📣 Our Discord Server is Live
JOIN US to chat about questions, bugs, plans, or anything off the top of your head.
What is ZenStack?
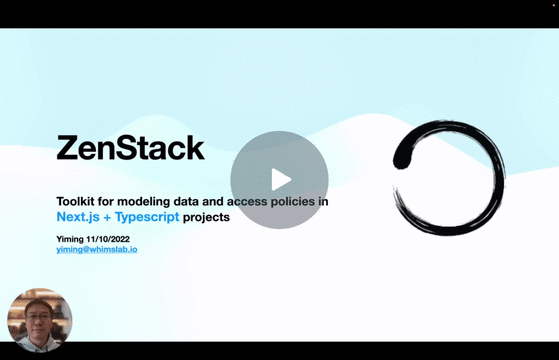
ZenStack is a toolkit for modeling data and access policies in full-stack development with Next.js and Typescript.
Next.js is an excellent full-stack framework. However, building the backend part of a web app is still quite challenging. For example, implementing CRUD services efficiently and securely is tricky and not fun.
ZenStack simplifies it by providing:
- An intuitive data modeling language for defining data types, relations, and access policies
model User {
id String @id @default(cuid())
email String @unique
// one-to-many relation to Post
posts Post[]
}
model Post {
id String @id @default(cuid())
title String
content String
published Boolean @default(false)
// one-to-many relation from User
author User? @relation(fields: [authorId], references: [id])
authorId String?
// must signin to CRUD any post
@@deny('all', auth() == null)
// allow CRUD by author
@@allow('all', author == auth())
}
- Auto-generated CRUD services and strongly typed React hooks
const { find } = usePost();
const posts = get({ where: { public: true } });
return (
<>
{posts?.map((post) => (
<Post key={post.id} post={post} />
))}
</>
);
Since CRUD APIs are automatically generated with access policies injected, you can safely implement most of your business logic in your front-end code. Read operations never return data that's not supposed to be visible to the current user, and writes will be rejected if unauthorized.
ZenStack is heavily inspired and built above Prisma ORM, which is, in our opinion, the best ORM toolkit in the market. Familiarity with Prisma should make it easy to pick up ZenStack, but it's not a prerequisite since the modeling language is intuitive and the development workflow is straightforward.
Getting started
A step by step guide for getting started
A complete sample with a collaborative todo app
How does it work?
ZenStack has four essential responsibilities:
- Modeling data and mapping the model to DB schema and programmable client library
- Integrating with authentication
- Generating CRUD APIs and enforcing data access policies
- Providing type-safe React hooks
Let's briefly go through each of them in this section.
Data modeling
ZenStack uses a schema language called ZModel
to define data types and their relations. The zenstack
CLI takes a schema file as input and generates database client code. Such client code allows you to program against database in server-side code in a fully typed way without writing any SQL. It also provides commands for synchronizing data models with DB schema, and generating "migration records" when your data model evolves.
Internally, ZenStack entirely relies on Prisma for ORM tasks. The ZModel language is a superset of Prisma's schema language. When zenstack generate
is run, a Prisma schema named 'schema.prisma' is generated beside your ZModel file. You don't need to commit schema.prisma to source control. The recommended practice is to run zenstack generate
during deployment, so Prisma schema is regenerated on the fly.
Authentication
ZenStack is not an authentication library, but it gets involved in two ways.
Firstly, if you use any authentication method that involves persisting users' identity, you'll model the user's shape in ZModel. Some auth libraries, like NextAuth, require user entity to include specific fields, and your model should fulfill such requirements. In addition, credential-based authentication requires validating user-provided credentials, and you should implement this using the database client generated by ZenStack.
To simplify the task, ZenStack automatically generates an adapter for NextAuth when it detects that the next-auth
npm package is installed. Please refer to the starter code for how to use it. We'll keep adding integrations/samples for other auth libraries in the future.
