What is vue3-apexcharts?
vue3-apexcharts is a Vue.js component for ApexCharts, a modern charting library that helps developers create interactive and responsive charts. This package allows seamless integration of ApexCharts into Vue 3 applications, providing a wide range of chart types and customization options.
What are vue3-apexcharts's main functionalities?
Line Chart
This feature allows you to create a line chart to visualize data trends over time. The code sample demonstrates how to set up a basic line chart with sales data.
{"template":"<apexchart type=\"line\" :options=\"chartOptions\" :series=\"series\" />","script":"import { defineComponent } from 'vue';\nimport VueApexCharts from 'vue3-apexcharts';\n\nexport default defineComponent({\n components: { VueApexCharts },\n data() {\n return {\n series: [{\n name: 'Sales',\n data: [10, 41, 35, 51, 49, 62, 69, 91, 148]\n }],\n chartOptions: {\n chart: {\n height: 350,\n type: 'line'\n },\n title: {\n text: 'Product Sales'\n },\n xaxis: {\n categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep']\n }\n }\n };\n }\n});"}
Bar Chart
This feature allows you to create a bar chart to compare different categories of data. The code sample demonstrates how to set up a basic bar chart with revenue data.
{"template":"<apexchart type=\"bar\" :options=\"chartOptions\" :series=\"series\" />","script":"import { defineComponent } from 'vue';\nimport VueApexCharts from 'vue3-apexcharts';\n\nexport default defineComponent({\n components: { VueApexCharts },\n data() {\n return {\n series: [{\n name: 'Revenue',\n data: [30, 40, 45, 50, 49, 60, 70, 91, 125]\n }],\n chartOptions: {\n chart: {\n height: 350,\n type: 'bar'\n },\n title: {\n text: 'Monthly Revenue'\n },\n xaxis: {\n categories: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep']\n }\n }\n };\n }\n});"}
Pie Chart
This feature allows you to create a pie chart to show proportions of a whole. The code sample demonstrates how to set up a basic pie chart with team performance data.
{"template":"<apexchart type=\"pie\" :options=\"chartOptions\" :series=\"series\" />","script":"import { defineComponent } from 'vue';\nimport VueApexCharts from 'vue3-apexcharts';\n\nexport default defineComponent({\n components: { VueApexCharts },\n data() {\n return {\n series: [44, 55, 13, 43, 22],\n chartOptions: {\n chart: {\n height: 350,\n type: 'pie'\n },\n labels: ['Team A', 'Team B', 'Team C', 'Team D', 'Team E'],\n title: {\n text: 'Team Performance'\n }\n }\n };\n }\n});"}
Other packages similar to vue3-apexcharts
vue-chartjs
vue-chartjs is a wrapper for Chart.js in Vue. It provides a simple way to create reactive and responsive charts using Chart.js. Compared to vue3-apexcharts, vue-chartjs offers a different set of chart types and customization options, leveraging the capabilities of Chart.js.
echarts-for-vue
echarts-for-vue is a Vue.js wrapper for Apache ECharts, a powerful charting and visualization library. It allows for the creation of complex and highly customizable charts. Compared to vue3-apexcharts, echarts-for-vue offers more advanced features and a wider range of chart types.
vue-d3-chart
vue-d3-chart is a Vue.js component for creating charts using D3.js. It provides a way to create highly customizable and interactive charts. Compared to vue3-apexcharts, vue-d3-chart offers more flexibility and control over the chart rendering process, but may require more effort to set up and customize.
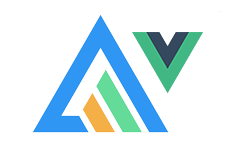
Vue 3 component for ApexCharts to build interactive visualizations in vue.
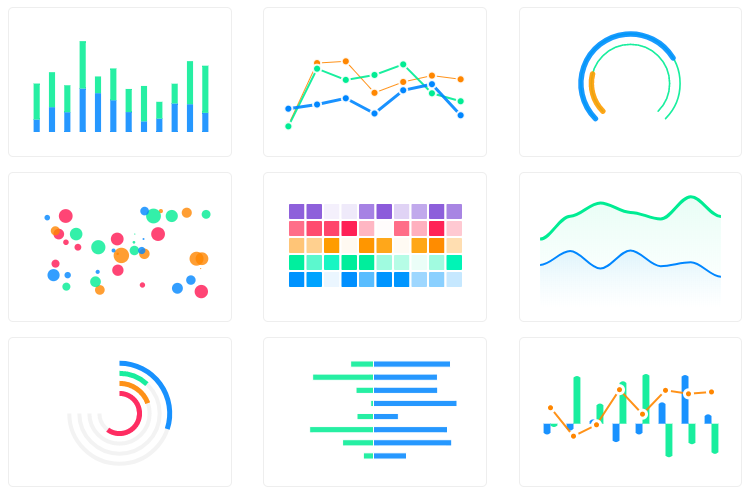
Download and Installation
Installing via npm
npm install --save apexcharts
npm install --save vue3-apexcharts
If you're looking for Vue 2.x.x compatibile component, check-out vue-apexcharts
Usage
import VueApexCharts from "vue3-apexcharts";
const app = createApp(App);
app.use(VueApexCharts);
OR
import VueApexCharts from "vue3-apexcharts";
export default {
components: {
apexchart: VueApexCharts,
},
};
To provide a $apexcharts
reference inside Vue instance
import ApexCharts from 'apexcharts';
app.config.globalProperties.$apexcharts = ApexCharts;
declare module '@vue/runtime-core' {
interface ComponentCustomProperties {
$apexcharts: typeof ApexCharts;
}
}
To create a basic bar chart with minimal configuration, write as follows:
<template>
<div>
<apexchart
width="500"
type="bar"
:options="chartOptions"
:series="series"
></apexchart>
</div>
</template>
export default {
data: function() {
return {
chartOptions: {
chart: {
id: "vuechart-example",
},
xaxis: {
categories: [1991, 1992, 1993, 1994, 1995, 1996, 1997, 1998],
},
},
series: [
{
name: "series-1",
data: [30, 40, 35, 50, 49, 60, 70, 91],
},
],
};
},
};
This will render the following chart

How do I update the chart?
