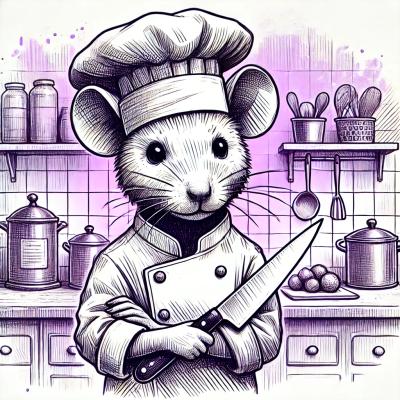
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
xendit-node
Advanced tools
This library is the abstraction of Xendit API for access from applications written with server-side Javascript.
Note: This library is only meant for usage from server-side with Xendit secret API key. For PCI compliance to be maintained, tokenization of credt cards info should be done on client side with Xendit.js.
Please check Xendit Docs
npm install --save xendit-node
For the full documentation, please refer to Xendit Node Client Docs
Configure package with your account's API keys
const Xendit = require('xendit-node');
const x = new Xendit({
publicKey: 'xnd_public...',
secretKey: 'xnd_...',
});
Instanitiate Card service using constructor that has been injected with Xendit keys
const { Card } = x;
const cardSpecificOptions = {};
const card = new Card(cardSpecificOptions);
Example: Capturing a charge
card
.captureCharge({
chargeID: 'charge-id-from-create-charge-endpoint',
externalID: 'your-system-tracking-id',
})
.then(({ id }) => {
console.log(`Charge created with ID: ${id}`);
})
.catch(e => {
console.error(`Charge creation failed with message: ${e.message}`);
});
card.createToken(data: {
cardNumber: string;
expMonth: string;
expYear: string;
cardCVN: string;
isSingleUse: boolean;
amount?: number;
shouldAuthenticate?: boolean;
})
card.createCharge(data: {
tokenID: string;
externalID: string;
amount?: number;
authID?: string;
cardCVN?: string;
capture?: boolean;
descriptor?: string;
})
card.captureCharge(data: {
chargeID: string;
amount: number;
})
card.getCharge(data: { chargeID: string })
card.createAuthetication(data: {
amount: number;
tokenID: string;
})
card.createAuthorization(data: {
tokenID: string;
externalID: string;
amount?: number;
authID?: string;
cardCVN?: string;
descriptor?: string;
})
card.reverseAuthorization(data: {
chargeID: string;
externalID: string;
})
card.createRefund(data: {
chargeID: string;
amount: number;
externalID: string;
xIdempotencyKey?: string;
})
Instanitiate VA service using constructor that has been injected with Xendit keys
const { VirtualAcc } = x;
const vaSpecificOptions = {};
const va = new VirtualAcc(vaSpecificOptions);
Example: Create a fixed virtual account
va.createFixedVA({
externalID: 'your-external-id',
bankCode: 'BCA',
name: 'Stanley Nguyen',
})
.then(({ id }) => {
console.log(`Fixed VA created with ID: ${id}`);
})
.catch(e => {
console.error(`VA creation failed with message: ${e.message}`);
});
va.getVABanks();
va.createFixedVA(data: {
externalID: string;
bankCode: string;
name: string;
virtualAccNumber?: string;
suggestedAmt?: number;
isClosed?: boolean;
expectedAmt?: number;
expirationDate?: Date;
isSingleUse?: boolean;
description?: string;
})
va.getFixedVA(data: { id: string })
va.updateFixedVA(data: {
id: string;
suggestedAmt?: number;
expectedAmt?: number;
expirationDate?: Date;
isSingleUse?: boolean;
description?: string;
})
va.getVAPayment(data: { paymentID: string })
paymentID
: ID of the payment that you obtained from your callback
Instanitiate Disbursement service using constructor that has been injected with Xendit keys
const { Disbursement } = x;
const disbursementSpecificOptions = {};
const d = new Disbursement(disbursementSpecificOptions);
Example: Create a disbursement
d.create({
externalID: 'your-external-tracking-ID',
bankCode: 'BCA',
accountHolderName: 'Stan',
accountNumber: '1234567890',
description: 'Payment for nasi padang',
amount: 10000,
})
.then(({ id }) => {
console.log(`Disbursement created with ID: ${id}`);
})
.catch(e => {
console.error(`Disbursement creation failed with message: ${e.message}`);
});
d.getBanks();
d.create(data: {
externalID: string;
bankCode: string;
accountHolderName: string;
accountNumber: string;
description: string;
amount: number;
emailTo?: string[];
emailCC?: string[];
emailBCC?: string[];
xIdempotencyKey?: string;
})
d.createBatch(data: {
reference: string;
disbursements: Array<{
externalID: string;
bankCode: string;
accountHolderName: string;
accountNumber: string;
description: string;
amount: number;
emailTo?: string[];
emailCC?: string[];
emailBCC?: string[];
}>;
xIdempotencyKey?: string;
})
d.getByID(data: { disbursementID: string })
d.getByExtID(data: { externalID: string })
Instanitiate Invoice service using constructor that has been injected with Xendit keys
const { Invoice } = x;
const invoiceSpecificOptions = {};
const i = new Invoice(invoiceSpecificOptions);
Example: Create an invoice
i.createInvoice({
externalID: 'your-external-id',
payerEmail: 'stanley@xendit.co',
description: 'Invoice for Shoes Purchase',
amount: 100000,
}).then(({ id }) => {
console.log(`Invoice created with ID: ${id}`);
});
i.createInvoice(data: {
externalID: string;
payerEmail: string;
description: string;
amount: number;
shouldSendEmail?: boolean;
callbackVirtualAccountID?: string;
invoiceDuration?: number;
successRedirectURL?: string;
failureRedirectURL?: string;
paymentMethods?: string[];
currency?: string;
midLabel?: string;
})
i.getInvoice(data: { invoiceID: string })
i.expireInvoice(data: { invoiceID: string })
i.getAllInvoices(data?: {
statuses?: string[];
limit?: number;
createdAfter?: Date;
createdBefore?: Date;
paidAfter?: Date;
paidBefore?: Date;
expiredAfter?: Date;
expiredBefore?: Date;
lastInvoiceID?: string;
clientTypes?: string[];
paymentChannels?: string[];
onDemandLink?: string;
recurringPaymentID?: string;
})
Running test suite
npm install
npm run test
Running examples
cp .env.sample .env # then fill in required environment variables
node examples/card.js # or whichever example you would like to run
There are a commit hook to run linting and formatting and push hook to run all tests. Please make sure they pass before making commits/pushes.
FAQs
OpenAPI client for xendit-node
The npm package xendit-node receives a total of 4,486 weekly downloads. As such, xendit-node popularity was classified as popular.
We found that xendit-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.