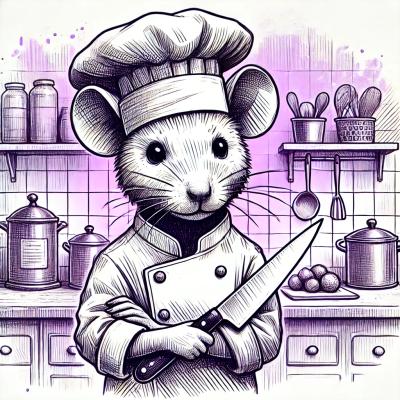
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
widget-crm-form
Advanced tools
# submodule
git submodule init
git submodule update
bash ./npm.sh i
# run
npm run serve
npm run dev
npm run build
#lint
npm run lint
npm run lint:js
npm run list:style
npm run lintfix
npm i -D path fs-extra widget-crm-form
// webpack.config.js
const path = require('path');
const fs = require('fs-extra');
const CrmCopyPlugin = require('widget-crm-form');
module.exports = {
plugins: [
new CrmCopyPlugin(fs, {
widget: path.resolve(__dirname, 'widget'),
src: path.resolve(__dirname, 'node_modules/widget-crm-form/dist'),
dest: path.resolve(__dirname, 'widget/app')
})
]
}
import timestamp from 'widget-crm-form/dist/timestamp';
/**
* @param {string} u шлях до віджета
* @param {boolean} f при відсутності шрифт Roboto - true
* @param {object} d document
* @param {object} w window
* @param {number|string} t Time Stamp
*/
!window.WidgetApp && (function(u, f, d, w, t) {
var s=d.createElement('script');
s.async=true;
s.src=u+'crm-form.js?t='+t;
// s.src=u+'crm-form-back-office.js?t='+t; для back-office
d.head.appendChild(s);
w.onload=function(){f&&w.WidgetApp.addFonts();};
}(`/widget/app/${timestamp}/`, false, document, window, (Date.now() / 180000 | 0)));
<?php
require($_SERVER["DOCUMENT_ROOT"] . "/widget/app/widget-timestamp.php");
?>
<!-- додається на сайт одноразово -->
<script>
/**
* @param {string} u шлях до віджета
* @param {boolean} f при відсутності шрифт Roboto - true
* @param {object} d document
* @param {object} w window
* @param {number|string} t Time Stamp
*/
!window.WidgetApp && (function(u, f, d, w, t) {
var s=d.createElement('script');
s.async=true;
s.src=u+'crm-form.js?t='+t;
// s.src=u+'crm-form-back-office.js?t='+t; для back-office
d.head.appendChild(s);
w.onload=function(){f&&w.WidgetApp.addFonts();};
}('/widget/app/<?php echo $widget_timestamp; ?>/',true,document,window,(Date.now()/180000|0)));
</script>
/**
* @description Виклик форми в попапі
* @param {number} id - ідентифікатор форми
* @param {any} saved - збережені дані по полях
* @example window.WidgetApp.crmFormsPopup(561);
* @example window.WidgetApp.crmFormsPopup(561, { 'answerList[44]': { disabled: true, value: 'sdfsdfsdf' } });
*/
window.WidgetApp.crmFormsPopup(id: number, saved?: any)
/**
* @description Виклик форм в попапі (селект)
* @param {{ id: number; name: string; }[]} items - масив елементів селекту, name - назва селекту для відображення
* @example window.WidgetApp.crmFormsPopupSelect([{"id": 560, "name": "Написати в відділ Сервіс"},{"id": 561, "name": "Написати нам повідомлення"},{"id": 562, "name": "Задати питання"}]);
*/
window.WidgetApp.crmFormsPopupSelect(items: { id: number; name: string; }[]);
/**
* @description Виклик форми в розмітці
* @param {HTMLElement} el HTMLElement
* @param {number} id ідентифікатор форми
* @example window.WidgetApp.crmFormsStatic(document.querySelector('.block'), 561);
* @example window.WidgetApp.crmFormsStatic(561, { 'answerList[44]': { disabled: true, value: 'sdfsdfsdf' } });
*/
window.WidgetApp.crmFormsStatic(el: HTMLElement, id: number, saved?: any);
/**
* @description попап авторизації для проекту 27ua
* @example window?.WidgetApp?.openAuthPopup();
*/
window?.WidgetApp?.openAuthPopup();
/**
* @description Компонент карт
* @param {HTMLElement} el HTMLElement
* @param {any} params параметри запиту
* @param {boolean} filters відображення фільтрів
* @param {boolean} parcerLockerSelect відображення фільтрів
* @example window.WidgetApp.addresses(document.querySelector('.block'), { lang: 'ua', info: 'contacts', true, false});
*/
window.WidgetApp.addresses(el: HTMLElement, params, filters: boolean, parcerLockerSelect?: boolean);
/**
* @description додавання шрифта Roboto
* @example window.WidgetApp.addFonts();
*/
window.WidgetApp.addFonts();
<!-- data-form-id (required) - ідентифікатор форми -->
<button data-form-id='560'>Написати в відділ Сервіс</button>
<button data-form-id='561'>Написати нам повідомлення</button>
<button data-form-id='562'>Задати питання</button>
<button data-form-id='562' data-form-saved='{ "answerList[44]": { "disabled": true, "value": "sdfsdfsdf" }}'>Задати питання</button>
<!-- data-form-items (required) - Масив з назвами і ідентифікаторами форм -->
<button data-form-items='[{"id": 560, "name": "Написати в відділ Сервіс"},{"id": 561, "name": "Написати нам повідомлення"},{"id": 562, "name": "Задати питання"}]'>Тематика звернення</button>
/**
* @description init
* @param {string} type init/destroy
* @param {number} id id чату (із запиту /api/v1/chats/assets)
* @example window?.WidgetApp?.chat('init', 4);
*/
window?.WidgetApp?.chat(type, id);
/**
* @description destroy
* @param {string} type init/destroy
* @example window?.WidgetApp?.chat('destroy');
*/
window?.WidgetApp?.chat(type);
<!-- data-wobblers (required) -->
<div data-wobblers></div>
<?php
require($_SERVER["DOCUMENT_ROOT"] . "/widget/app/widget-timestamp.php");
?>
<script>
/**
* @param {string} u шлях до віджета
* @param {object} d document
* @param {object} w window
* @param {number|string} t Time Stamp
*/
(function(u, d, w, t) {
var s=d.createElement('script');
s.async=true;
s.src=u+'nuxt-addresses.js?t='+t;
d.head.appendChild(s);
}('<?=B2B_ROOT?>/resources/widget/app/<?= $widget_timestamp; ?>/',document,window,(Date.now()/180000|0)));
</script>
<!-- data-addresses (required) -->
<div data-addresses></div>
© 2024
FAQs
widget omni channel platform & reviews
The npm package widget-crm-form receives a total of 1,181 weekly downloads. As such, widget-crm-form popularity was classified as popular.
We found that widget-crm-form demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.