Simple! Just change the series
or any option
and it will automatically re-render the chart.
Click on the below example to see this in action
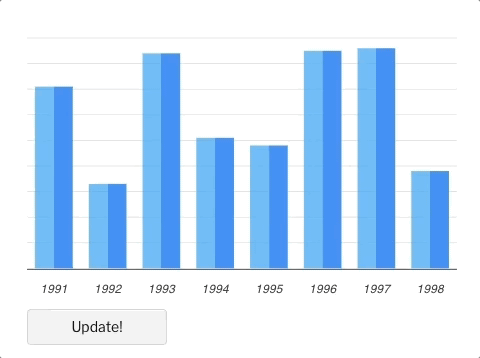
<template>
<div class="app">
<apexchart
width="550"
type="bar"
:options="chartOptions"
:series="series"
></apexchart>
<div>
<button @click="updateChart">Update!</button>
</div>
</div>
</template>
export default {
data: function() {
return {
chartOptions: {
chart: {
id: "vuechart-example",
},
xaxis: {
categories: [1991, 1992, 1993, 1994, 1995, 1996, 1997, 1998],
},
},
series: [
{
name: "series-1",
data: [30, 40, 45, 50, 49, 60, 70, 81],
},
],
};
},
methods: {
updateChart() {
const max = 90;
const min = 20;
const newData = this.series[0].data.map(() => {
return Math.floor(Math.random() * (max - min + 1)) + min;
});
const colors = ["#008FFB", "#00E396", "#FEB019", "#FF4560", "#775DD0"];
this.chartOptions = {
colors: [colors[Math.floor(Math.random() * colors.length)]],
};
this.series = [
{
data: newData,
},
];
},
},
};
Important: While updating the options, make sure to update the outermost property even when you need to update the nested property.
✅ Do this
this.chartOptions = {
...this.chartOptions,
...{
xaxis: {
labels: {
style: {
colors: ["red"],
},
},
},
},
};
❌ Not this
this.chartOptions.xaxis = {
labels: {
style: {
colors: ['red']
}
}
}}
Props
Prop | Type | Description |
---|
series* | Array | The series is an array which accepts an object in the following format. To know more about the format of dataSeries, checkout Series docs on the website. |
type* | String | line , area , bar , pie , donut , scatter , bubble , heatmap , radialBar , candlestick |
width | Number/String | Possible values for width can be 100% or 400px or 400 |
height | Number/String | Possible values for height can be 100% or 300px or 300 |
options | Object | The configuration object, see options on API (Reference) |
Methods
You don't actually need to call updateSeries() or updateOptions() manually. Changing the props will automatically update the chart. You only need to call these methods to update the chart forcefully.
Method | Description |
---|
updateSeries | Allows you to update the series array overriding the existing one |
updateOptions | Allows you to update the configuration object |
toggleSeries | Allows you to toggle the visibility of series programatically. Useful when you have custom legend. |
appendData | Allows you to append new data to the series array. |
addXaxisAnnotation | Draw x-axis annotations after chart is rendered. |
addYaxisAnnotation | Draw y-axis annotations after chart is rendered. |
addPointAnnotation | Draw point (xy) annotations after chart is rendered. |
How to call methods of ApexCharts without referencing the chart element?
Sometimes, you may want to call methods of the core ApexCharts library from some other place, and you can do so on window.ApexCharts
global variable directly. You need to target the chart by chart.id
while calling this method
Example
window.ApexCharts.exec("vuechart-example", "updateSeries", [
{
data: [40, 55, 65, 11, 23, 44, 54, 33],
},
]);
In the above method, vuechart-example
is the ID of chart, updateSeries
is the name of the method you want to call and the third parameter is the new Series you want to update.
More info on the .exec()
method can be found here
All other methods of ApexCharts can be called the same way.
Running the examples
Basic Examples are included to show how to get started using ApexCharts with Vue 3 easily.
To run the examples,
cd demo
yarn install
yarn start
Development
Install dependencies
yarn install
Bundling
yarn build
License
Vue3-ApexCharts is released under MIT license. You are free to use, modify and distribute this software, as long as the copyright header is left intact.