Secondly, authentication is almost always connected to authorization. ZModel allows you to reference the current login user via auth()
function in access policy expressions. Like,
model Post {
author User @relation(fields: [authorId], references: [id])
...
@@deny('all', auth() == null)
@@allow('all', auth() == author)
The value returned by auth()
is provided by your auth solution via the getServerUser
hook function you provide when mounting ZenStack APIs. Check this code for an example.
Data access policy
The primary value that ZenStack adds over a traditional ORM is the built-in data access policy engine. This allows most business logic to be safely implemented in front-end code. Since ZenStack delegates database access to Prisma, it enforces access policies by analyzing queries sent to Prisma and injecting guarding conditions. For example, suppose we have a policy saying "a post can only be accessed by its author if it's not published", expressed in ZModel as:
@@deny('all', auth() != author && !published)
When client code sends a query to list all Post
s, ZenStack's generated code intercepts it and injects the where
clause before passing it through to Prisma (conceptually):
{
where: {
AND: [
{ ...userProvidedFilter },
{
NOT: {
AND: [
{ author: { not: { id: user.id } } },
{ published: { not: true } },
],
},
},
];
}
}
Similar procedures are applied to write operations and more complex queries involving nested reads and writes. To ensure good performance, ZenStack generates conditions statically, so it doesn't need to introspect ZModel at runtime. The engine also makes the best effort to push down policy constraints to the database to avoid fetching data unnecessarily and discarding afterward.
Please BEWARE that policy checking is only applied when data access is done using the generated client-side hooks or, equivalently, the RESTful API. If you use service.db
to access the database directly from server-side code, policies are bypassed, and you have to do all necessary checking by yourself. We've planned to add helper functions for "injecting" the policy checking on the server side in the future.
Type-safe React hooks
Strongly-typed React hooks are generated for CRUD operations, saving the need to write boilerplate code.
Thanks to Prisma's power, ZenStack generates accurate Typescript types for your data models:
- The model itself
- Argument types for listing models, including filtering, sorting, pagination, and nested reads for related models
- Argument types for creating and updating models, including nested writes for related models
The cool thing is that the generated types are shared between client-side and server-side code, so no matter which side of code you're writing, you can always enjoy the pleasant IDE intellisense and typescript compiler's error checking.
Programming with the generated code
Client-side
The generated CRUD services should be mounted at /api/zenstack
route. The following React hooks are generated for each data model:
- find: listing entities with filtering, ordering, pagination, and nested relations
const { find } = usePost();
const posts = find({
where: { published: false },
include: { author: true },
orderBy: { updatedAt: 'desc' },
});
- get: fetching a single entity by id, with nested relations
const { get } = usePost();
const post = get(id, {
include: { author: true },
});
- create: creating a new entity, with the support for nested creation of related models
const { create } = usePost();
const post = await create({
data: {
title: 'My New Post',
author: {
connect: { id: session.user.id },
},
comments: {
create: [{ content: 'First comment' }],
},
},
});
- update: updating an entity, with the support for nested creation/update of related models
const { update } = usePost();
const post = await update(id, {
data: {
const: 'My post content',
comments: {
create: [{ content: 'A new comment' }],
},
},
});
const { del } = usePost();
const post = await del(id);
Internally ZenStack generated code uses SWR to do data fetching so that you can enjoy its caching, polling, and automatic revalidation features.
Server-side
If you need to do server-side coding, either through implementing an API endpoint or by using getServerSideProps
for SSR, you can directly access the database client generated by Prisma:
import service from '@zenstackhq/runtime';
export const getServerSideProps: GetServerSideProps = async () => {
const posts = await service.db.post.findMany({
where: { published: true },
include: { author: true },
});
return {
props: { posts },
};
};
Please note that server-side database access is not protected by access policies. This is by-design so as to provide a way of bypassing the policies. Please make sure you implement authorization properly.
Learning more
Reach out to us for issues, feedback and ideas!
Discord | Twitter |
Discussions | Issues