message changed from ${oldValue} to ${newValue}`);
}
}
The vue-property-decorator package extends vue-class-component by adding decorators for props, watch, emit, and more. It provides a more comprehensive set of tools for defining Vue components using TypeScript and class-style syntax.
The vue-tsx-support package provides a way to write Vue components using TypeScript and JSX. It offers type safety and a more React-like development experience, which can be beneficial for developers familiar with React.
The vue-decorator package is another library that provides decorators for Vue components. It is similar to vue-class-component but focuses more on providing a wide range of decorators for different Vue features.
Experimental ES2016/TypeScript decorator for class-style Vue components.
Note:
data
, el
and all Vue lifecycle hooks can be directly declared as class member methods, but you cannot invoke them on the instance itself. When declaring custom methods, you should avoid these reserved names.
For all other options, declare them as static properties.
import VueComponent from 'vue-class-component'
@VueComponent
export default class Component {
// template
static template = `
<div>
<input v-model="msg">
<p>prop: {{propMessage}}</p>
<p>msg: {{msg}}</p>
<p>computed msg: {{computedMsg}}</p>
<button @click="greet">Greet</button>
</div>
`
// props
static props = {
propMessage: String
}
// return initial data
data () {
return {
msg: 123
}
}
// lifecycle hook
ready () {
this.greet()
}
// computed
get computedMsg () {
return 'computed ' + this.msg
}
// method
greet () {
alert('greeting: ' + this.msg)
}
}
$ npm install && npm run build
Theoretically, this should also work properly as a TypeScript 1.5+ decorator, but I'm not familiar enough with TypeScript to figure out how type checks would come into play. If you'd like to make it work properly with TypeScript, feel free to contribute!
FAQs
ES201X/TypeScript class decorator for Vue components
We found that vue-class-component demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.