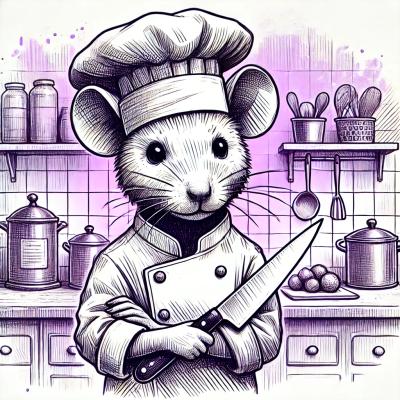
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The xorshift npm package is a JavaScript implementation of the xorshift family of pseudorandom number generators (PRNGs). It is designed to provide fast and high-quality random number generation suitable for various applications such as simulations, games, and procedural content generation.
Basic Random Number Generation
This feature allows you to generate a basic random number using the xorshift algorithm. The `random` method returns a floating-point number between 0 and 1.
const xorshift = require('xorshift');
const rng = xorshift.create();
console.log(rng.random());
Seeded Random Number Generation
This feature allows you to initialize the random number generator with a specific seed. This is useful for reproducible results in simulations or tests.
const xorshift = require('xorshift');
const seed = [1, 2, 3, 4];
const rng = xorshift.create(seed);
console.log(rng.random());
Random Integer Generation
This feature allows you to generate a random integer. The `randomint` method returns a 32-bit integer.
const xorshift = require('xorshift');
const rng = xorshift.create();
console.log(rng.randomint());
Random Number Generation in a Range
This feature allows you to generate a random number within a specified range. The `randomInRange` function demonstrates how to use the `random` method to achieve this.
const xorshift = require('xorshift');
const rng = xorshift.create();
function randomInRange(min, max) {
return min + Math.floor(rng.random() * (max - min + 1));
}
console.log(randomInRange(1, 100));
The seedrandom package provides a seeded random number generator that can be used to create reproducible random sequences. It supports multiple algorithms including Alea, XOR128, and Tychei. Compared to xorshift, seedrandom offers more flexibility in terms of algorithms and seeding options.
The random-js package is a versatile random number generator library that supports multiple PRNG algorithms including Mersenne Twister and native Math.random. It provides a rich set of features for generating random numbers, integers, and booleans, as well as shuffling arrays. Compared to xorshift, random-js offers a broader range of algorithms and utilities.
The chance package is a comprehensive library for generating random data in JavaScript. It includes functions for generating random numbers, strings, dates, and even entire objects. While xorshift focuses on efficient random number generation, chance provides a wide array of random data generation utilities.
Pseudorandom number generator using xorshift128+
npm install xorshift
var xorshift = require('xorshift');
for (var i = 0; i < 10; i++) {
console.log(xorshift.random()); // number in range [0, 1)
}
This module exports a default pseudo random generator. This generators seed have
already been set (using Date.now()
). If this is not suitable a custom
generator can be initialized using the constructor function
xorshift.constructor
. In both cases random numbers can be generated using
the two methods .random
and .randomint
.
var xorshift = require('xorshift');
This method returns a random 64-bit double, with its value in the range [0, 1).
That means 0 is inclusive and 1 is exclusive. This is equivalent to
Math.random()
.
console.log(xorshift.random()); // number between 0 and 1
This method will serve most purposes, for instance to randomly select between 2, 3 and 4, this function can be used:
function uniformint(a, b) {
return Math.floor(a + xorshift().random() * (b - a));
}
console.log(uniformint(2, 4));
This method returns a random 64-bit integer. Since JavaScript doesn't support 64-bit integers, the number is represented as an array with two elements in big-endian order.
This method is useful if high precision is required, the xorshift.random()
method won't allow you to get this precision since a 64-bit IEEE754 double
only contains the 52 most significant bits.
var bview = require('binary-view');
console.log(bview( new Uint32Array(xorshift.randomint()) ));
This method is used to construct a new random generator, with a specific seed. This is useful when testing software where random numbers are involved and getting consistent results is important.
var XorShift = require('xorshift').constructor;
var rng1 = new XorShift([1, 0, 2, 0]);
var rng2 = new XorShift([1, 0, 2, 0]);
assert(rng1.random() === rng2.random());
A XorShift
instance have both methods random
and randomint
. In fact the
xorshift
module is an instance of the XorShift
constructor.
The constructor can also be accessed as require('xorshift').XorShift
, which
is useful when using the import
syntax.
import { XorShift } from 'xorshift'
This module implements the xorshift128+ pseudo random number generator.
This is the fastest generator passing BigCrush without systematic errors, but due to the relatively short period it is acceptable only for applications with a very mild amount of parallelism; otherwise, use a xorshift1024* generator. – http://xorshift.di.unimi.it
This source also has a reference implementation for the xorshift128+ generator. A wrapper around this implementation has been created and is used for testing this module. To compile and run it:
gcc -O2 reference.c -o reference
./reference <numbers> <seed0> <seed1>
<numbers>
can be any number greater than zero, and it will be the number
of random numbers written to stdout
. The default value is 10
.<seed0>
and <seed1>
forms the 128bit seed that the algorithm uses. Default
is [1, 2]
.This software is licensed under "MIT"
Copyright (c) 2014 Andreas Madsen & Emil Bay
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Random number generator using xorshift128+
We found that xorshift demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.