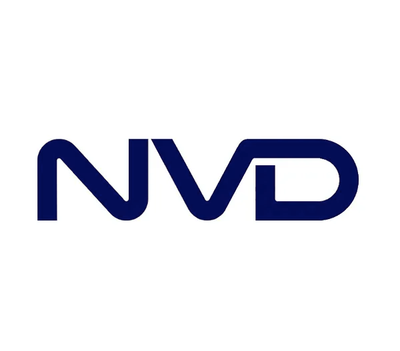
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
write-json-file
Advanced tools
The write-json-file npm package allows you to write JSON data to a file in a simple and straightforward manner. It is useful for saving configuration files, data storage, and other scenarios where JSON data needs to be persisted to the filesystem.
Write JSON to a file
This feature allows you to write a JSON object to a specified file. The example demonstrates writing a simple JSON object to a file named 'foo.json'.
const writeJsonFile = require('write-json-file');
(async () => {
await writeJsonFile('foo.json', {foo: true});
})();
Write JSON to a file with options
This feature allows you to specify options such as indentation when writing JSON to a file. The example demonstrates writing a JSON object to a file with an indentation of 2 spaces.
const writeJsonFile = require('write-json-file');
(async () => {
await writeJsonFile('foo.json', {foo: true}, {indent: 2});
})();
Write JSON to a file with replacer function
This feature allows you to use a replacer function to modify the JSON data before writing it to a file. The example demonstrates writing a JSON object to a file while excluding the 'bar' property.
const writeJsonFile = require('write-json-file');
(async () => {
await writeJsonFile('foo.json', {foo: true, bar: false}, {replacer: (key, value) => key === 'bar' ? undefined : value});
})();
The jsonfile package provides similar functionality for reading and writing JSON files. It offers a simple API for both synchronous and asynchronous operations. Compared to write-json-file, jsonfile includes both read and write capabilities in a single package.
The fs-extra package extends the native Node.js fs module with additional methods, including methods for reading and writing JSON files. It provides a more comprehensive set of file system utilities compared to write-json-file, which focuses solely on writing JSON data.
The json-write package is another alternative for writing JSON data to files. It offers a straightforward API similar to write-json-file but with fewer configuration options. It is a simpler and more lightweight option for basic JSON writing tasks.
Stringify and write JSON to a file atomically
Creates directories for you as needed.
$ npm install --save write-json-file
const writeJsonFile = require('write-json-file');
writeJsonFile('foo.json', {foo: true}).then(() => {
console.log('done');
});
Returns a Promise
.
Type: Object
Type: string
number
Default: \t
Indentation as a string or number of spaces.
Pass in null
for no formatting.
Type: boolean
function
Default: false
Sort the keys recursively.
Optionally pass in a compare
function.
Type: function
Passed into JSON.stringify
.
Type: number
Default: 0o666
Mode used when writing the file.
MIT © Sindre Sorhus
FAQs
Stringify and write JSON to a file atomically
The npm package write-json-file receives a total of 1,915,501 weekly downloads. As such, write-json-file popularity was classified as popular.
We found that write-json-file demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.