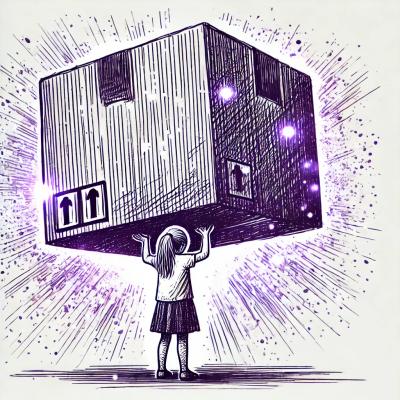
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
vue-multiselect
Advanced tools
vue-multiselect is a powerful and flexible Vue.js component for creating dropdowns with multi-select capabilities. It supports single and multiple selections, tagging, filtering, and custom rendering of options.
Single Select
This feature allows users to select a single option from a dropdown list. The `multiple` prop is set to `false` to enable single selection.
<template>
<multiselect v-model="selected" :options="options" :multiple="false"></multiselect>
</template>
<script>
import Multiselect from 'vue-multiselect';
export default {
components: { Multiselect },
data() {
return {
selected: null,
options: ['Option 1', 'Option 2', 'Option 3']
};
}
};
</script>
Multiple Select
This feature allows users to select multiple options from a dropdown list. The `multiple` prop is set to `true` to enable multiple selections.
<template>
<multiselect v-model="selected" :options="options" :multiple="true"></multiselect>
</template>
<script>
import Multiselect from 'vue-multiselect';
export default {
components: { Multiselect },
data() {
return {
selected: [],
options: ['Option 1', 'Option 2', 'Option 3']
};
}
};
</script>
Tagging
This feature allows users to add new options (tags) to the dropdown list. The `taggable` prop is set to `true`, and the `@tag` event is used to handle the addition of new tags.
<template>
<multiselect v-model="selected" :options="options" :taggable="true" @tag="addTag"></multiselect>
</template>
<script>
import Multiselect from 'vue-multiselect';
export default {
components: { Multiselect },
data() {
return {
selected: [],
options: ['Option 1', 'Option 2', 'Option 3']
};
},
methods: {
addTag(newTag) {
this.options.push(newTag);
this.selected.push(newTag);
}
}
};
</script>
Custom Option Rendering
This feature allows users to customize the rendering of options in the dropdown list. The `custom-label` prop is used to define a method that returns a custom label for each option.
<template>
<multiselect v-model="selected" :options="options" :custom-label="customLabel"></multiselect>
</template>
<script>
import Multiselect from 'vue-multiselect';
export default {
components: { Multiselect },
data() {
return {
selected: null,
options: [
{ name: 'Option 1', id: 1 },
{ name: 'Option 2', id: 2 },
{ name: 'Option 3', id: 3 }
]
};
},
methods: {
customLabel(option) {
return `${option.name} (ID: ${option.id})`;
}
}
};
</script>
vue-select is a Vue.js component that provides a similar set of features to vue-multiselect, including single and multiple selections, tagging, and custom option rendering. It is known for its simplicity and ease of use.
vue-multiselect-next is a fork of vue-multiselect that aims to provide additional features and improvements. It offers similar functionalities with enhanced performance and additional customization options.
vue-treeselect is a multi-select component with nested options support. It is particularly useful for hierarchical data structures and provides features like single and multiple selections, search, and custom rendering.
Probably the most complete selecting solution for Vue.js, without jQuery.
http://monterail.github.io/vue-multiselect/
npm install vue-multiselect
<template>
<div>
<multiselect
:selected="selected"
:options="options"
@update="updateSelected">
</multiselect>
</div>
</template>
import Multiselect from 'vue-multiselect'
export default {
components: { Multiselect },
data () {
return {
selected: null,
options: ['list', 'of', 'options']
}
},
methods: {
updateSelected (newSelected) {
this.selected = newSelected
}
}
}
You can now author custom components based on vue-multiselect mixins.
import { multiselectMixin, pointerMixin } from 'vue-multiselect'
export default {
mixins: [multiselectMixin, pointerMixin],
data () {
return {
selected: null,
options: ['list', 'of', 'options']
}
}
}
in jade-lang/pug-lang
multiselect(
:options="source",
:selected="value",
:searchable="false",
:close-on-select="false",
:allow-empty="false",
@update="updateSelected",
label="name",
placeholder="Select one",
key="name"
)
multiselect(
:options="source",
:selected="value",
:close-on-select="true",
:clear-on-select="false",
@update="updateValue",
placeholder="Select one",
label="name",
key="name"
)
multiselect(
:options="source",
:selected="multiValue",
:multiple="true",
:close-on-select="true",
@update="updateMultiValue",
placeholder="Pick some",
label="name",
key="name"
)
with @tag
event
multiselect(
:options="taggingOptions",
:selected="taggingSelected",
:multiple="true",
:taggable="true",
@tag="addTag",
@update="updateSelectedTagging",
tag-placeholder="Add this as new tag",
placeholder="Type to search or add tag",
label="name",
key="code"
)
addTag (newTag) {
const tag = {
name: newTag,
code: newTag.substring(0, 2) + Math.floor((Math.random() * 10000000))
}
this.taggingOptions.push(tag)
this.taggingSelected.push(tag)
},
Using partial API
multiselect(
:options="styleList",
:selected="selectedStyle",
:option-height="130",
:custom-label="styleLabel",
@update="updateSelectedStyle",
option-partial="customOptionPartial"
placeholder="Fav No Man’s Sky path"
label="title"
key="title"
)
import customOptionPartial from './partials/customOptionPartial.html'
Vue.partial('customOptionPartial', customOptionPartial)
// ...Inside Vue component
methods: {
styleLabel ({ title, desc }) {
return `${title} – ${desc}`
},
updateSelectedStyle (style) {
this.selectedStyle = style
}
}
<div>
<img class="option__image" :src="option.img" alt="No Man’s Sky" />
<div class="option__desc">
<span class="option__title">{{ option.title }}</span>
<span class="option__small">
{{ option.desc }}
</span>
</div>
</div>
multiselect(
:options="countries",
:selected="selectedCountries",
:multiple="multiple",
:searchable="searchable",
@search-change="asyncFind",
placeholder="Type to search",
label="name"
key="code"
)
span(slot="noResult").
Oops! No elements found. Consider changing the search query.
methods: {
asyncFind (query) {
this.countries = findService(query)
}
}
// multiselectMixin.js
props: {
/**
* Array of available options: Objects, Strings or Integers.
* If array of objects, visible label will default to option.label.
* If `labal` prop is passed, label will equal option['label']
* @type {Array}
*/
options: {
type: Array,
required: true
},
/**
* Equivalent to the `multiple` attribute on a `<select>` input.
* @default false
* @type {Boolean}
*/
multiple: {
type: Boolean,
default: false
},
/**
* Presets the selected options value.
* @type {Object||Array||String||Integer}
*/
selected: {},
/**
* Key to compare objects
* @default 'id'
* @type {String}
*/
key: {
type: String,
default: false
},
/**
* Label to look for in option Object
* @default 'label'
* @type {String}
*/
label: {
type: String,
default: false
},
/**
* Enable/disable search in options
* @default true
* @type {Boolean}
*/
searchable: {
type: Boolean,
default: true
},
/**
* Clear the search input after select()
* @default true
* @type {Boolean}
*/
clearOnSelect: {
type: Boolean,
default: true
},
/**
* Hide already selected options
* @default false
* @type {Boolean}
*/
hideSelected: {
type: Boolean,
default: false
},
/**
* Equivalent to the `placeholder` attribute on a `<select>` input.
* @default 'Select option'
* @type {String}
*/
placeholder: {
type: String,
default: 'Select option'
},
/**
* Sets maxHeight style value of the dropdown
* @default 300
* @type {Integer}
*/
maxHeight: {
type: Number,
default: 300
},
/**
* Allow to remove all selected values
* @default true
* @type {Boolean}
*/
allowEmpty: {
type: Boolean,
default: true
},
/**
* Reset this.value, this.search, this.selected after this.value changes.
* Useful if want to create a stateless dropdown, that fires the this.onChange
* callback function with different params.
* @default false
* @type {Boolean}
*/
resetAfter: {
type: Boolean,
default: false
},
/**
* Enable/disable closing after selecting an option
* @default true
* @type {Boolean}
*/
closeOnSelect: {
type: Boolean,
default: true
},
/**
* Function to interpolate the custom label
* @default false
* @type {Function}
*/
customLabel: {
type: Function,
default: false
},
/**
* Disable / Enable tagging
* @default false
* @type {Boolean}
*/
taggable: {
type: Boolean,
default: false
},
/**
* String to show when highlighting a potential tag
* @default 'Press enter to create a tag'
* @type {String}
*/
tagPlaceholder: {
type: String,
default: 'Press enter to create a tag'
},
/**
* Number of allowed selected options. No limit if false.
* @default False
* @type {Number}
*/
max: {
type: Number,
default: false
},
/**
* Will be passed with all events as second param.
* Useful for identifying events origin.
* @default null
* @type {String|Integer}
*/
id: {
default: null
}
}
// pointerMixin.js
props: {
/**
* Enable/disable highlighting of the pointed value.
* @type {Boolean}
* @default true
*/
showPointer: {
type: Boolean,
default: true
}
},
// Multiselect.vue
props: {
/**
* String to show when pointing to an option
* @default 'Press enter to select'
* @type {String}
*/
selectLabel: {
type: String,
default: 'Press enter to select'
},
/**
* String to show next to selected option
* @default 'Selected'
* @type {String}
*/
selectedLabel: {
type: String,
default: 'Selected'
},
/**
* String to show when pointing to an alredy selected option
* @default 'Press enter to remove'
* @type {String}
*/
deselectLabel: {
type: String,
default: 'Press enter to remove'
},
/**
* Decide whether to show pointer labels
* @default true
* @type {Boolean}
*/
showLabels: {
type: Boolean,
default: true
},
/**
* Limit the display of selected options. The rest will be hidden within the limitText string.
* @default 'label'
* @type {String}
*/
limit: {
type: Number,
default: 99999
},
/**
* Function that process the message shown when selected
* elements pass the defined limit.
* @default 'and * more'
* @param {Int} count Number of elements more than limit
* @type {Function}
*/
limitText: {
type: Function,
default: count => `and ${count} more`
},
/**
* Set true to trigger the loading spinner.
* @default False
* @type {Boolean}
*/
loading: {
type: Boolean,
default: false
},
/**
* Disables the multiselect if true.
* @default false
* @type {Boolean}
*/
disabled: {
type: Boolean,
default: false
}
}
# serve with hot reload at localhost:8080
npm run dev
# lib distribution build with minification
npm run bundle
# build the docs into gh-pages
npm run docs
# run unit tests
npm run test
# run unit tests watch
npm run unit-watch
For detailed explanation on how things work, checkout the guide and docs for vue-loader.
FAQs
Multiselect component for Vue
The npm package vue-multiselect receives a total of 222,025 weekly downloads. As such, vue-multiselect popularity was classified as popular.
We found that vue-multiselect demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.